I'm new to Swift/iOS development.
I have a UITableView
in my storyboard
that I want to populate with some data. In my attempt to do so, I created a class that inherits from UITableViewController
. The implementation is not yet complete, but my understanding is that by inheriting from this class I can provide an IBOutlet
to both dataSource
and delegate
.
When I try to drag the outlet into the source file, I don't get the insertion point that I got when I tested this before. See image below:
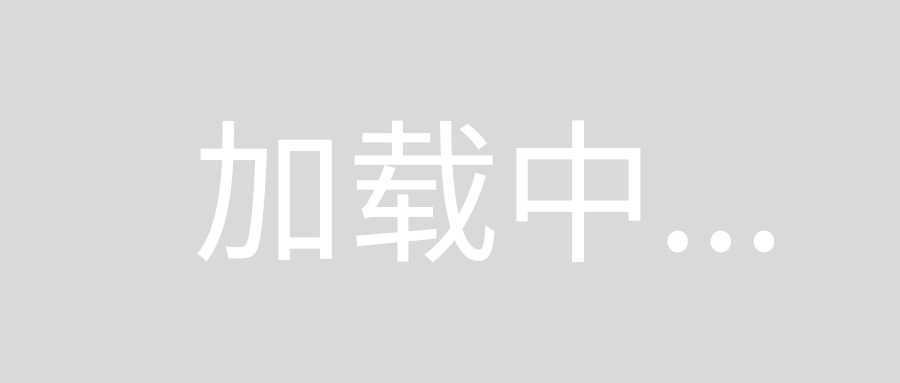
What do I have to do to define this class as the handler for the UITableView
?
In your storyboard, select the UITableView
and change this to the name of your UITableViewController
subclass. You then do not need to do any linking stuff.
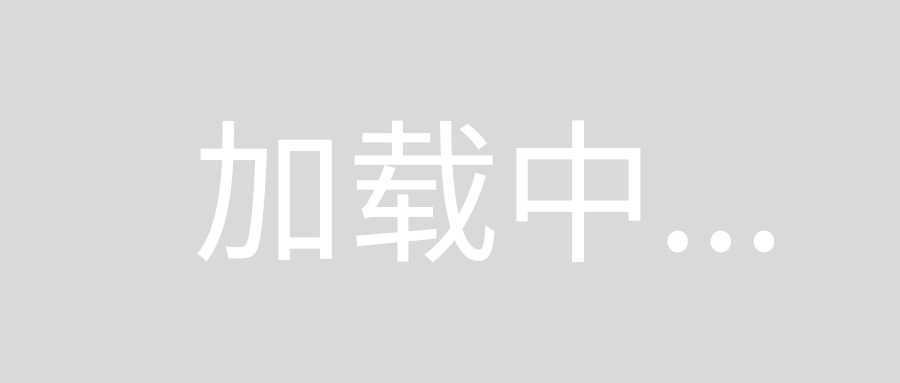
Set your viewController to inherit from UIViewController
, not from UITableViewController
(It seems like your IB is set up like that).
Do not forget to set your class to ZeroconfTableController
on the interface builder.
Than, you will be able to set the delegate
and datasource
. NOTE: the delegate
and the datasource
will not create IBOutlet
s.
Assign the delegate
and the dataSource
the following way:
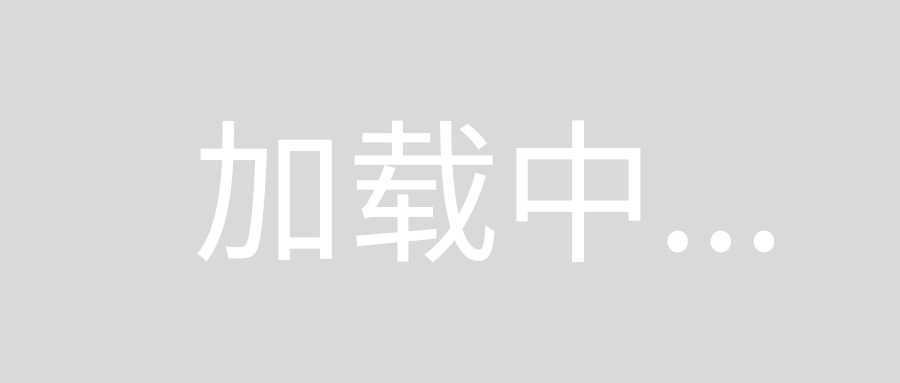
Also make sure, your viewController conforms to the protocols.
class ZeroconfTableController: UIViewController, UITableViewDataSource, UITableViewDelegate {
If you're using your own UITableView
, inherit from UIViewController
, not UITableViewController
.
If however, you want to use a UITableViewController
(which personally I don't use) in your storyboard, then do inherit from UITableViewController
. In this case you won't have to wire up the UITableViewDataSource
nor the UITableViewDelegate
.