Consider the follwing plot:
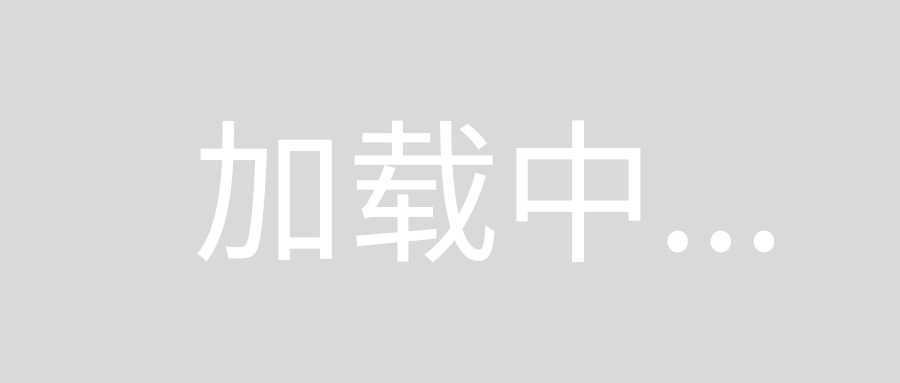
produced by this function:
def timeDiffPlot(dataA, dataB, saveto=None, leg=None):
labels = list(dataA["graph"])
figure(figsize=screenMedium)
ax = gca()
ax.grid(True)
xi = range(len(labels))
rtsA = dataA["running"] / 1000.0 # running time in seconds
rtsB = dataB["running"] / 1000.0 # running time in seconds
rtsDiff = rtsB - rtsA
ax.scatter(rtsDiff, xi, color='r', marker='^')
ax.scatter
ax.set_yticks(range(len(labels)))
ax.set_yticklabels(labels)
ax.set_xscale('log')
plt.xlim(timeLimits)
if leg:
legend(leg)
plt.draw()
if saveto:
plt.savefig(saveto, transparent=True, bbox_inches="tight")
What matters here is the positive or negative difference of the values to x = 0
. It would be nice to visualize this more clearly, e.g.
- emphasize the x=0 axis
- draw a line from x=0 to the plot marker
Can this be done with matplotlib? What code would need to be added?
As pointed out by Rutger Kassies, there are actually some "stem" functions that automate the "manual" method from my other answer. The function for horizontal stem lines is hlines()
(vlines()
is for vertical stem bars):
import numpy
from matplotlib import pyplot
x_arr = numpy.random.random(10)-0.5; y_arr = numpy.arange(10)
pyplot.hlines(y_arr, 0, x_arr, color='red') # Stems
pyplot.plot(x_arr, y_arr, 'D') # Stem ends
pyplot.plot([0, 0], [y_arr.min(), y_arr.max()], '--') # Middle bar
The documentation for hlines()
is on the Matplotlib website.
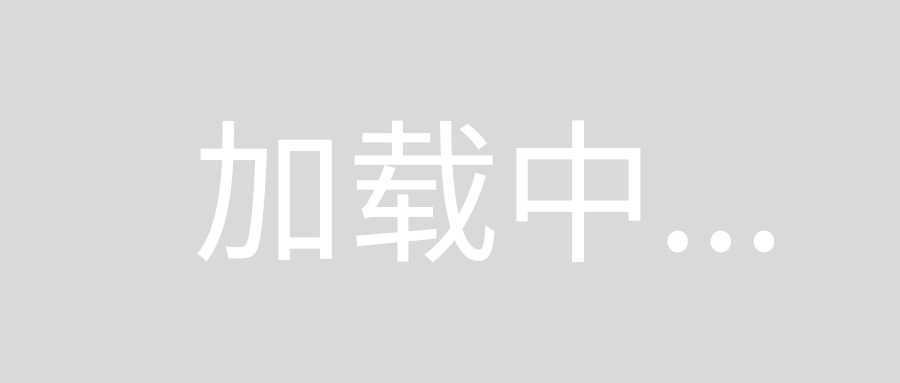
(See my other answer, for a faster solution.)
Matplotlib offers vertical "stem" bars: http://matplotlib.org/api/pyplot_api.html#matplotlib.pyplot.stem. However, I can't find a horizontal equivalent of stem()
.
One can nonetheless draw horizontal stem bars quite easily, through repeated plot()
calls (one for each stem). Here is an example
import numpy
from matplotlib.pyplot import plot
x_arr = numpy.random.random(10)-0.5; y_arr = numpy.arange(10)
# Stems:
for (x, y) in zip(x_arr, y_arr):
plot([0, x], [y, y], color='red')
# Stem ends:
plot(x_arr, y_arr, 'D')
# Middle bar:
plot([0, 0], [y_arr.min(), y_arr.max()], '--')
with the following result:
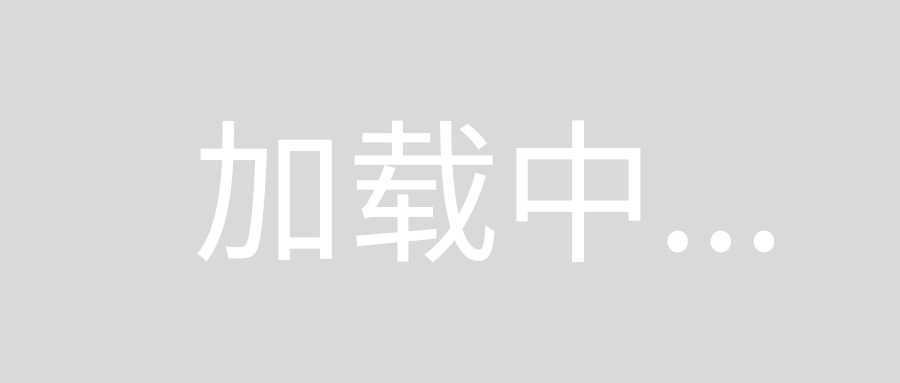
Note, however, that drawing bars from x = 0 does not make sense when x is on a log scale, as David Zwicker pointed out, since x = 0 is infinitely far on the left of the x axis.