Want to improve this question? Update the question so it focuses on one problem only by editing this post.
Closed 4 years ago.
Improve this question
Hey i want to make a banner or ribbon similar to given photo with a textview or any layout in android using xml or java. I cant able to figure it out how to do that.
So please help me out its really needed.
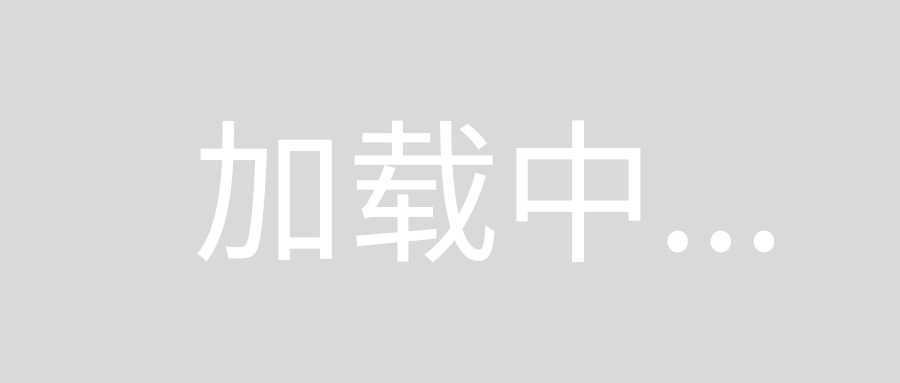
To create a banner like that You need to do these things
- Create a Shape drawable of an Inverted right angled triangle by tweaking this code
- Set the background of the textView to this shape
- Change the textView Gravity to right/end align
Here's a simple imageview with the text banner in the corner which I wrote for my own use. The same structure could be applied to any type of view.
public class BannerImageView extends ImageView {
private Paint mRibbonPaint;
private Paint mTextPaint;
private Paint mBoxPaint;
private float mScale;
private String mBannerText;
public BannerImageView(Context context, AttributeSet attrs) {
super(context, attrs);
initPainters(attrs);
}
public BannerImageView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
initPainters(attrs);
}
private void initPainters(AttributeSet attrs) {
TypedArray attributes = getContext().getTheme().obtainStyledAttributes(attrs, R.styleable.BannerImageView, defStyleAttr, 0);
mBannerText = attributes.getString(R.styleable.BannerImageView_label);
mBoxPaint = new Paint();
int white = ContextCompat.getColor(getContext(), R.color.white);
mBoxPaint.setColor(white);
mBoxPaint.setAlpha(156);
mRibbonPaint = new Paint();
mRibbonPaint.setColor(ContextCompat.getColor(getContext(), R.color.banner_color));
mRibbonPaint.setAntiAlias(true);
mRibbonPaint.setStyle(Paint.Style.STROKE);
mRibbonPaint.setStrokeCap(Paint.Cap.BUTT);
mScale = getResources().getDisplayMetrics().density;
mRibbonPaint.setStrokeWidth(dp(16));
mTextPaint = new Paint();
mTextPaint.setColor(white);
mTextPaint.setTextSize( dp(12) );
}
/**
* Converts dp to px
* @param dp
* @return
*/
private float dp(float dp) {
return dp * mScale + 0.5f;
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
if ( !TextUtils.isEmpty(mBannerText) ) {
canvas.drawRect(0, 0, getRight(), getBottom(), mBoxPaint);
canvas.drawLine(-dp(16), dp(64), dp(64), -dp(16), mRibbonPaint);
canvas.save();
canvas.rotate(-45, 0, 0);
canvas.drawText(mBannerText, -dp(24), dp(38), mTextPaint);
canvas.restore();
}
}
Then declare the extra attributes you want to use in values/attrs.xml
<declare-styleable name="BannerImageView">
<attr name="label" format="string"/>
</declare-styleable>
Of course you use in your XML layouts as usual but remember the app namespace for the custom attributes
<com.my.package.BannerImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:label="Sold Out" />