Consider these two pink square:
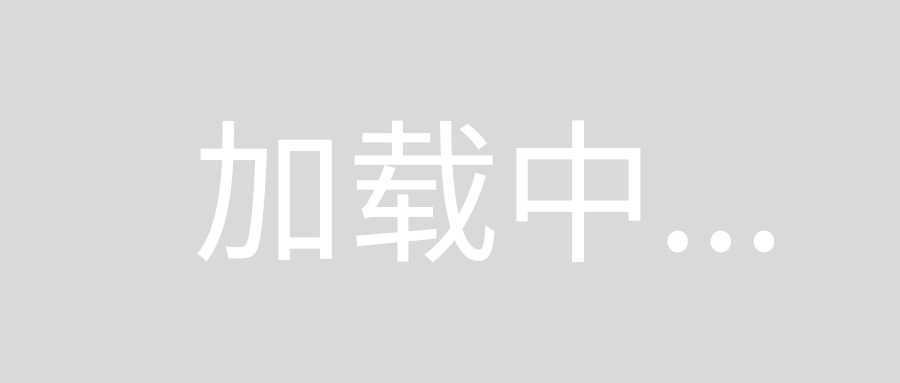
And this:
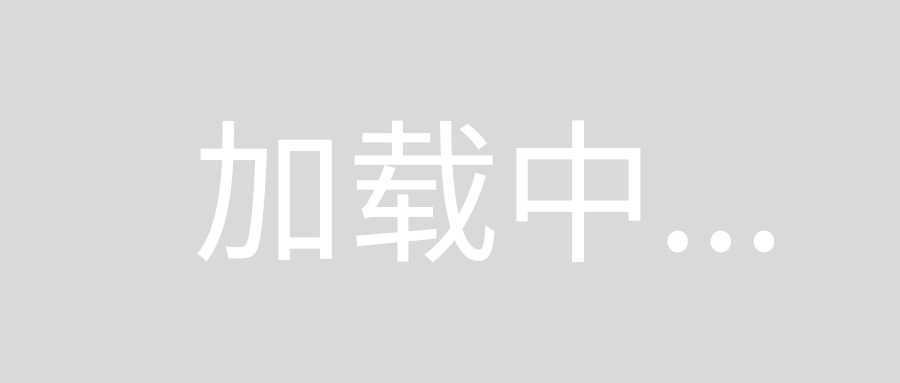
As you may know, one is lighter and one is darker or more sharp.
The problem is, I can tell it by human eyes, but is this possible to use a system way or programme way to detect this information? At least, is this possible to have a value that tell me that colour is more like white or that colour is less like white? (Assume I got the RGB code of that colour.) Thanks.
Since you haven't specified any particular language/script to detect the darker/lighter hex, I would like to contribute a PHP solution to this
Demo
$color_one = "FAE7E6"; //State the hex without #
$color_two = "EE7AB7";
function conversion($hex) {
$r = hexdec(substr($hex,0,2)); //Converting to rgb
$g = hexdec(substr($hex,2,2));
$b = hexdec(substr($hex,4,2));
return $r + $g + $b; //Adding up the rgb values
}
echo (conversion($color_one) > conversion($color_two)) ? 'Color 1 Is Lighter' : 'Color 1 Is Darker';
//Comparing the two converted rgb, the greater one is darker
As pointed out by @Some Guy, I have modified my function for yielding a better/accurate result... (Added Luminance)
function conversion($hex) {
$r = 0.2126*hexdec(substr($hex,0,2)); //Converting to rgb and multiplying luminance
$g = 0.7152*hexdec(substr($hex,2,2));
$b = 0.0722*hexdec(substr($hex,4,2));
return $r + $g + $b;
}
Demo 2
Below is the Python code to determine light or dark color. The formulation is based on the HSP value. HSP (Highly Sensitive Poo) equation is from http://alienryderflex.com/hsp.html used to determine whether the color is light or dark.
import math
def isLightOrDark(rgbColor=[0,128,255]):
[r,g,b]=rgbColor
hsp = math.sqrt(0.299 * (r * r) + 0.587 * (g * g) + 0.114 * (b * b))
if (hsp>127.5):
return 'light'
else:
return 'dark'