可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
Context
I have started a personal project in java with Gradle
as the build system and I want to use Dagger 2 as a DI. The main reason of doing that is to get used to that library and be able to use it easily in bigger projects.
What have I tried
I've managed to make the Google sample runs on IntelliJ IDEA
Problem
IntelliJ IDEA keeps telling me that it cannot resolve the generated class (in this case DaggerCoffeeApp_Coffee
). It's a bit annoying not to know if the written code is correct (specially when you are learning to use Dagger 2).
All java classes are the same as the Google sample. Here is my build.gradle
file:
apply plugin: 'java'
repositories {
mavenCentral()
}
dependencies {
testCompile group: 'junit', name: 'junit', version: '4.12'
compile 'com.google.dagger:dagger:2.0.1'
compile 'com.google.dagger:dagger-compiler:2.0.1'
}
Question
Is there any way to make IntelliJ IDEA recognize DaggerCoffeeApp_Coffee
as a generated class (and so make it possible to go to its implementation by `ctrl + left click)?
回答1:
Finally I made it!
I had to add the apt
and the idea
plugin so right now my build.gradle
file look like this:
buildscript {
repositories {
maven {
url "https://plugins.gradle.org/m2/"
}
}
dependencies {
classpath "net.ltgt.gradle:gradle-apt-plugin:0.4"
}
}
apply plugin: "net.ltgt.apt"
apply plugin: 'java'
apply plugin: 'idea'
repositories {
mavenCentral()
}
dependencies {
testCompile group: 'junit', name: 'junit', version: '4.12'
compile 'com.google.dagger:dagger:2.0.1'
apt 'com.google.dagger:dagger-compiler:2.0.1'
}
回答2:
Simplest way I found:
Add idea
plugin and add Dagger2 dependency like below:
plugins {
id "net.ltgt.apt" version "0.10"
}
apply plugin: 'java'
apply plugin: 'idea'
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
dependencies {
testCompile group: 'junit', name: 'junit', version: '4.12'
compile 'com.google.dagger:dagger:2.11'
apt 'com.google.dagger:dagger-compiler:2.11'
}
Turn on Annotation Processing
for IntelliJ: Go to Settings
and search for Annotation Processors
, check Enable annotation processing like below image:
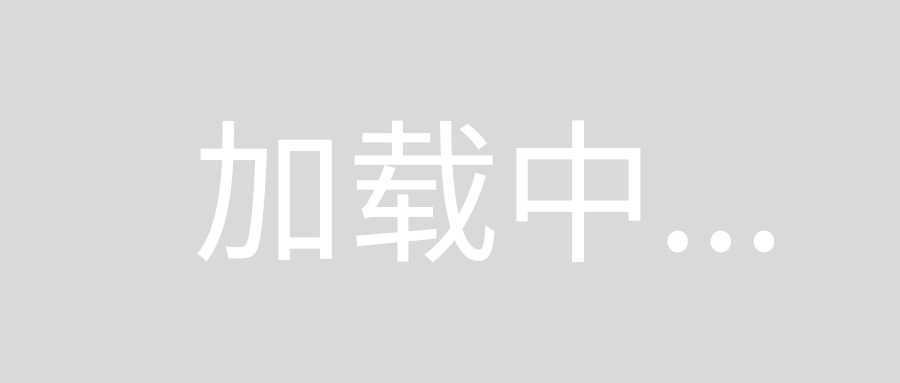
回答3:
you must manually enable the annotation processing in IntelliJ.
From: Settings --> Build, Execution, Deployment --> Compiler --> Annotation Processors --> Enable annotation processing and Obtain processors from project classpath
then rebuild the project and you will find the generated classes in the project.
Please note that I have used this solution in a (java) android project.
回答4:
I'm using version 2017.3.3
of IntelliJ IDEA, version 0.14
of the net.ltgt.apt
plugin and version 2.14.1
of Dagger and as well as applying the idea
plugin in the build.gradle
file (as in Pelocho's answer) I found I also had to tell IntelliJ where it can find the sources generated by Dagger, as follows:
apply plugin: 'idea'
idea {
module {
sourceDirs += file("$buildDir/generated/source/apt/main")
testSourceDirs += file("$buildDir/generated/source/apt/test")
}
}
回答5:
Here's the solution that worked for me:
File -> Project Structure -> (select your project under list of modules) -> Open 'Dependencies' tab
Then, click on green '+' sign, select 'JARs or directory' and select 'build/classes/main' folder.
Another solution would be to link folder with build class files using 'dependencies' block inside build.gradle:
https://stackoverflow.com/a/22769015/5761849
回答6:
This is what I had to do in order to get Idea to work with Dagger2 and gradle.
- Turn on annotation processing as shown in the answers above.
Add the following to the build.gradle
file in order for Idea to see the generated classes as sources.
sourceDirs += file("$projectDir/out/production/classes/generated/")
Here's the full listing of my build.gradle
plugins {
id 'java'
id 'idea'
id "net.ltgt.apt" version "0.10"
}
idea {
module {
sourceDirs += file("$projectDir/out/production/classes/generated/")
}
}
repositories {
mavenCentral()
}
dependencies {
compile 'com.google.dagger:dagger:2.16'
apt 'com.google.dagger:dagger-compiler:2.16'
}
sourceCompatibility = 1.8
Also, I had to add the following gradle task (to my build.gradle file) to clear out my out
directory. When I moved some files around and Dagger2 regenerated the source files, the out
directory wasn't being cleared out :(. I also included this task in my run configuration, so that it gets triggered before I rebuild my project.
task clearOutFolder(type: Delete) {
delete 'out'
}