I'm about to create a solution that will list events that will occur in specific days, and was looking for a Calendar control that I can deal with to make only the days with events clickable, and of course receive this click event and handle the rest myself in my Controller.
(something like the old asp:Calendar server-side control in Webforms).
is there any that match this scenario?
Update: What I'm exactly looking for is a Mini Calendar, not a full Calendar like the one in Outlook.
this is what I'm exactly looking for:
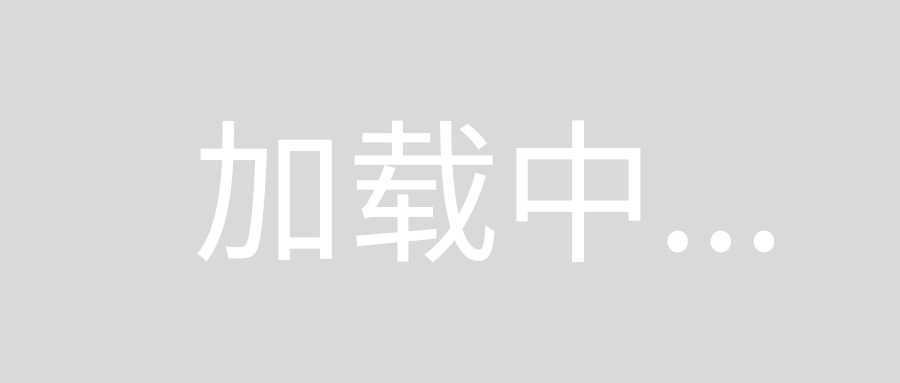
jQuery UI can do the exact styling you want.. (and functionality)
You will need to download the jQuery UI script and the Smoothness theme (visit http://jqueryui.com/download/ and select core and datepicker from the radio buttons, and smoothness from the drop-down to the right)
html
<div id="datepicker"></div>
javascript
var activeDays=[1,4,10,21,22,23,27,28,30];
$('#datepicker').datepicker(
{
beforeShowDay: function(date) {
var hilight = [false,''];
if ( activeDays.indexOf( date.getDate() ) > -1)
{
hilight = [true,'isActive'];
}
return hilight;
}
}
);
The activeDays
variable could hold dates directly (instead of just the day number) and you would need to alter the conditional inside the function to check for a match.
The isActive
is a class used to control the styling of the active dates..
.isActive a.ui-state-default{
background:none;
background-color:pink;
}
Live example at http://jsfiddle.net/gaby/C95nx/2/
the above code and example renders as
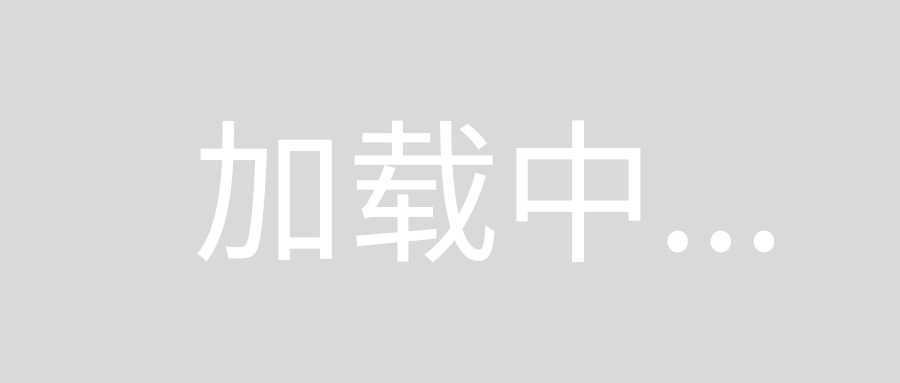
I made something like this a couple of years ago by heavily modifying a javascript calendar called MooTools Event Calendar
There are several other javascript calendars that look pretty good as well.
These 2 use JQuery:
- Full Calendar
- Web-Delicious Events Calendar and Planner
You can use jquery UI datepicker along with the beforeShowDay
event to customize which days are selectable and which days are not. Here is the minimal code which disables selecting the date 5 and you can extend the logic.
$(function() {
$( "#datepicker" ).datepicker({
beforeShowDay: function(date) {
if(date.getDate() == 5){
return[false, ''];
}
else{
return[true, ''];
}
}
});
});
Here's a quick demo
Many people use the jQuery UI datepicker. I'm not sure of a built in asp.net mvc control for the calendar.
If you can still use Javascript in your code, take this control and customize its UI. It has source code available for your own customization requirements.
http://www.javascriptkit.com/script/script2/eventscalendar.shtml#
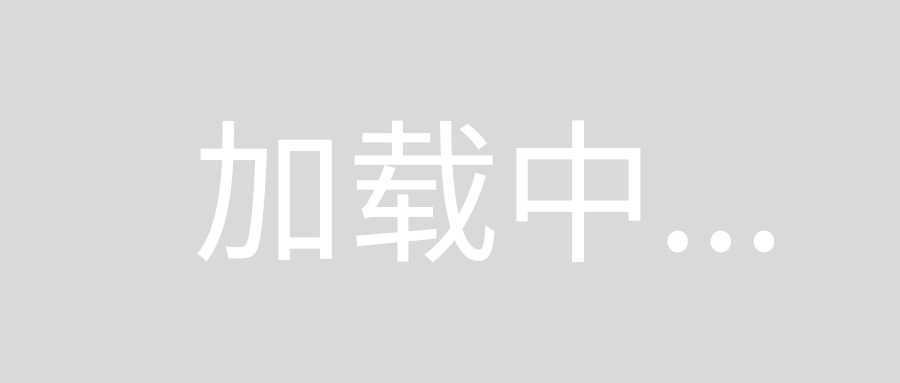
I realise this is beyond late as an answer, but your question is quite high up in Google results, so hopefully it will help other people. I wrote an article on this very topic here:
Datepicker based MVC3 Events Calendar
How to limit days available in jquery ui date-picker
or
http://test.thecodecentral.com/cms/jqueryui/datepicker/
You can integrate MVC with silverlight and use silverlight calendar
http://developerstyle.posterous.com/silverlight-calendar-with-mvc-3
In order to complement @gaby-aka-g-petrioli's answer I included onChangeMonthYear
and onSelect
methods that allows get click actions.
$("#my_datepicker_calendar").datepicker({
dateFormat : 'm/d/yy', // month/day/year
changeMonth : true,
changeYear : true,
beforeShowDay : function(date)
{
var mm = date.getMonth() + 1, dd = date.getDate(), yy = date.getFullYear();
var dt = mm + "/" + dd + "/" + yy;
if ( typeof selectedDates[dt] != 'undefined' ) {
return [true, "", selectedDates[dt].content];
}
return [false, ""];
},
onChangeMonthYear : function(year, month)
{
alert('Month selected is ' + month + ' and year is ' + year);
},
onSelect : function(date)
{
var date = date.split("/");
alert('Date selected is ' + date[0] + '/' + date[1] + '/' + date[2] + '.');
}
});