I'm starting to investigate how to work with Facebook programmatically.
I've set URL scheme in my app, so that I could open it from browser using "myappopenup://".
Then I created an app in Facebook. Copied an AppId and tried to make like this:
let url = NSURL(string: "https://www.facebook.com/dialog/oauth?client_id={MY_APP_ID_WAS_HERE}&redirect_uri=myappopenup://fbcallback")
UIApplication.sharedApplication().openURL(url!)
After running this code, simulator opened safari (I've tried with SFSafariViewController first). but everything I saw was:
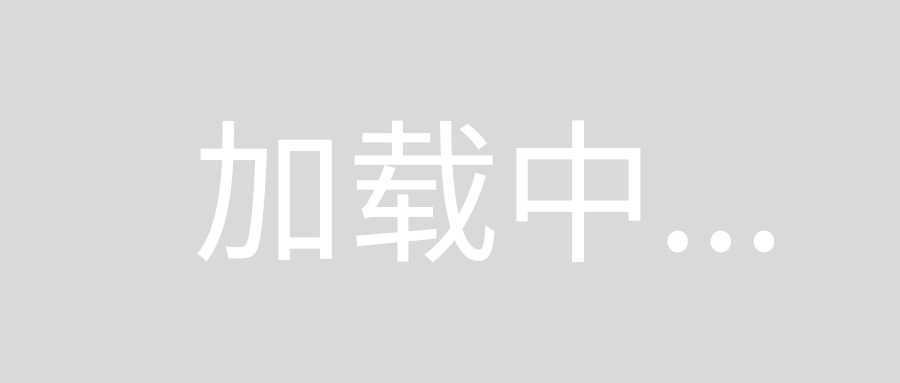
So, I'd like to know: Is it possible to redirect from the Facebook oauth to my app?
Yes it is possible. Here's the necessary redirect_uri:
https://graph.facebook.com/oauth/authorize
?response_type=token
&client_id='+'1247676148624015
&redirect_uri=fb1247676148624015://authorize
Make sure your facebook app is set up:
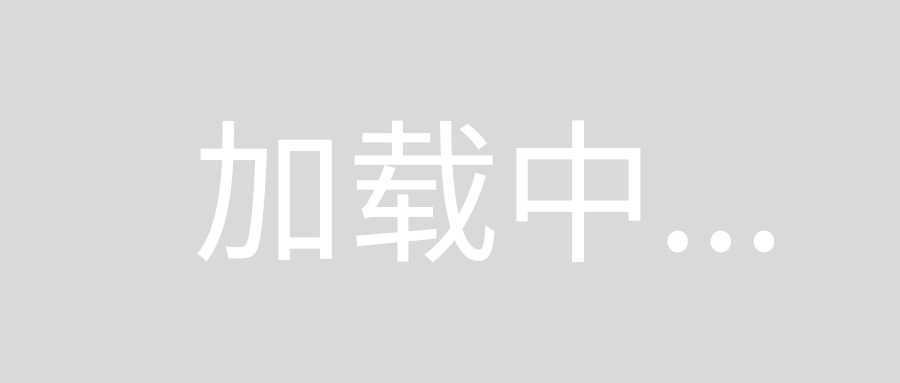
And make sure your custom URL scheme is set up:
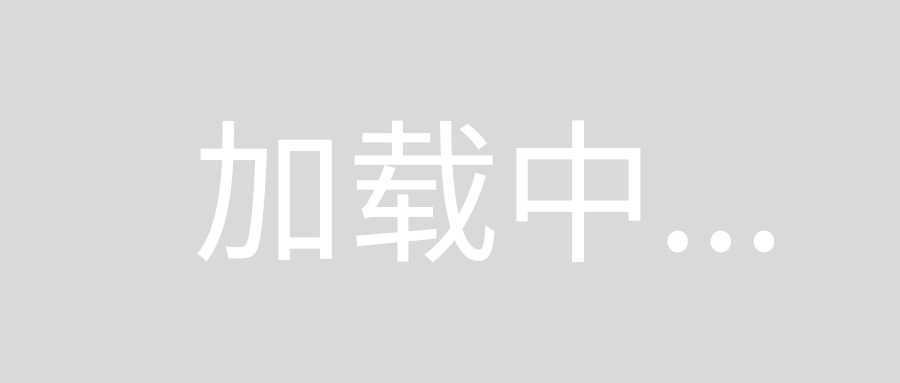
Disappointing comment above -- likely misled many people. This stuff is not well-documented at all, though.
I contacted Facebook support and they confirmed that it's not supported:
Thanks for getting in touch. We'll make the messaging more clear on this, but we do not support custom URI schemes as valid redirect URIs. To perform login in a native app without opening the browser, please use the official SDKs (is https://github.com/xamarin/FacebookComponents what you're looking for?).
They responded in less that 12 hours, which at least was impressive.
I managed to make Alex Paterson solution work here. I had to add the redirect_uri URL on Facebook Login settings too. On section Valid OAuth redirect URIs I've added the callback URL like this:
https://graph.facebook.com/oauth/authorize?
response_type=token&
client_id=12345678910&
redirect_uri=fb12345678910://authorize
As some of the other answers & comments indicate, I don't think that what you're trying to do is supported by Facebook - my impression is that they really want you to use the FBSDK to accomplish authenticating and receiving a token from facebook.
It feels like I've tried every possible configuration under the sun with the someapp://authorize
strategy, but it really seems likes they only want you to do this for web apps.
I'm building with react native (so this may not directly apply. I'm sure the obj-C is somewhat similar in practice), so I'm using the react-native-fbsdk
library which exposes LoginButton
and AccessToken
components, and you can use them as such:
<LoginButton
readPermissions={["email", "public_profile"]}
onLoginFinished={
(error, result) => {
if (error) {
alert("Login failed with error: " + result.error);
} else if (result.isCancelled) {
alert("Login was cancelled");
} else {
AccessToken.getCurrentAccessToken().then((data) => {
const token = data.accessToken;
// send the token to your backend
}
)
alert("Login was successful with permissions: " + result.grantedPermissions)
}
}
}
onLogoutFinished={() => alert("User logged out")}
/>