I am a happy django developer and now want to build a small python desktop app. I have decided to use wxpython as my gui toolkit.
Now starts the confusion. How should I organize my code? are there any simple starting point schemes? Any pointers to real world code of small wxpython application with database interactions ?
This is my favorite way to get started with a new wxPython project: http://www.oneminutepython.com/
It also starts laying out the code for you in a nice way.
I stick to the "what I write, that I get" rule. So I usually start with one of those:
1) Using default Frame sizer for panel:
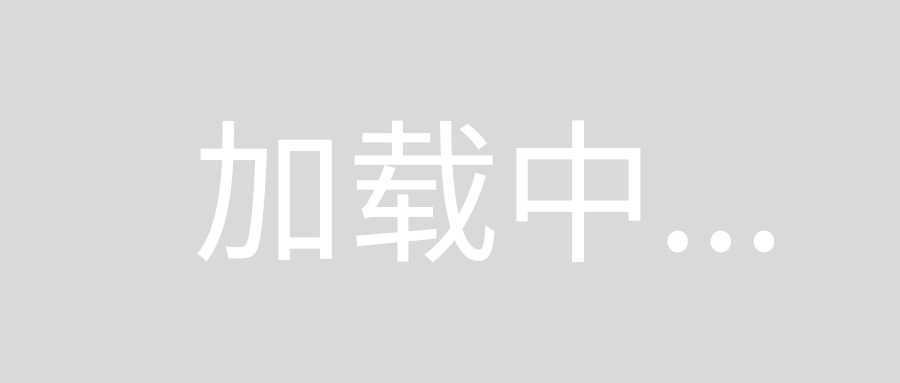
import wx
class MainWindow(wx.Frame):
def __init__(self, *args, **kwargs):
wx.Frame.__init__(self, *args, **kwargs)
self.panel = wx.Panel(self)
self.button = wx.Button(self.panel, label="Test")
self.sizer = wx.BoxSizer()
self.sizer.Add(self.button)
self.panel.SetSizerAndFit(self.sizer)
self.Show()
app = wx.App(False)
win = MainWindow(None)
app.MainLoop()
2) Using default Frame sizer for panel and Border for everything inside:
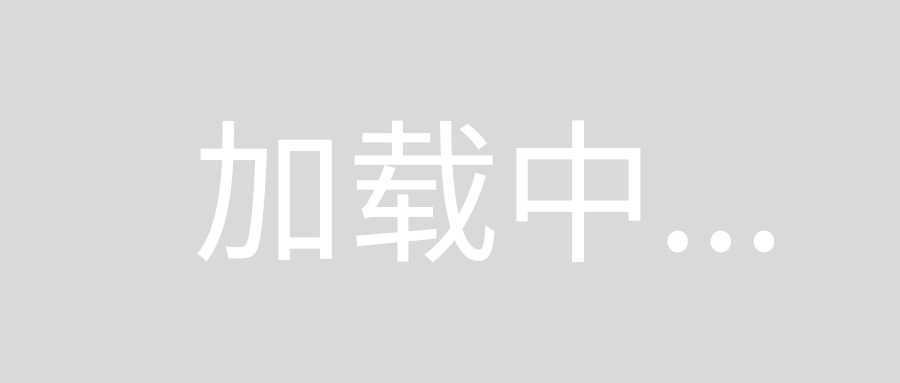
import wx
class MainWindow(wx.Frame):
def __init__(self, *args, **kwargs):
wx.Frame.__init__(self, *args, **kwargs)
self.panel = wx.Panel(self)
self.button = wx.Button(self.panel, label="Test")
self.sizer = wx.BoxSizer()
self.sizer.Add(self.button)
self.border = wx.BoxSizer()
self.border.Add(self.sizer, 1, wx.ALL | wx.EXPAND, 5)
self.panel.SetSizerAndFit(self.border)
self.Show()
app = wx.App(False)
win = MainWindow(None)
app.MainLoop()
3) Using custom Frame sizer for panel, so I can control it, for example call "Fit" and "Layout" on it when needed:
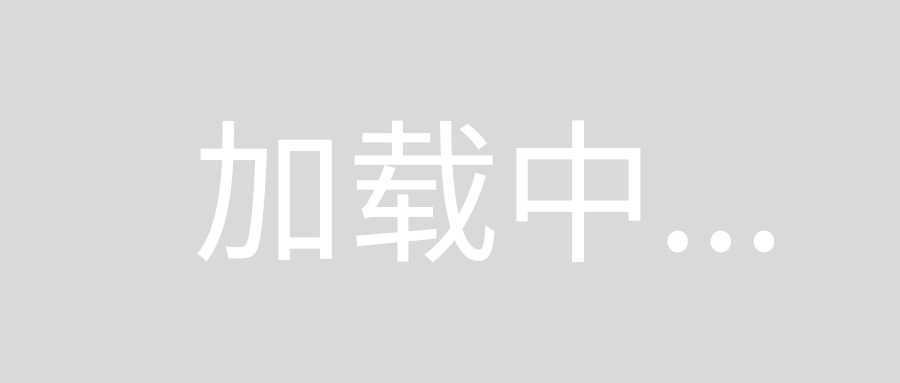
import wx
class MainWindow(wx.Frame):
def __init__(self, *args, **kwargs):
wx.Frame.__init__(self, *args, **kwargs)
self.panel = wx.Panel(self)
self.button = wx.Button(self.panel, label="Test")
self.windowSizer = wx.BoxSizer()
self.windowSizer.Add(self.panel, 1, wx.ALL | wx.EXPAND)
self.sizer = wx.BoxSizer()
self.sizer.Add(self.button)
self.border = wx.BoxSizer()
self.border.Add(self.sizer, 1, wx.ALL | wx.EXPAND, 5)
self.panel.SetSizerAndFit(self.border)
self.SetSizerAndFit(self.windowSizer)
self.Show()
app = wx.App(False)
win1 = MainWindow(None)
app.MainLoop()
That is my starting point. Then I just add other primitive widgets and bind events for them. If I need a new panel, I usually put it in a new module and derive the Panel class. I do the same for special widgets which have to derive from the primitive ones - like drawing panels, OpenGL canvas, special case buttons etc.
It is usually also good idea to have functionality separated from GUI. So I write functionality first the way it does not need GUI.