canvas.DrawTextOnPath does not seem to work on a Lollipop device. See the difference here. (The Nexus 10
image is correct but Lollipop does not display correctly)
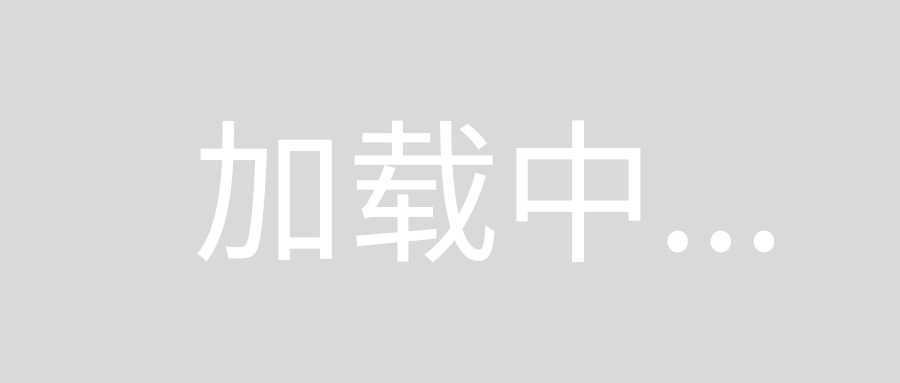
The code is a simple path draw.
// Path for the inner circle
unitPath = new Path();
unitPath.addArc(unitRect, 180.0f, 180.0f);
// Draw the text and the path
canvas.drawTextOnPath("Inner Circle", unitPath, 0.0f, 0.0f, unitPaint);
canvas.drawPath(unitPath,unitPaint);
The Android Studio test project illustrating this issue can be seen here for anyone who wants to see it.
https://dl.dropboxusercontent.com/u/6768304/WebLinks/TestApp.rar
Is there something "different" I need to do on this device?
OK so it seems that DrawTextOnPath is a little broken now with font sizes below 1.0f
The solution is to scale everything up, draw the text then shrink it back down.
The drawTitle method in the demo project would change from this:
private void drawTitle(Canvas canvas) {
canvas.drawTextOnPath(upperTitle, upperTitlePath, 0.0f, 0.02f, unitPaint);
canvas.drawTextOnPath(lowerTitle, lowerTitlePath, 0.0f, 0.0f, unitPaint);
canvas.drawTextOnPath(unitTitle, unitPath, 0.0f, 0.0f, unitPaint);
canvas.drawPath(unitPath,unitPaint);
}
to this:
private void drawTitle(Canvas canvas) {
//Save original font size
float originalTextSize = unitPaint.getTextSize();
// set a magnification factor
final float magnifier = 100f;
// Scale the canvas
canvas.save();
canvas.scale(1f / magnifier, 1f / magnifier);
// create new rects and paths based on the new scale
unitRect = new RectF();
unitRect.set((faceRect.left + unitPosition) * magnifier, (faceRect.top + unitPosition) * magnifier, (faceRect.right - unitPosition) * magnifier, (faceRect.bottom - unitPosition) * magnifier);
unitPath = new Path();
unitPath.addArc(unitRect, 180.0f, 180.0f);
titleRect = new RectF();
titleRect.set((faceRect.left + titlePosition) * magnifier, (faceRect.top + titlePosition) * magnifier, (faceRect.right - titlePosition) * magnifier, (faceRect.bottom - titlePosition) * magnifier);
upperTitlePath = new Path();
upperTitlePath.addArc(titleRect, 180.0f, 180.0f);
titleRect = new RectF();
titleRect.set((faceRect.left + titlePosition) * magnifier, (faceRect.top + titlePosition) * magnifier, (faceRect.right - titlePosition) * magnifier, (faceRect.bottom - titlePosition) * magnifier);
lowerTitlePath = new Path();
lowerTitlePath.addArc(titleRect, -180.0f, -180.0f);
// increase the font size
unitPaint.setTextSize(originalTextSize * magnifier);
// do the drawing of the text
canvas.drawTextOnPath(unitTitle, unitPath, 0.0f, 0.0f, unitPaint);
canvas.drawTextOnPath(upperTitle, upperTitlePath, 0.0f, 0.02f, unitPaint);
canvas.drawTextOnPath(lowerTitle, lowerTitlePath, 0.0f, 0.0f, unitPaint);
// bring everything back to normal
canvas.restore();
unitPaint.setTextSize(originalTextSize);
canvas.drawPath(unitPath, unitPaint);
}
Yes, broken as of Lollipop. Worked perfectly in 4.4.4.
https://code.google.com/p/android/issues/detail?id=40965
I'm setting text size to 5.f if it was smaller, scaling the canvas down, and scaling the baseline path up appropriately. Slow but it works, can't wait till I can remove this awful cludge.