This question already has answers here:
Closed 2 years ago.
I have created a shadow using QuartzCore
for my UITextView
with this following code.
myTextView.layer.masksToBounds = NO;
myTextView.layer.shadowColor = [UIColor blackColor].CGColor;
myTextView.layer.shadowOpacity = 0.7f;
myTextView.layer.shadowOffset = CGSizeMake(2.0f, 2.0f);
myTextView.layer.shadowRadius = 8.0f;
myTextView.layer.shouldRasterize = YES;
It creates a shadow
and looks good too.
Here it is my output for the above code.
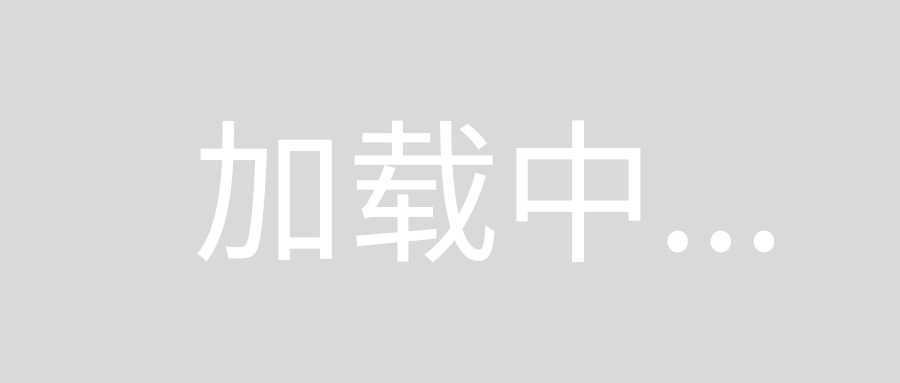
But When I try to add a text to the myTextView
, my textView text goes out of the bounds and it looks outside of the myTextView
like below.
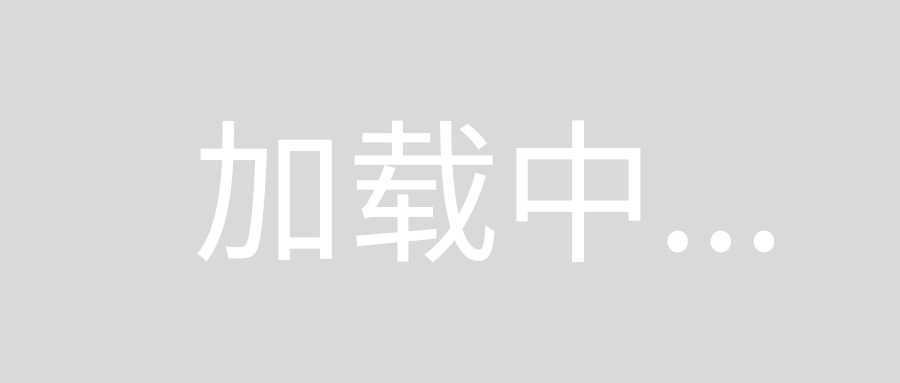
It's happening only when I add shadow. The text inside the textView
is not showing weird If I don't add shadow.What I am doing wrong?? How could I overcome this? Why it is happening?
UPDATE:
@borrrden
said
I found It is happening, because of setting the maskToBounds = NO;
If we set YES
then we cannot get shadow. Reason Here it is an answer
There is no "right" solution, because of UIView behavior. When masksToBounds is NO, any sublayers that extend outside the layer's boundaries will be visible. And UITextField scroll text outside the UITextField layer.
Add clear view behind the UITextView and drop a shadow on it.
Just add another UIView under your textView and set it's layer to display shadow (do not forget to set it's background color to something other than clear color - or shadow wont be drawn)
myTextView = [UITextView alloc] initWithFrame:CGRectMake(100,100,200,200);
UIView* shadowView = [UIView alloc] initWithFrame:myTextView.frame];
shadowView.backgroundColor = myTextView.backgroundColor;
shadowView.layer.masksToBounds = NO;
shadowView.layer.shadowColor = [UIColor blackColor].CGColor;
shadowView.layer.shadowOpacity = 0.7f;
shadowView.layer.shadowOffset = CGSizeMake(2.0f, 2.0f);
shadowView.layer.shadowRadius = 8.0f;
shadowView.layer.shouldRasterize = YES;
[someView addSubview:shadowView];
[someView addSubView:myTextView];
In addition to the previous answer, just copy all attributes from the original uiTextView to the helper view:
UITextView *helperTextView = [[UITextView alloc] init];
helperTextView = textView; //copy all attributes to the helperTextView;
shadowTextView = [[UIView alloc] initWithFrame:textView.frame];
shadowTextView.backgroundColor = textView.backgroundColor;
shadowTextView.layer.opacity = 1.0f;
shadowTextView.layer.masksToBounds = NO;
shadowTextView.layer.shadowColor = [UIColorFromRGB(0x00abff)CGColor];
shadowTextView.layer.shadowOpacity = 1.0f;
shadowTextView.layer.shadowOffset = CGSizeMake(1.0f, 1.0f);
shadowTextView.layer.shadowRadius = 10.0f;
shadowTextView.layer.cornerRadius = 8.0f;
shadowTextView.layer.shouldRasterize = YES;
[self.view addSubview:shadowTextView];
[self.view addSubview:helperTextView];