How does one programmatically change proxy settings on a mac osx. I am fluent in ios, and since mac os programming is similar there shouldn't be much problems with it. However I lack the logic needed to create proxy changes programmatically.. Manual tweaking is extremely easy. This is the network tab in System Preferences I am after:
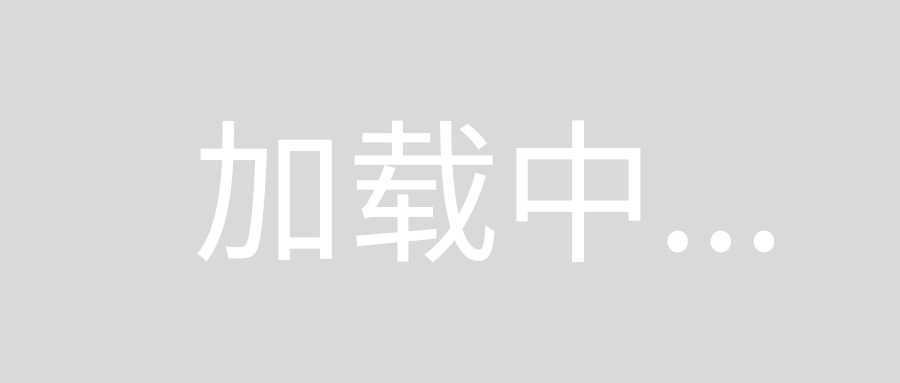
What I have tried:
let dynamicStore: SCDynamicStoreRef = SCDynamicStoreCreate(nil, "setProxy" as CFString, nil, nil)!
let updated = SCDynamicStoreSetValue(dynamicStore, "HTTPProxy" as CFStringRef, "111.222.333.1") // updated is false, indicating unsuccessful operation
Also tried this, but the dictionary returned is read only
let dynamicStore: SCDynamicStoreRef = SCDynamicStoreCreate(nil, "myFunc" as
CFString, nil, nil)!
let proxyDict = SCDynamicStoreCopyProxies(dynamicStore)
if let proxyDict = SCDynamicStoreCopyProxies(dynamicStore) as NSDictionary? {
if let port = proxyDict["HTTPPort"] as? Int {
print("HTTPPort:", port)
proxyDict["HTTPPort"] = 8088; // can't do that
}
}
I am running out of ideas.
From this answer, here is a sample script:
e=$(networksetup -getsocksfirewallproxy wi-fi | grep "No")
if [ -n "$e" ]; then
echo "Turning on proxy"
networksetup -setsocksfirewallproxystate wi-fi on
else
echo "Turning off proxy"
networksetup -setsocksfirewallproxystate wi-fi off
fi
It swiches (on/off) the SOCKS proxy for the user's Wi-Fi connection.
You can use this idea to make the script for your needs. You can implement your desired logic in a bash script like that or in Cocoa.
- Use
networksetup -listallnetworkservices
to list all connections.
- Use
networksetup -setwebproxy
to setup proxy for a particular connection.
For example, here's my Terminal output:
$ networksetup -listallnetworkservices
An asterisk (*) denotes that a network service is disabled.
MBBEthernet
Wi-Fi
Wi-Fi Modem Yota 4G LTE
Bluetooth PAN
Thunderbolt Bridge
iPhone
$ networksetup -setwebproxy some bad args
** Error: The parameters were not valid.
$ networksetup -setwebproxy Wi-Fi 1.2.3.4 8080
The last command gave the empty stdout, it means success.
See also man networksetup
.
How to execute a shell command from a Cocoa app.
The above commands executed from Terminal app asked for authorization. I'm sorry I'm not sure if you can leave it "as is" (just launch your NSTask
, OS X will do the rest), or you need to obtain proper credentials via SFAuthorization before doing that.
CFDictionaryCreateMutableCopy
would do the trick, but even after you successfully changed the dictionary, SCDynamicStoreSetMultiple
just has no effect.