I'm initializing Hibernate without any XML by something like
org.hibernate.SessionFactory sessionFactory =
new org.hibernate.cfg.Configuration().
.setProperty(...)
.setProperty(...)
...
.buildSessionFactory();
My classes use an ID like
@Id @Generated(GenerationTime.INSERT) @GeneratedValue private Integer id;
The generator used is SequenceStyleGenerator
, which seems to be the replacement for the deprecated SequenceGenerator
and SequenceHiLoGenerator
and whatever. It uses
public static final int DEFAULT_INCREMENT_SIZE = 1;
and seems to allow configuration via
public static final String INCREMENT_PARAM = "increment_size";
but that's all I could find out. I guess I have to set some property "xxx.yyy.increment_size" or pass it in another way to Hibernate, but I can't see how.
I'm aware of @SequenceGenerator
, but it seems to be completely ignored
I guess you are looking for how to set increment_size
property for your SequenceSytleGenerator
.
Sample snippet below setting increment_size
using @GenericGenerator
annotation with hilo
optimizer and SEQUENCE
strategy.
@GeneratedValue(strategy = GenerationType.SEQUENCE, generator = "hilo_generator")
@GenericGenerator(
name = "hilo_generator",
strategy = "org.hibernate.id.enhanced.SequenceStyleGenerator",
parameters = {
// Or leave it out to get "hibernate_sequence".
@Parameter(name = "sequence_name", value = "hilo_sequence"),
// Or leave it out as this is the default.
@Parameter(name = "initial_value", value = "1"),
@Parameter(name = "increment_size", value = "5"),
@Parameter(name = "optimizer", value = "hilo")
})
There's no way you can globally set the DEFAULT_INCREMENT_SIZE
with a Hibernate configuration property. You need to use the @Id
configuration properties instead.
@Generated vs @GeneratedValue
You don't need to use @Generated
along with @GeneratedValue
. The @Generated
annotation is for non-id entity attributes that are generated by the database during INSERT or UPDATE. For more details about the @Generated
annotation, check out this article.
On the other hand, the @GeneratedValue
is only for the entity identifier attributes, and it's what you need to use when the entity identifier is generated automatically upon persisting an entity.
Sequence generator
The sequence generator requires an extra database roundtrip to call the sequence object when you persist the entity. For this reason, Hibernate offers the sequence-based optimizers to reduce the number of roundtrips needed to fetch entity identifier values.
Now, if you want to use hilo
, the identifier mapping will look as follows:
@Id
@GeneratedValue(
strategy = GenerationType.SEQUENCE,
generator = "post_sequence"
)
@GenericGenerator(
name = "post_sequence",
strategy = "sequence",
parameters = {
@Parameter(name = "sequence_name", value = "post_sequence"),
@Parameter(name = "initial_value", value = "1"),
@Parameter(name = "increment_size", value = "3"),
@Parameter(name = "optimizer", value = "hilo")
}
)
private Long id;
Apart from having to use the Hibernate-specific @GenericGenerator
, the problem with hilo
is that the generated identifiers don't include the database sequence value, so an 3rd-party client using the database will not know how to generate the next identifier value unless they know the hilo
algorithm and the allocationSize
.
For this reason, it's better to use pooled
or pooled-lo
.
Pooled optimizer
The pooled
optimizer is very easy to set up. All you need to do is set the allocationSize
of the JPA @SequenceGenerator
annotation, and Hibernate is going to switch to using the pooled
optimizer:
@Id
@GeneratedValue(
strategy = GenerationType.SEQUENCE,
generator = "post_sequence"
)
@SequenceGenerator(
name = "post_sequence",
sequenceName = "post_sequence",
allocationSize = 3
)
private Long id;
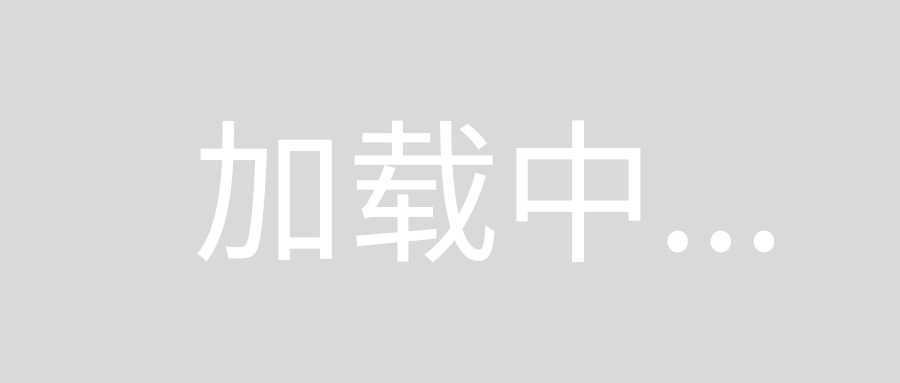
Pooled-lo optimizer
To use the pooled-lo optimizer, just add the following configuration property:
<property name="hibernate.id.optimizer.pooled.preferred" value="pooled-lo" />
Now, the entity identifier mapping is identical to the one I showed you before for the pooled
optimizer.
To understand how the pooled-lo works, check out this diagram:
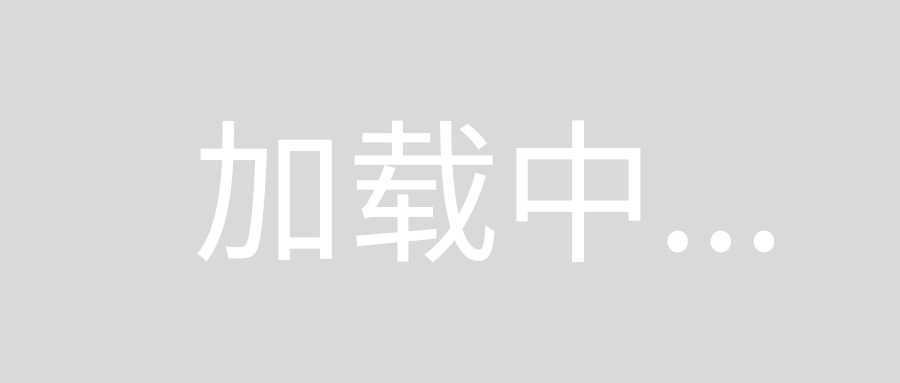
If you have been using the legacy hilo
optimizer, you might want to switch to using pooled
or pooled-lo
, as hilo
is not interoperable with other clients that might not be aware of the hilo
identifier allocation strategy.
For more details about how you can migrate from hilo
to pooled
or pooled-lo
, check out this article.