I'm using this for creating twitter follow buttons on my twitter web-app. Implementation is as easy as this:
<span id="follow-twitterapi"></span>
<script type="text/javascript">
twttr.anywhere(function (T) {
T('#follow-twitterapi').followButton("twitterapi");
});
</script>
when you put that code into your html document you get this button in that span element in an iframe:
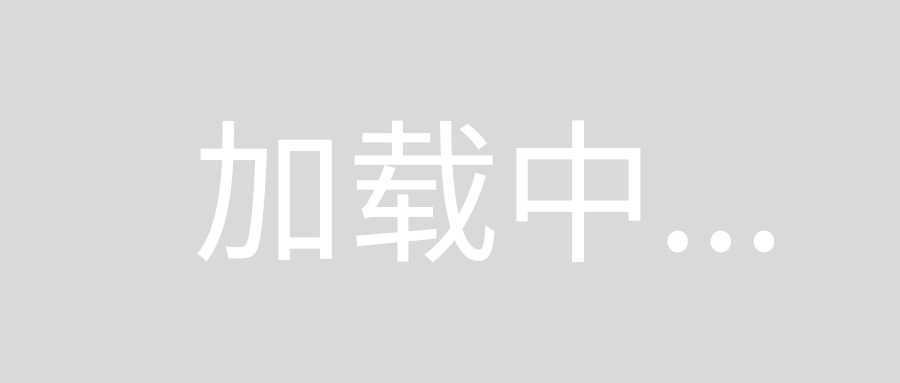
after you click follow, you get this (or a similar thing saying you're following @someone):
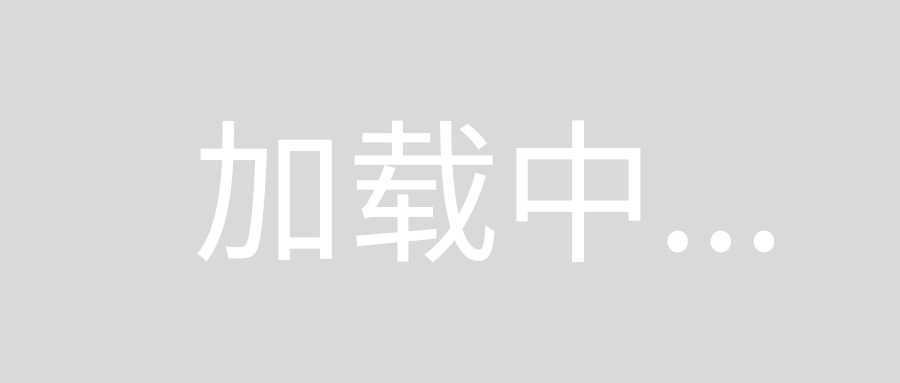
however i can't get any information back to my app after this button is used, (because this is rendered in an iframe and because of same-origin policy of javascript, i can't access to contents of that iframe), is there any methods provided with this widget, to inform my app when a user starts following someone using this?
Thanks.
You can. Here is my code:
<a href="https://twitter.com/gafitescu" class="twitter-follow-button">Follow @gafitescu</a>
<script src="//platform.twitter.com/widgets.js" type="text/javascript"></script>
<script type="text/javascript">
twttr.events.bind('follow', function(event) {
console.log(event);
var followed_user_id = event.data.user_id;
var followed_screen_name = event.data.screen_name;
});
</script>
You might try using JSONP to overcome the cross-domain restriction.
Instead of alerting, as below (which just shows how to get the data that might be relevant--a list of follower ids or a total number of followers), you could set a variable to check for the last count and see if it has incremented (of course, that could be due to it incrementing by someone not using your app, but not sure if you needed that).
$.getJSON('http://api.twitter.com/1/followers/ids.json?screen_name=brettz9&callback=?',
function (obj) {
// Shows the follower ids
alert(obj);
}
);
or just check the follower count:
$.getJSON('http://api.twitter.com/1/users/show.json?screen_name=brettz9&callback=?',
function (obj) {
alert(obj.followers_count);
}
);
Instead of using your original script then, you'd just include the follow image directly inside a link and set an onclick event which, if clicked, would swap the image and start polling with setInterval
to check to see if the follower count changed. Or if you wanted to use the widget to take advantage of its user awareness, you could just poll anyways.
You can use below code. You will get
FOLLOW!
alert after clicking on follow button
<html>
<a href=http://twitter.com/directdialogs class="twitter-follow-button"
data-show-count="true">Follow@directdialogs</a>
<script type="text/javascript" charset="utf-8">
window.twttr = (function (d,s,id) {
var t, js, fjs = d.getElementsByTagName(s)[0];
if (d.getElementById(id)) return; js=d.createElement(s); js.id=id;
js.src="//platform.twitter.com/widgets.js"; fjs.parentNode.insertBefore(js, fjs);
return window.twttr || (t = { _e: [], ready: function(f){ t._e.push(f) } });
}(document, "script", "twitter-wjs"));
</script>
<script>
twttr.ready(function (twttr) {
twttr.events.bind('follow', function(e) {
alert("FOLLOW!")
});
});
</script>
</html>