I have a UITableViewController
with a UISearchDisplayController
(and UISearchBar
) that is contained in a UINavigationController
as the root element. Is it possible to configure it so the UISearchBar
appears in place of the UINavigationBar
? I don't think hiding the navigation bar will work, a the next screen (pushed on) requires it to be visible (and this will create a strange animation glitch).
I'm basically going for a screen like the App Store search tab.
I've uploaded a sample screenshots of how it looks now:
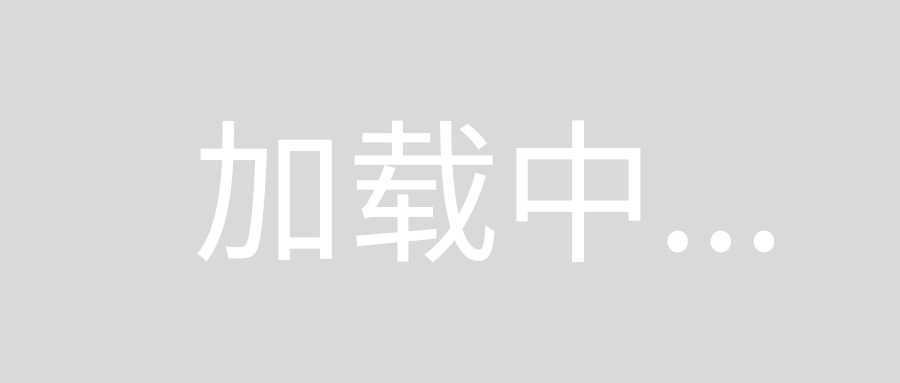
Assign yourself as the delegate of the UINavigationController and implement - (void)navigationController:(UINavigationController *)navigationController willShowViewController:(UIViewController *)viewController animated:(BOOL)animated
.
Then use the passed in navigationController to hide the navigation bar.
[navigationController setNavigationBarHidden:YES animated:animated]
Edit: Come to think of it, it would be better to pass the animated value into -setNavigationBarHidden:animated:
as well. Code updated.
Here is my solution:
You don't need to hide the UINavigationBar, instead, you could merge the UISearchBar into UINavigationBar.
In YourClass.m file:
1. add a UISearchBar property
2. add the UISearchBar into the NavigationItem in the viewDidLoad section.
code:
@interface YourClass ()
@property (weak, nonatomic) IBOutlet UISearchBar *searchBar;
@end
@implementation YourClass
- (void)viewDidLoad
{
[super viewDidLoad];
// Reveal cancel button in UISearchBar.
searchBar.showsCancelButton = YES;
// Add UISearchBar as titleView of the UINavigationBar.
self.navigationItem.titleView = searchBar;
}
last, don't forget to edit your .xib file. Just add the UISearchBar object right down the UINavigationBar and connect the referencing outlets of the UISearchBar to the File's Owner.
Good luck!
I guess you can hide your navigationbar by setting the 'navigationBarHidden' property to true.
[navigationController setNavigationBarHidden:YES animated:NO];
In the viewDidLoad method of the controller you are pushing
[[self navigationController] setNavigationBarHidden:NO animated:YES];