I am using this to retrieve the database connection atm.
$db = Zend_Db_Table::getDefaultAdapter();
I do set this up in my config like this:
resources.db.adapter = pdo_mysql
resources.db.isDefaultTableAdapter = true
resources.db.params.host = localhost
resources.db.params.username = root
resources.db.params.password = password
resources.db.params.dbname = db
resources.db.params.profiler.enabled = true
resources.db.params.profiler.class = Zend_Db_Profiler
I would like to output everything to a sql.log for example. Is this possible to apply on the default adapter? for example through the settings, so I can ignore it in production environment?
Much appriciated.
I did look at: How to enable SQL output to log file with Zend_Db? but it didn't seem to cover my issue.
/Marcus
There is an example of extending Zend_Db_Profiler so you can write the queries to /logs/db-queries.log file.
So you have to do the following:
- Create My_Db_Profiler_Log class in the library folder
- Add the following lines to the application.ini
resources.db.params.profiler.enabled = true
resources.db.params.profiler.class = My_Db_Profiler_Log
Note: be aware, that the log file will become very big, very soon! So it is a good idea to log only the queries you are interested in. And this example should be considered only as a starting point in implementation of such a logging system.
Here is the code for the custom profiler class:
<?php
class My_Db_Profiler_Log extends Zend_Db_Profiler {
/**
* Zend_Log instance
* @var Zend_Log
*/
protected $_log;
/**
* counter of the total elapsed time
* @var double
*/
protected $_totalElapsedTime;
public function __construct($enabled = false) {
parent::__construct($enabled);
$this->_log = new Zend_Log();
$writer = new Zend_Log_Writer_Stream(APPLICATION_PATH . '/logs/db-queries.log');
$this->_log->addWriter($writer);
}
/**
* Intercept the query end and log the profiling data.
*
* @param integer $queryId
* @throws Zend_Db_Profiler_Exception
* @return void
*/
public function queryEnd($queryId) {
$state = parent::queryEnd($queryId);
if (!$this->getEnabled() || $state == self::IGNORED) {
return;
}
// get profile of the current query
$profile = $this->getQueryProfile($queryId);
// update totalElapsedTime counter
$this->_totalElapsedTime += $profile->getElapsedSecs();
// create the message to be logged
$message = "\r\nElapsed Secs: " . round($profile->getElapsedSecs(), 5) . "\r\n";
$message .= "Query: " . $profile->getQuery() . "\r\n";
// log the message as INFO message
$this->_log->info($message);
}
}
?>
Extend the Zend_Db_Profiler to write to an SQL.log and attach the profiler to your db adapter
<?php
class File_Profiler extends Zend_Db_Profiler {
/**
* The filename to save the queries
*
* @var string
*/
protected $_filename;
/**
* The file handle
*
* @var resource
*/
protected $_handle = null;
/**
* Class constructor
*
* @param string $filename
*/
public function __construct( $filename ) {
$this->_filename = $filename;
}
/**
* Change the profiler status. If the profiler is not enabled no
* query will be written to the destination file
*
* @param boolean $enabled
*/
public function setEnabled( $enabled ) {
parent::setEnabled($enabled);
if( $this->getEnabled() ) {
if( !$this->_handle ) {
if( !($this->_handle = @fopen($this->_filename, "a")) ) {
throw new Exception("Unable to open filename {$this->_filename} for query profiling");
}
}
}
else {
if( $this->_handle ) {
@fclose($this->_handle);
}
}
}
/**
* Intercept parent::queryEnd to catch the query and write it to a file
*
* @param int $queryId
*/
public function queryEnd($queryId) {
$state = parent::queryEnd($queryId);
if(!$this->getEnabled() || $state == self::IGNORED) {
return;
}
$profile = $this->getQueryProfile($queryId);
@fwrite($this->_handle, round($profile->getElapsedSecs(),5) . " " . $profile->getQuery() . " " . ($params=$profile->getQueryParams())?$params:null);
}
}
Haven't test it, but it should do the trick. Give it a try and let me know.
Btw you do know that you can log all queries on the mysql as well?
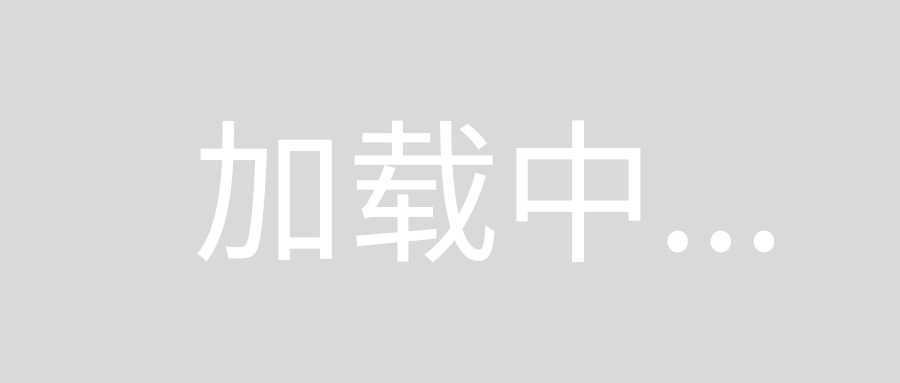
this will let you see sql queries to the web page , IT MIGHT BE OFF TOPIC but it helpful
I am highly recommend you to use ZF debug bar , it will give you very handy information
i am using it to see my doctrine queries , and it had support for zend db
too
https://github.com/jokkedk/ZFDebug