Is there a way to make the tab bar transparent? I tried the following but it just showed a white background. Do I need to implement my own tabBarComponent? If so, is there any documentation on that class and what interface I need to implement?
const MainTabNavigator = TabNavigator(
{
MessageCenter: { screen: MessageCenterStack },
Camera: { screen: CameraStack },
},
{
tabBarPosition: 'bottom',
swipeEnabled: true,
animationEnabled: true,
tabBarOptions: {
style: {
backgroundColor: 'transparent',
},
}
}
);
I have to set position absolute
and give it a left right and bottom for it the backgroundColor transparent to take effect.
tabBarOptions: {
showIcon: true,
showLabel: false,
lazyLoad: true,
style: {
backgroundColor: 'transparent',
borderTopWidth: 0,
position: 'absolute',
left: 50,
right: 50,
bottom: 20,
height: 100
}
}
I finally got it working on android and ios by adding a container view for the custom tab bar component and make the container absolute positioned and leave the tab bar as it is
Here is the custom tab bar component
const TabBarComponent = (props) => (<BottomTabBar {...props} />)
Here is the tab bar options
{
tabBarComponent: props => {
return (
<View style={{
position: 'absolute',
left: 0,
right: 0,
bottom: 0,
}}>
<TabBarComponent {...props} />
</View>
)
},
tabBarOptions: {
style: {
borderTopWidth: 0,
backgroundColor: '#FFFFFF',
borderTopRightRadius: 20,
borderTopLeftRadius: 20,
height: 55,
paddingBottom: 5,
}
},
initialRouteName: 'HomeScreen',
}
And the final result
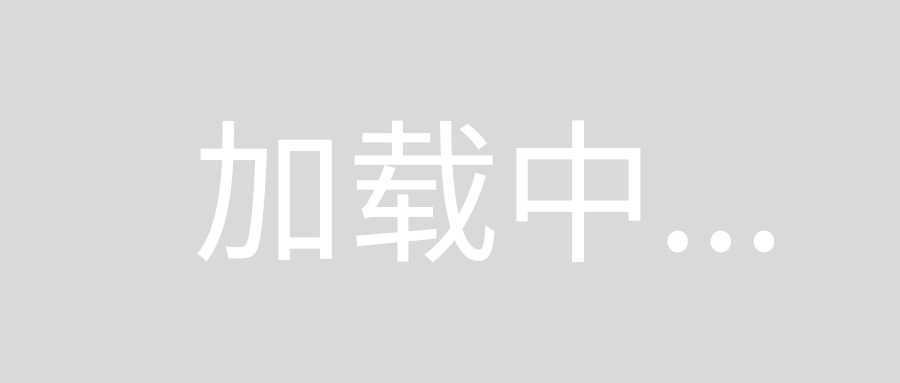
A lot of the answers here seem to be a little convoluted for the question. So for others looking how to do so, here's a simple answer:
Within the tab bar options change position to absolute and background colour to transparent such that it looks like:
tabBarOptions: {
style: {
backgroundColor: 'transparent',
position: 'absolute',
left: 0,
bottom: 0,
right: 0
}
}
Mohammed Tawfik's answer worked for me but I had to import <BottomTabBar>
component from react-navigation-tabs
instead of suggested react-navigation
.