how to create custom contact list with checkbox to select multiple contacts from list in android
public class AddFromContacts extends Activity {
ArrayList<String> listname;
// ArrayList<String> list_no;
Context context;
LayoutInflater inflater;
ListView contactlistView;
String name;
@Override
protected void onCreate(Bundle savedInstanceState) {
// TODO Auto-generated method stub
super.onCreate(savedInstanceState);
setContentView(R.layout.show);
contactlistView = (ListView) findViewById(R.id.ContactlistView);
listname = new ArrayList<String>();
// list_no = new ArrayList<String>();
ContentResolver cr = getContentResolver();
Cursor cur = cr.query(ContactsContract.Contacts.CONTENT_URI, null,
null, null, null);
if (cur.getCount() > 0) {
while (cur.moveToNext()) {
String id = cur.getString(cur
.getColumnIndex(ContactsContract.Contacts._ID));
String name = cur
.getString(cur
.getColumnIndex(ContactsContract.Contacts.DISPLAY_NAME));
if (Integer
.parseInt(cur.getString(cur
.getColumnIndex(ContactsContract.Contacts.HAS_PHONE_NUMBER))) > 0) {
Cursor pCur = cr.query(
ContactsContract.CommonDataKinds.Phone.CONTENT_URI,
null,
ContactsContract.CommonDataKinds.Phone.CONTACT_ID
+ " = ?", new String[] { id }, null);
while (pCur.moveToNext()) {
// Do something with phones
String phoneNo = pCur
.getString(pCur
.getColumnIndex(ContactsContract.CommonDataKinds.Phone.NUMBER));
listname.add(name + "\n" + phoneNo);
// list_no.add(phoneNo);
}
pCur.close();
}
}
}
contactlistView.setAdapter(new Contact(this));
}
class Contact extends BaseAdapter {
Context myContext;
public Contact(AddFromContacts contactActivity) {
// TODO Auto-generated constructor stub
this.myContext = contactActivity;
}
@Override
public int getCount() {
// TODO Auto-generated method stub
return listname.size();
}
@Override
public Object getItem(int arg0) {
// TODO Auto-generated method stub
return arg0;
}
@Override
public long getItemId(int arg0) {
// TODO Auto-generated method stub
return arg0;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
if (convertView == null) {
inflater = (LayoutInflater) myContext
.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
convertView = inflater.inflate(R.layout.checkbox, null);
final ViewHolder viewHolder = new ViewHolder();
viewHolder.text_name = (TextView) convertView
.findViewById(R.id.name);
viewHolder.id = (TextView) convertView.findViewById(R.id.id);
viewHolder.checkBox = (CheckBox) convertView
.findViewById(R.id.checkBox);
convertView.setTag(viewHolder);
}
final ViewHolder holder = (ViewHolder) convertView.getTag();
// holder.text_name.setText(list_no.get(position));
holder.id.setText(listname.get(position));
if (holder != null) {
holder.checkBox
.setOnCheckedChangeListener(new OnCheckedChangeListener() {
public void onCheckedChanged(
CompoundButton buttonView, boolean isChecked) {
// TODO Auto-generated method stub
// Toast.makeText(myContext, name + " Selected",
// Toast.LENGTH_LONG).show();
}
});
}
return convertView;
}
}
class ViewHolder {
TextView text_name, id;
CheckBox checkBox;
// EditText search;
}
}
but i am facing problem with this at the time of select contacts from list it select those contacts from the list which are not selected by me
Display.java
public class Display extends Activity implements OnItemClickListener{
List<String> name1 = new ArrayList<String>();
List<String> phno1 = new ArrayList<String>();
MyAdapter ma ;
Button select;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.display);
getAllContacts(this.getContentResolver());
ListView lv= (ListView) findViewById(R.id.lv);
ma = new MyAdapter();
lv.setAdapter(ma);
lv.setOnItemClickListener(this);
lv.setItemsCanFocus(false);
lv.setTextFilterEnabled(true);
// adding
select = (Button) findViewById(R.id.button1);
select.setOnClickListener(new OnClickListener()
{
@Override
public void onClick(View v) {
