On top of this problem, I have another. I try to get binary data from a external process, but the data(a image) seems to be corrupted. The screenshot below shows the corruption: The left image was done by executing the program on command line, the right one from code.
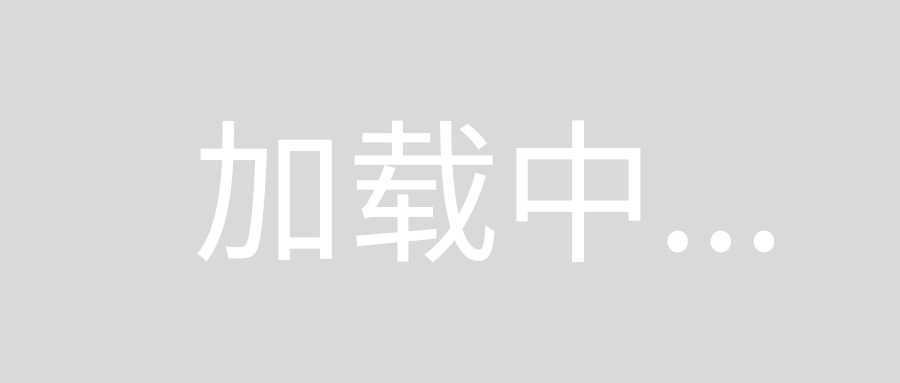
My Code so far:
var process = new Process
{
StartInfo =
{
Arguments = string.Format(@"-display"),
FileName = configuration.PathToExternalSift,
RedirectStandardError = true,
RedirectStandardInput = true,
RedirectStandardOutput = true,
UseShellExecute = false,
CreateNoWindow = true,
},
EnableRaisingEvents = true
};
process.ErrorDataReceived += (ProcessErrorDataReceived);
process.Start();
process.BeginErrorReadLine();
//Reads in pbm file.
using (var streamReader = new StreamReader(configuration.Source))
{
process.StandardInput.Write(streamReader.ReadToEnd());
process.StandardInput.Flush();
process.StandardInput.Close();
}
//redirect output to file.
using (var fileStream = new FileStream(configuration.Destination, FileMode.OpenOrCreate))
{
process.StandardOutput.BaseStream.CopyTo(fileStream);
}
process.WaitForExit();
Is this some kind of encoding problem? I used the Stream.CopyTo-Approach as mentioned here in order to avoid there problems.
I found the problem. The redirection of the output was correct, the reading of the input seems to be the problem. So I changed the code from:
using (var streamReader = new StreamReader(configuration.Source))
{
process.StandardInput.Write(streamReader.ReadToEnd());
process.StandardInput.Flush();
process.StandardInput.Close();
}
to
using (var fileStream = new StreamReader(configuration.Source))
{
fileStream.BaseStream.CopyTo(process.StandardInput.BaseStream);
process.StandardInput.Close();
}
Not it works!
For all the people that might have the same problem, here the corrected code:
var process = new Process
{
StartInfo =
{
Arguments = string.Format(@"-display"),
FileName = configuration.PathToExternalSift,
RedirectStandardError = true,
RedirectStandardInput = true,
RedirectStandardOutput = true,
UseShellExecute = false,
CreateNoWindow = true,
},
EnableRaisingEvents = true
};
process.ErrorDataReceived += (ProcessErrorDataReceived);
process.Start();
process.BeginErrorReadLine();
//read in the file.
using (var fileStream = new StreamReader(configuration.Source))
{
fileStream.BaseStream.CopyTo(process.StandardInput.BaseStream);
process.StandardInput.Close();
}
//redirect output to file.
using (var fileStream = new FileStream(configuration.Destination, FileMode.OpenOrCreate))
{
process.StandardOutput.BaseStream.CopyTo(fileStream);
}
process.WaitForExit();