In an app that uses a NSStatusItem
with a custom view like this:
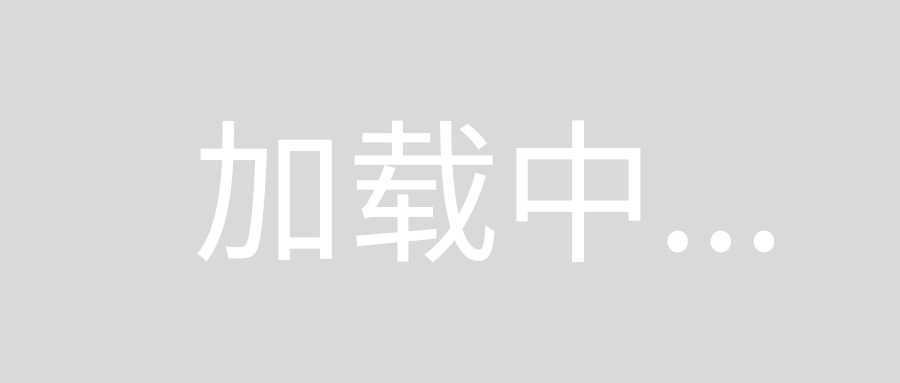
... how can you get notifications when:
- The status bar gets hidden because of a full screen app
- The status item moves position because another item is added/removed/resized?
Both are necessary to move the custom view to the right position when the item changes places.
There is a method -[NSStatusItem setView:]
. When you set a custom view for your status item, this view is automatically inserted into a special status bar window. And you can access that window using a method -[NSView window]
to observe its NSWindowDidMoveNotification
:
- (void)applicationDidFinishLaunching:(NSNotification *)notification
{
NSStatusItem *statusItem = [self newStatusItem];
NSView *statusItemView = [self newStatusItemView];
statusItem.view = statusItemView;
NSNotificationCenter *dnc = [NSNotificationCenter defaultCenter];
[dnc addObserver:self selector:@selector(statusBarDidMove:)
name:NSWindowDidMoveNotification object:statusItemView.window];
}
- (void)statusBarDidMove:(NSNotification *)note
{
NSWindow *window = note.object;
NSLog(@"%@", NSStringFromRect(window.frame)); // i.e. {{1159, 900}, {24, 22}}
}
You will receive the notification every time the status bar becomes visible or hidden and when your icon is moved. This is your chance to update a location of your popup panel.