Here's the thing: my app needs a series of tutorial screens at the beginning when people launch it, along with two buttons on the bottom of the screen to let people register or login. (like the image shows)
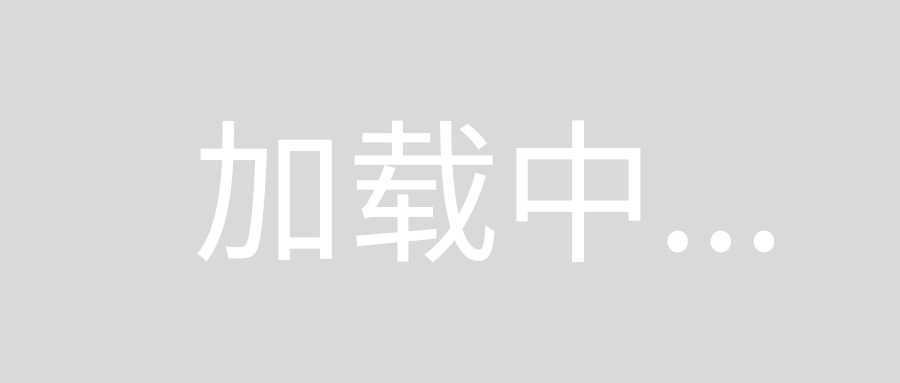
My question is : how can I keep the buttons on the screen when people slide the tutorial images ?
I tried several ways:
In PageContentViewController (subclass of UIViewController), I have a UIImageView for the tutorial image, and two UIButton for the buttons. This way, the buttons also get slide, not what I want.
I use a new ViewController for the buttons, and in my rootviewController, I add this new viewcontroller as a subview. This way, the new viewcontroller hides the tutorial images underneath it. I can't slide the image. I also tried to set the alpha value of the new viewcontroller, so that I can see the images underneath it, but still can't slide it.
Any better ideas or sample code I can learn from ?
This is very simple. The trick is to add the buttons "on top of " your page view controller view. That is, after you add the page view controller, you can use the sendSubviewToBack method to ensure that the buttons remain in the foreground while you will be able to swipe between the different view controllers of your page view controller. You can understand this behaviour by simply following these steps:
- Open Xcode.
- Create a New Project and select "Page-Based Application".
Xcode will create a basic app with a UIPageViewController implementation in the RootViewController. In RootViewController s viewDidLoad method, you will see the following code:
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
// Configure the page view controller and add it as a child view controller.
self.pageViewController = [[UIPageViewController alloc] initWithTransitionStyle:UIPageViewControllerTransitionStyleScroll navigationOrientation:UIPageViewControllerNavigationOrientationHorizontal options:nil];
self.pageViewController.delegate = self;
DataViewController *startingViewController = [self.modelController viewControllerAtIndex:0 storyboard:self.storyboard];
NSArray *viewControllers = @[startingViewController];
[self.pageViewController setViewControllers:viewControllers direction:UIPageViewControllerNavigationDirectionForward animated:NO completion:nil];
self.pageViewController.dataSource = self.modelController;
[self addChildViewController:self.pageViewController];
[self.view addSubview:self.pageViewController.view];
// Set the page view controller's bounds using an inset rect so that self's view is visible around the edges of the pages.
CGRect pageViewRect = self.view.bounds;
self.pageViewController.view.frame = pageViewRect;
[self.pageViewController didMoveToParentViewController:self];
// Add the page view controller's gesture recognizers to the book view controller's view so that the gestures are started more easily.
self.view.gestureRecognizers = self.pageViewController.gestureRecognizers;
}
Now Take the Main.storyboard and you will see the RootViewController Scene.Add a UIButton to its view using the interface builder.
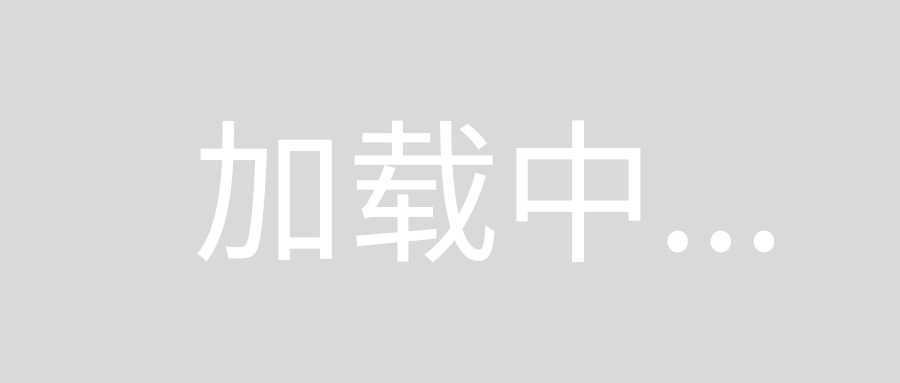
Now add the following line at the end of the viewDidLoad method.
[self.view sendSubviewToBack:self.pageViewController.view];
And give it a run! you will see that it is just what you wanted.
See the screenshots of the output:
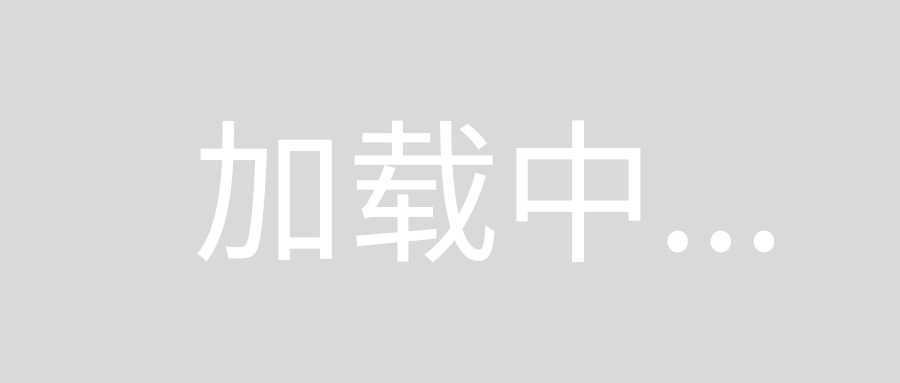