I'm trying to merge 2 dataframes, but for some reason it's throwing KeyError: Player_Id
I'm trying to merge on Striker_Id
and Player_Id
This is how my Dataframe looks like
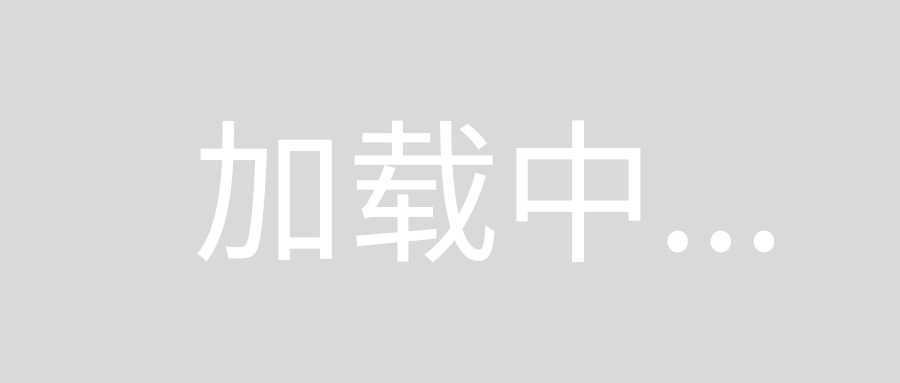
Merge Code:
player_runs.merge(matches_played_by_players,left_on='Striker_Id',right_on='Player_Id',how='left')
What am I doing wrong?
Hmm, from looking at your problem, it seems like you're trying to merge on the indexes, but you treat them as columns? Try changing your merge code a bit -
player_runs.merge(matches_played_by_players,
left_index=True,
right_index=True,
how='left')
Furthermore, make sure that both indexes are of the same type (in this case, consider str
int
?)
player_runs.index = player_runs.index.astype(int)
And,
matches_played_by_players.index = matches_played_by_players.index.astype(int)
you're basically merging on none existing columns. this is because reset_index
creates a new data-frame rather than changing the data frame it's applied to. setting the parameter inplace=True
when using reset_index should resolve this issue, alternatively merge on the index of each data-frame. i.e.
pd.merge(df1,df2,left_index=True,right_index=True,how='left')