I am having trouble trying to figure out how to display a transparent circle or rectangle at a desired location unique from the users location. Im a beginner with mapkit so thanks in advance.
class FirstViewController: UIViewController, MKMapViewDelegate, CLLocationManagerDelegate
{
@IBOutlet weak var mapView: MKMapView!
let locationManager = CLLocationManager()
override func viewDidLoad()
{
super.viewDidLoad()
self.locationManager.delegate = self
self.locationManager.desiredAccuracy = kCLLocationAccuracyBest
self.locationManager.requestAlwaysAuthorization()
self.locationManager.startUpdatingLocation()
self.mapView.showsUserLocation = true
}
func locationManager(manager: CLLocationManager, didUpdateLocations locations: [CLLocation])
{
let location = locations.last
let center = CLLocationCoordinate2D(latitude: location!.coordinate.latitude, longitude: location!.coordinate.longitude)
let region = MKCoordinateRegion(center: center, span: MKCoordinateSpan(latitudeDelta: 1, longitudeDelta: 1))
self.mapView.setRegion(region, animated: true)
self.locationManager.stopUpdatingLocation()//
}
func locationManager(manager: CLLocationManager, didFailWithError error: NSError)
{
print("Errors: " + error.localizedDescription)
}
}
This has been updated to support Swift 4.2. Comments are provided to explain several of the choices I made.
import UIKit
import MapKit
class Map: UIViewController {
var mapView = MKMapView()
func setup() {
// Assign delegate here. Can call the circle at startup,
// or at a later point using the method below.
// Includes <# #> syntax to simplify code completion.
mapView.delegate = self
showCircle(coordinate: <#CLLocationCoordinate2D#>,
radius: <#CLLocationDistance#>)
}
// Radius is measured in meters
func showCircle(coordinate: CLLocationCoordinate2D,
radius: CLLocationDistance) {
let circle = MKCircle(center: coordinate,
radius: radius)
mapView.addOverlay(circle)
}
}
extension Map: MKMapViewDelegate {
func mapView(_ mapView: MKMapView,
rendererFor overlay: MKOverlay) -> MKOverlayRenderer {
// If you want to include other shapes, then this check is needed.
// If you only want circles, then remove it.
if let circleOverlay = overlay as? MKCircle {
let circleRenderer = MKCircleRenderer(overlay: circleOverlay)
circleRenderer.fillColor = .black
circleRenderer.alpha = 0.1
return circleRenderer
}
// If other shapes are required, handle them here
return <#Another overlay type#>
}
}
I have achieve the following using the following
import UIKit
import MapKit
class MapVC: UIViewController,CLLocationManagerDelegate {
var locationManager = CLLocationManager()
@IBOutlet weak var mapView : MKMapView!
override func viewDidLoad() {
super.viewDidLoad()
locationManager.delegate = self
locationManager.desiredAccuracy = kCLLocationAccuracyBest
// Check for Location Services
if (CLLocationManager.locationServicesEnabled()) {
locationManager.requestAlwaysAuthorization()
locationManager.requestWhenInUseAuthorization()
}
//Zoom to user location
if let userLocation = locationManager.location?.coordinate {
let viewRegion = MKCoordinateRegion(center: userLocation, latitudinalMeters: 200, longitudinalMeters: 200)
let region = CLCircularRegion(center: userLocation, radius: 5000, identifier: "geofence")
mapView.addOverlay(MKCircle(center: userLocation, radius: 200))
mapView.setRegion(viewRegion, animated: false)
}
DispatchQueue.main.async {
self.locationManager.startUpdatingLocation()
}
}
}
extension MapVC : MKMapViewDelegate{
func mapView(_ mapView: MKMapView, rendererFor overlay: MKOverlay) -> MKOverlayRenderer {
var circleRenderer = MKCircleRenderer()
if let overlay = overlay as? MKCircle {
circleRenderer = MKCircleRenderer(circle: overlay)
circleRenderer.fillColor = UIColor.green
circleRenderer.strokeColor = .black
circleRenderer.alpha = 0.5
}
return circleRenderer
}
}
My Output:
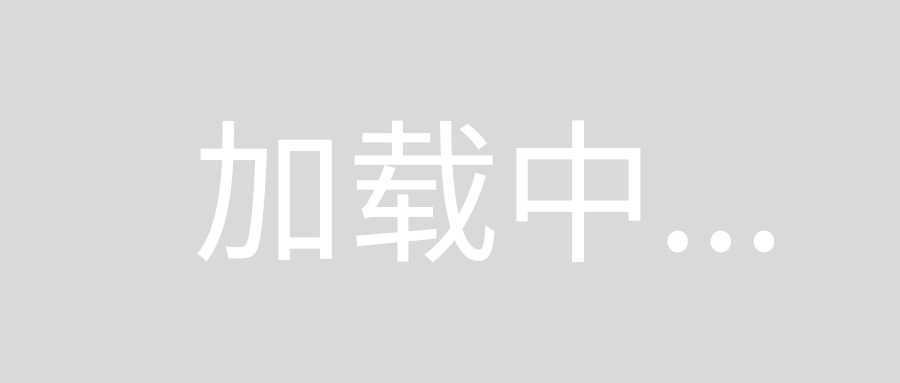