I'm using Firebase and I'm trying to add a username to the database with the email and password.
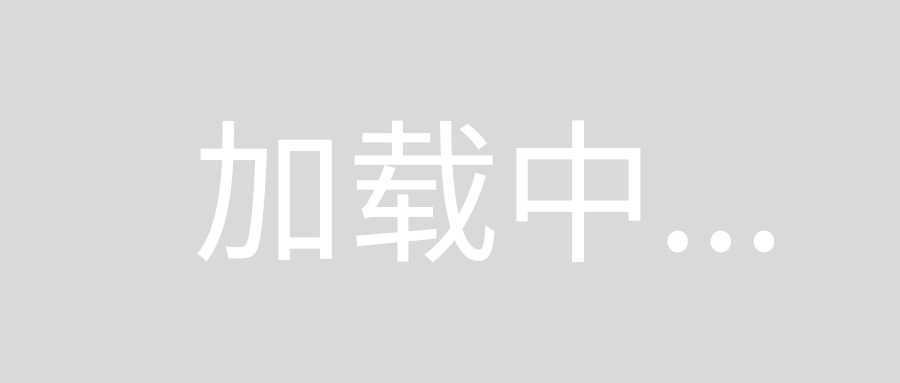
Is there a way to do it or is createUserWithEmailAndPassword()
function only for email and password?
signUp.addEventListener("click", function(user)
{
var username = usernameTxt.value;
var email = emailTxt.value;
var password = passwordTxt.value;
firebase.auth().createUserWithEmailAndPassword(email, password).catch(function(error)
{
// Handle Errors here.
var errorCode = error.code;
var errorMessage = error.message;
if (errorCode == 'auth/email-already-in-use')
{
alert('email-already-in-use.');
}
else
{
alert(errorMessage);
}
console.log(error);
});
});
The solution that i found to how to add username with the createUserWithEmailAndPassword() function:
firebase.auth().onAuthStateChanged(function(user) {
var username = usernameTxt.value;
if (user) {
firebaseDataBase.ref('users/' + user.uid).set({
email: user.email,
uid : user.uid,
username: username
});
console.log("User is signed in.");
} else {
console.log("No user is signed in.");
}
});
This cannot be done through createUserWithEmailAndPassword()
but there is a firebase method for this . You will need to listen for when authentication state is changed , get the user , then update the profile info . See Below
This code would come after createUserWithEmailAndPassword()
firebase.auth().onAuthStateChanged(function(user) {
if (user) {
// Updates the user attributes:
user.updateProfile({ // <-- Update Method here
displayName: "NEW USER NAME",
photoURL: "https://example.com/jane-q-user/profile.jpg"
}).then(function() {
// Profile updated successfully!
// "NEW USER NAME"
var displayName = user.displayName;
// "https://example.com/jane-q-user/profile.jpg"
var photoURL = user.photoURL;
}, function(error) {
// An error happened.
});
}
});
As stated in firebase User Api here : https://firebase.google.com/docs/reference/js/firebase.User#updateProfile
Hope this helps
You need to create that database users
child node yourself ;-)
The createUserWithEmailAndPassword
function only creates a new user in Firebase authentication service. The database itself isn't changed at all as a result.
To add this new user to the database as well, try:
firebase.database().ref("users").child(user.uid).set(...)
You can create a users
endpoint and store custom user data in there.
function writeUserData(userId, name, email, imageUrl) {
firebase.database().ref('users/' + userId).set({
username: name,
email: email,
profile_picture : imageUrl,
// Add more stuff here
});
}
Have a look to https://firebase.google.com/docs/database/web/read-and-write