I'm using Pandas
to draw a scatterplot matrix: from pandas.tools.plotting import scatter_matrix
. The problem is that the names of the columns in the DataFrame
are too long and I need them to be vertical in the x-axis and horizontal in the y-axis so they can fit. I'm not able to figure out at all how to do that in Pandas. I know how to do it in matplotlib
but not in Pandas.
My code:
pylab.clf()
df = pd.DataFrame(X, columns=the_labels)
axs = scatter_matrix(df, alpha=0.2, diagonal='kde')
Edit:
I need to use pylab.clf()
because I'm plotting a lot of figures, so calling pylab.figure()
each time is too memory consuming.
Major help from this answer: https://stackoverflow.com/a/18994338/2632856
a = [[1,2], [2,3], [3,4], [4, 5], [1, 6], [2,7], [1,8]]
df = pd.DataFrame(a,columns=['askdabndksbdkl','aooweoiowiaaiwi'])
axs = pd.scatter_matrix( df, alpha=0.2, diagonal='kde')
n = len(df.columns)
for x in range(n):
for y in range(n):
# to get the axis of subplots
ax = axs[x, y]
# to make x axis name vertical
ax.xaxis.label.set_rotation(90)
# to make y axis name horizontal
ax.yaxis.label.set_rotation(0)
# to make sure y axis names are outside the plot area
ax.yaxis.labelpad = 50
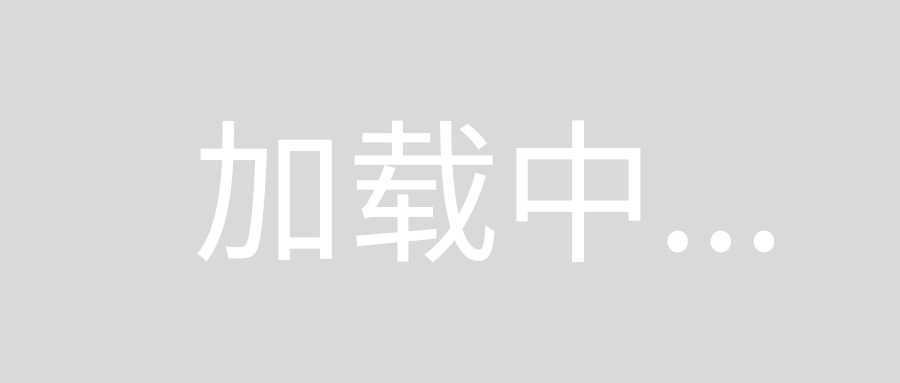
scatter_matrix
returns a two-dimensional array of matplotlib
subplots. This means you should be able to iterate over the two arrays and use matplotlib
functions to rotate axes. Based on the source used to implement scatter_matrix
and the private helper function _label_axis
, it looks as though you should be able to perform your rotations for all the plots with:
from matplotlib.artist import setp
x_rotation = 90
y_rotation = 90
for row in axs:
for subplot in row:
setp(subplot.get_xticklabels(), rotation=x_rotation)
setp(subplot.get_yticklabels(), rotation=y_rotation)
I don't have a good way to test this so it may require a bit of playing around.