StringBuilder checkedcontacts= new StringBuilder();
for(int i = 0; i < name1.size(); i++)
{
if(ma.mCheckStates.get(i)==true)
{
checkedcontacts.append(name1.get(i).toString());
checkedcontacts.append("\n");
}
else
{
}
}
Toast.makeText(Display.this, checkedcontacts,1000).show();
}
});
}
@Override
public void onItemClick(AdapterView<?> arg0, View arg1, int arg2, long arg3) {
// TODO Auto-generated method stub
ma.toggle(arg2);
}
public void getAllContacts(ContentResolver cr) {
Cursor phones = cr.query(ContactsContract.CommonDataKinds.Phone.CONTENT_URI, null,null,null, null);
while (phones.moveToNext())
{
String name=phones.getString(phones.getColumnIndex(ContactsContract.CommonDataKinds.Phone.DISPLAY_NAME));
String phoneNumber = phones.getString(phones.getColumnIndex(ContactsContract.CommonDataKinds.Phone.NUMBER));
name1.add(name);
phno1.add(phoneNumber);
}
phones.close();
}
class MyAdapter extends BaseAdapter implements CompoundButton.OnCheckedChangeListener
{ private SparseBooleanArray mCheckStates;
LayoutInflater mInflater;
TextView tv1,tv;
CheckBox cb;
MyAdapter()
{
mCheckStates = new SparseBooleanArray(name1.size());
mInflater = (LayoutInflater)Display.this.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
}
@Override
public int getCount() {
// TODO Auto-generated method stub
return name1.size();
}
@Override
public Object getItem(int position) {
// TODO Auto-generated method stub
return position;
}
@Override
public long getItemId(int position) {
// TODO Auto-generated method stub
return 0;
}
@Override
public View getView(final int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
View vi=convertView;
if(convertView==null)
vi = mInflater.inflate(R.layout.row, null);
tv= (TextView) vi.findViewById(R.id.textView1);
tv1= (TextView) vi.findViewById(R.id.textView2);
cb = (CheckBox) vi.findViewById(R.id.checkBox1);
tv.setText("Name :"+ name1.get(position));
tv1.setText("Phone No :"+ phno1.get(position));
cb.setTag(position);
cb.setChecked(mCheckStates.get(position, false));
cb.setOnCheckedChangeListener(this);
return vi;
}
public boolean isChecked(int position) {
return mCheckStates.get(position, false);
}
public void setChecked(int position, boolean isChecked) {
mCheckStates.put(position, isChecked);
}
public void toggle(int position) {
setChecked(position, !isChecked(position));
}
@Override
public void onCheckedChanged(CompoundButton buttonView,
boolean isChecked) {
// TODO Auto-generated method stub
mCheckStates.put((Integer) buttonView.getTag(), isChecked);
}
}
}
display.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<ListView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_above="@+id/button1"
android:id="@+id/lv"/>
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:text="Select" />
</RelativeLayout>
row.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:layout_marginLeft="15dp"
android:layout_marginTop="34dp"
android:text="TextView" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBottom="@+id/checkBox1"
android:layout_alignLeft="@+id/textView1"
android:text="TextView" />
<CheckBox
android:id="@+id/checkBox1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:layout_below="@+id/textView1"
android:layout_marginRight="22dp"
android:layout_marginTop="23dp" />
</RelativeLayout>
Explanation:
The above uses a Custom Adapter. A custom layout row.xml
with 2 textviews and one checkbox is inflated for each row. getAllContacts()
will get all contacts from the contacts list and you store them in a list.
Custom Adapter displays the items in a custom layout inflated for each row.
When you check the check box and click display displays a toast with selected contacts name.
Use a view holder for smooth scrolling and performance. I have not used a view holder.
http://developer.android.com/training/improving-layouts/smooth-scrolling.html
Snap shot
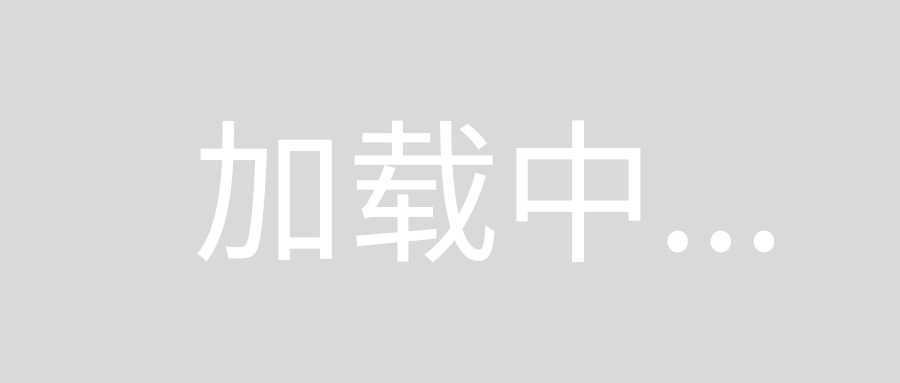