I have RelativeLayout
like this:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
android:id="@+id/contacts"
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_weight="0.2"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<ImageView
android:id="@+id/image"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:adjustViewBounds="true"
android:contentDescription="@string/content_description_contacts"
android:scaleType="fitXY"
android:src="@drawable/contacts" />
<TextView
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_alignBottom="@id/image"
android:paddingBottom="10dp"
android:textColor="@drawable/text_color"
android:text="@string/button_contacts"
android:textSize="12sp" />
</RelativeLayout>
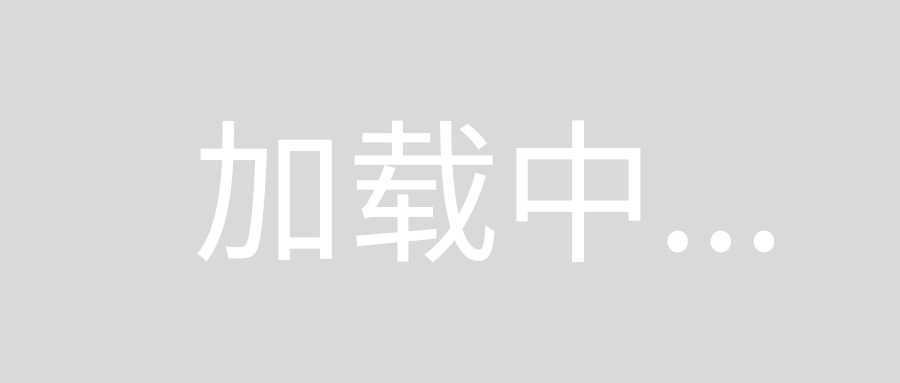
and seems like:
My contacts
Selector seems:
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_pressed="true"
android:drawable="@drawable/contacts_over" />
<item android:state_selected="true"
android:drawable="@drawable/contacts_selected" />
<item
android:drawable="@drawable/contacts_default" />
</selector>
As you can see I have 3 images: by default, selected and pressed.
But I have a problem: only default and state_selected
images are work as expected, but state_pressed
dosn't seem to work.
I have several above mentioned RelativeLayouts
and no one works with state_pressed
.
Does anybody know where is my problem?
Thanks!
make sure your RelativeLayout is clickable
You could also set android:addStatesFromChildren="true"
in your RelativeLayout
instead of the android:clickeable="true"
. If your children are already clickeable, focusable, etc. You shouldn't make your RelativeLayout
clickeable or focusable.
Try to add to your ImageView android:clickable="true"
In my opinion you should use Button
and create selector for it instead of making custom button by creating RelativeLayout
and putting there ImageView
and TextView
.
Then you can use android:drawableTop="@drawable/your_contact_icon"
. Afterwards you can check if your selector works fine.
hope these helps someone;
- make sure view clickable. descendants can block click event. more info search the below attributes.
android:clickable, android:descendantFocusability, android:focusable, android:focusableInTouchMode
in style xml you should define item element state attributes or make sure the item with no attribute must be at the end.
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_pressed="true">
<shape>
<solid android:color="@color/lightGold"/>
<stroke android:width="1dp" android:color="@color/lightGrey"/>
<padding android:bottom="@dimen/padding1" android:left="@dimen/padding1" android:right="@dimen/padding1" android:top="@dimen/padding1"/>
</shape>
</item>
<item>
<shape>
<solid android:color="@color/white_two"/>
<stroke android:width="1dp" android:color="@color/lightGrey"/>
<padding android:bottom="@dimen/padding1" android:left="@dimen/padding1" android:right="@dimen/padding1" android:top="@dimen/padding1"/>
</shape>
</item>
But this sample will not work;
<?xml version="1.0" encoding="utf-8"?><selector xmlns:android="http://schemas.android.com/apk/res/android">
<item>
<shape>
<solid android:color="@color/white_two"/>
<stroke android:width="1dp" android:color="@color/lightGrey"/>
<padding android:bottom="@dimen/padding1" android:left="@dimen/padding1" android:right="@dimen/padding1" android:top="@dimen/padding1"/>
</shape>
</item>
<item android:state_pressed="true">
<shape>
<solid android:color="@color/lightGold"/>
<stroke android:width="1dp" android:color="@color/lightGrey"/>
<padding android:bottom="@dimen/padding1" android:left="@dimen/padding1" android:right="@dimen/padding1" android:top="@dimen/padding1"/>
</shape>
</item>
or you can define state attribute for both item. So order will be not important.
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_pressed="false">
<shape>
<solid android:color="@color/white_two"/>
<stroke android:width="1dp" android:color="@color/lightGrey"/>
<padding android:bottom="@dimen/padding1" android:left="@dimen/padding1" android:right="@dimen/padding1" android:top="@dimen/padding1"/>
</shape>
</item>
<item android:state_pressed="true">
<shape>
<solid android:color="@color/lightGold"/>
<stroke android:width="1dp" android:color="@color/lightGrey"/>
<padding android:bottom="@dimen/padding1" android:left="@dimen/padding1" android:right="@dimen/padding1" android:top="@dimen/padding1"/>
</shape>
</item>
</selector>
Ref;
During each state change, the state list is traversed top to bottom
and the first item that matches the current state is used—the
selection is not based on the "best match," but simply the first item
that meets the minimum criteria of the state
State List
https://developer.android.com/guide/topics/resources/drawable-resource.html