I used the code in this link to map textures of human faces. This code uses GLKIT to render the images. Code works fine in simulator but the same code if I run in device its not working. The below are the screen shots of the images where it works in device and not in my ipad.
Code I used to Load Texture:
- (void)loadTexture:(UIImage *)textureImage
{
glGenTextures(1, &_texture);
glBindTexture(GL_TEXTURE_2D, _texture);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR);
CGImageRef cgImage = textureImage.CGImage;
float imageWidth = CGImageGetWidth(cgImage);
float imageHeight = CGImageGetHeight(cgImage);
CFDataRef data = CGDataProviderCopyData(CGImageGetDataProvider(cgImage));
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA, imageWidth, imageHeight, 0, GL_RGBA,
GL_UNSIGNED_BYTE, CFDataGetBytePtr(data));
}
Image of simulator:
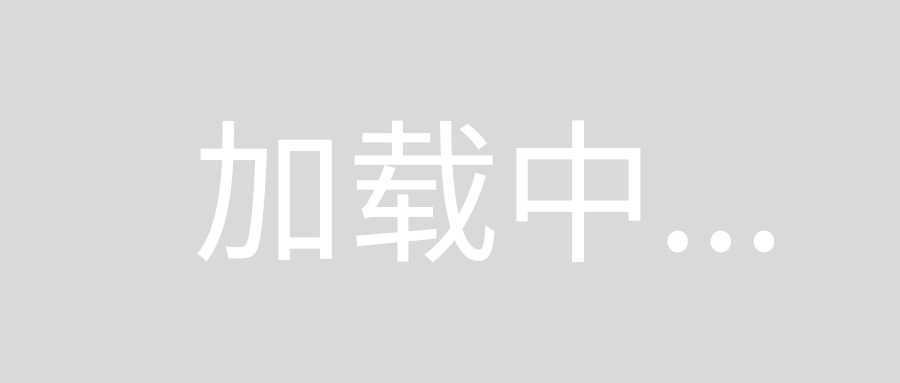
The same code in device gives the following output:
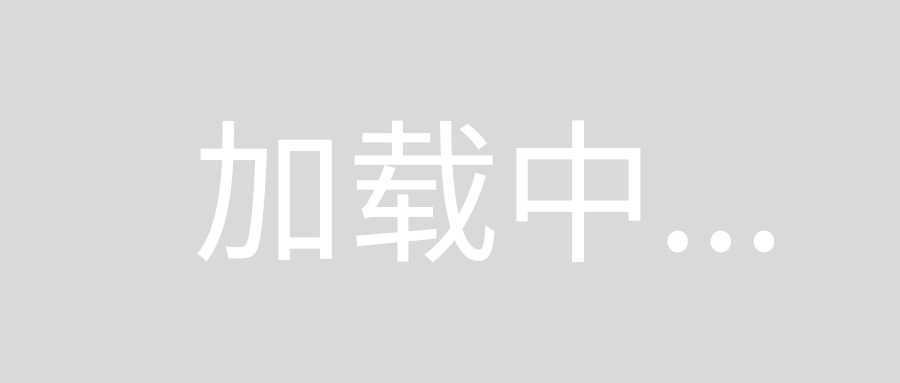
Is There something i`m missing?
I finally caved in and switched to GLKTextureLoader. As Adam mentions, it's pretty sturdy.
Here's an implementation which might work for you:
- (void)loadTexture:(NSString *)fileName
{
NSDictionary* options = [NSDictionary dictionaryWithObjectsAndKeys:
[NSNumber numberWithBool:YES],
GLKTextureLoaderOriginBottomLeft,
nil];
NSError* error;
NSString* path = [[NSBundle mainBundle] pathForResource:fileName ofType:nil];
GLKTextureInfo* texture = [GLKTextureLoader textureWithContentsOfFile:path options:options error:&error];
if(texture == nil)
{
NSLog(@"Error loading file: %@", [error localizedDescription]);
}
glBindTexture(GL_TEXTURE_2D, texture.name);
}
I'll change it for the mtl2opengl
project on GitHub soon...
Looking at the screenshots ... I wonder if the problem is that you're going from iPhone to iPad?
i.e. retina to non-retina?
Looks to me like your image loading might be ignoring the Retina scale, so that the texture map is "2x too big" on the iPad.
Using Apple's method (my other answer) should fix that automatically, but if not: you could try making two copies of the image, one at current size (named "image@2x.png") and one at half size (resize in photoshop / preview.app / etc) (named "image.png")