I'm trying to do some Cypress assertions to see whether or not it contains one or another string. It can be in English or Spanish, so either one should pass the test.
cy.get(el).should('contain', 'submit').or('contain', 'enviar')
obviously doesnt work.
const runout = ['submit', 'enviar']
const el = '[data-test=btn-submit]'
function checkArray(arr, el) {
for(let i = 0; i < arr.length; i++) {
if(cy.get(el).contains(arr[i])) {
return true
} else {
if (i === arr.length) {
return false
}
}
}
}
cy.expect(checkArray(runout,el)).to.be.true
fails the test, still checking both strings.
You can try a regular expression, see contains#Regular-Expression.
See this question Regular expression containing one word or another for some formats
I think something as simple as this will do it,
cy.get(el).contains(/submit|enviar/g)
Experiment first on Regex101 or similar online tester.
Maybe build it with
const runout = ['submit', 'enviar']
const regex = new RegExp(`${runout.join('|')}`, 'g')
cy.get(el).contains(regex)
Maybe this way is able to check 'Or' condtion.
I used google homepage to test and try to find either 'Google Search' or 'Not Google Search' in my test.
If cannot find both will throw an error.
describe('check if contains one of the text', function()
{
it('Test google search button on google homepage',()=> {
let url = 'https://www.google.com/';
let word1 = 'Not Google Search';
let word2 = 'Google Search';
cy.visit(url);
cy.get('input[value="Google Search"]').invoke('attr','value').then((text)=>{
if (text=== word1) {
console.log('found google not search');
}
else if (text === word2) {
console.log('found google search');
}
else {
console.log(text);
throw ("cannot find any of them");
}
})
})
})
In the test case above word2 is found so it logged 'Found google search' in the console.
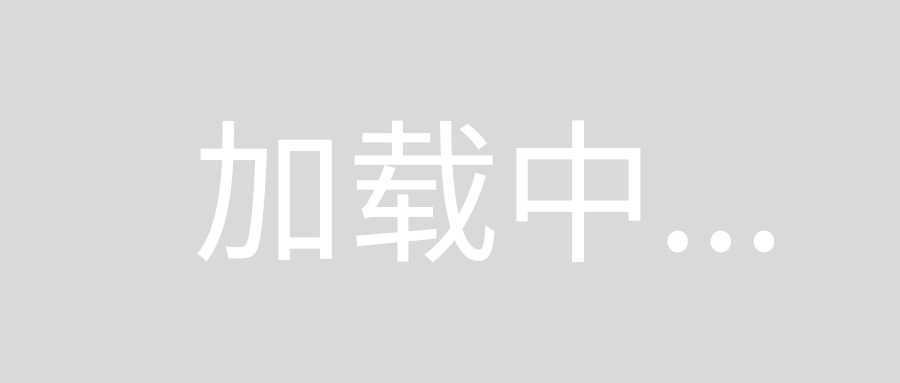