I'm trying to make an Android layout: 3 components inside a vertical LinearLayout. The center component is a ScrollView
that contains a TextView
. When the TextView
contains a significant amount of text (more than can fit on the screen), the ScrollView
grows all the way to the bottom of the screen, shows scrollbars, and pushes the last component, a LinearLayout
with a Button
inside, off the screen.
If the text inside the TextView
inside the ScrollView
is short enough, the button at the bottom of the screen is positioned perfectly.
The layout I'm trying to achieve is:
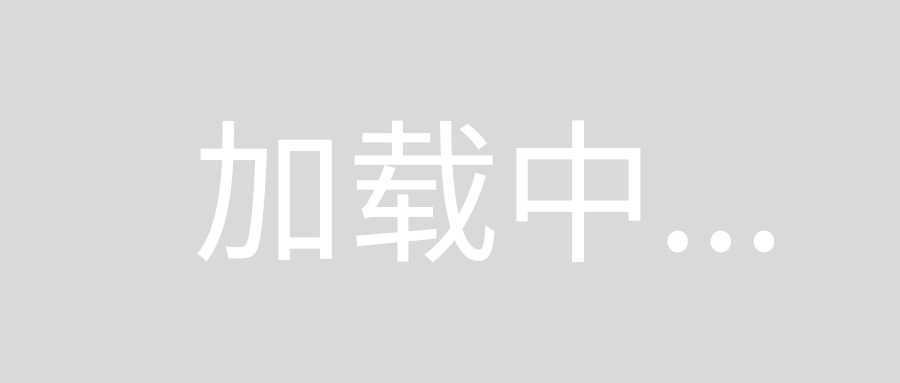
The XML for the layout I've written is:
<?xml version="1.0" encoding="UTF-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="#FFFFFF"
android:layout_marginLeft="10dip"
android:layout_marginRight="10dip"
android:layout_marginTop="10dip"
android:layout_marginBottom="10dip"
android:text="Title />
<ScrollView
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:autoLink="web"
android:textColor="#FFFFFF"
android:background="#444444"
android:padding="10dip" />
</ScrollView>
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:layout_weight="1">
<LinearLayout
android:orientation="horizontal"
android:layout_width="0dip"
android:layout_height="wrap_content"
android:layout_weight="1"/>
<Button android:id="@+id/login_button"
android:layout_width="0dip"
android:layout_height="wrap_content"
android:layout_gravity="bottom"
android:layout_weight="1"
android:text="@string/next_button"/>
</LinearLayout>
</LinearLayout>
The scrollview is the second view object and is set to wrap_content, which is more than the screen.
I recommend a RelativeLayout. Top textview first with android:alignParentTop="true"
, the bottom LinearLayout next with android:alignParentBottom="true"
and the scrollview listed last in the xml with the value android:alignBelow="@id/whatYouCallTheHeader
.
This will align the bottom bar at the bottom of the screen, and the header at the top, no matter the size. Then the scrollview will have its own place, after the header and footer have been placed.
you should go for relativeLayout rather than LinearLayout. And you can use some properties like alignBelow and all.
Try adding a layout weight into the ScrollView
ie.
<ScrollView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_weight="1">
This worked for me in a situation almost identical to the one you're presenting but left me wondering why, because it is counter-intuitive that increasing the layout weight of a control from 0 (the default if you don't specify a layout_weight
) to 1 should make a control which is already using too much space smaller.
I suspect the reason it works is that by not specifying a layout_weight you actually allow the layout to ignore the size of the scroll view relative to other controls and conversely if do specify one you give it permission to shrink it in proportion to the weights you assign.
![Fixed Header-Footer and scrollable Body layout ][1]
This is what you are looking for . Most of the app in android had this type of layout ,
a fixed header and footer and a scrollable body . The xml for this layout is
<?xml version="1.0" encoding="UTF-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:background="#5599DD"
android:layout_height="fill_parent">
<!-- Header goes here -->
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="#FFFFFF"
android:layout_marginLeft="10dip"
android:layout_marginRight="10dip"
android:layout_marginTop="10dip"
android:layout_marginBottom="10dip"
android:textSize="20sp"
android:layout_gravity="center"
android:text="Title" />
<!-- Body goes here -->
<ScrollView
android:layout_weight="1"
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:autoLink="web"
android:text="@string/lorem_ipsum"
android:textColor="#FFFFFF"
android:padding="10dip" />
</ScrollView>
<!-- footer goes here -->
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content">
<RelativeLayout
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<Button
android:id="@+id/login_button"
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:layout_gravity="bottom"
android:layout_alignParentRight="true"
android:text="Button"/>
</RelativeLayout>
</LinearLayout>
</LinearLayout>