I have a TabBarView in my main.dart and every tab got a class to show the content(it's listview object), when i go between the tabs, the listview page refresh everytime, is it normal for tabbarview? I don't expect it will refresh everytime when i go between the tabs.
is it the problem my class? how to fix this? the code is something like this.
class ListWidget extends StatefulWidget {
final catID;
ListWidget(this.catID);
_ListWidgetState createState() => new _ListWidgetState(catID);
}
class _ListWidgetState extends State<ListWidget> {
var catID;
void initState() {
super.initState();
_fetchListData();
}
@override
Widget build(BuildContext context) {
// TODO: implement build
return new Scaffold(.......
}
If I understood you well, you are complaining about the refreshing because you need the views to save their states after moving between tabs. There is an open issue on the subject and there is a way around this problem mentioned in the comments.
Update:
There is a workaround for this issue by using AutomaticKeepAliveClientMixin
which you can learn more about in this article.
MahMoos is right, however it's good to have an example here ...
- Use AutomaticKeepAliveClientMixin
- override wantKeepAlive property and return true
`
class ListWidget extends StatefulWidget {
@override
_ListWidgetState createState() => _ListWidgetState();
}
class _ListWidgetState extends State<ListWidget> with
AutomaticKeepAliveClientMixin<ListWidget>{ // ** here
@override
Widget build(BuildContext context) {
return Container();
}
@override
bool get wantKeepAlive => true; // ** and here
}
If you want that your Tab view data does not refresh when you change Tab you should use
AutomaticKeepAliveClientMixin
class BaseScreen extends StatefulWidget {
BaseScreen(this.title, this.listener, {Key key}) : super(key: key);
}
class BaseScreenState extends State<BaseScreen> with AutomaticKeepAliveClientMixin {
@override
Widget build(BuildContext context) {
screenWidth = MediaQuery.of(context).size.width;
screenHeight = MediaQuery.of(context).size.height;
primaryColor = Theme.of(context).primaryColor;
textTheme = Theme.of(context).textTheme;
return Scaffold(
key: scaffoldKey,
appBar: getAppBar(),
body: Container(),
);
}
@override
bool get wantKeepAlive => true;
}
I was facing the same issue and this tutorial helped me.
Happy Coding.
For doing this : Use of the mixin
and AutomaticKeepAliveClientMixin
on our State.
And Implement : bool get wantKeepAlive => true;
Note: in this example wantKeepAlive => true
for first tab and wantKeepAlive => false
for second tab. So you can see very well how it works.
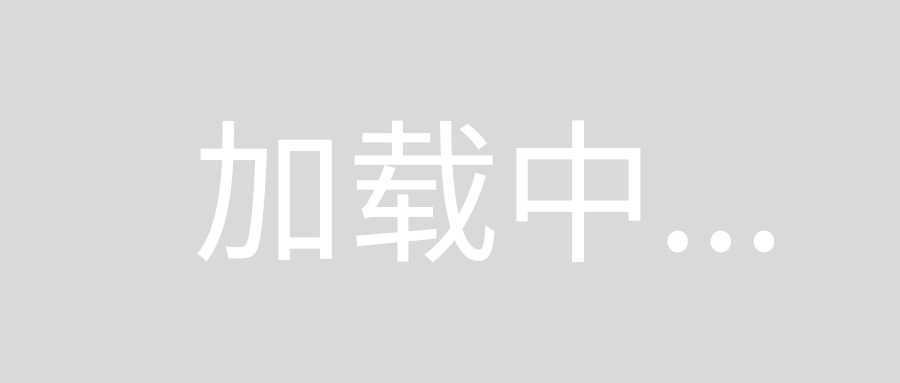
class ResidentListScreen extends StatefulWidget {
@override
_ResidentListScreenState createState() => _ResidentListScreenState();
}
class _ResidentListScreenState extends State<ResidentListScreen> with
AutomaticKeepAliveClientMixin<ResidentListScreen>{
@override
bool get wantKeepAlive => true;
@override
void initState() {
super.initState();
}
@override
Widget build(BuildContext context) {
}
}