I am setting a background
image to LinearLayout
like this:
1.back_xml:
<?xml version="1.0" encoding="UTF-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/back" >
</item>
<item>
<shape>
<solid/>
<stroke android:width="1dip" android:color="#225786" />
<corners android:radius="10dip"/>
<padding android:left="0dip" android:top="0dip" android:right="0dip" android:bottom="0dip" />
</shape>
</item>
2. tile.xml
<bitmap xmlns:android="http://schemas.android.com/apk/res/android"
android:src="@drawable/pie_chart_background"
android:tileMode="repeat">
</bitmap>
now I am setting the back.xml as a background
to LinearLayout
it works fine.
I need to have a image
with rounded corners and also a border to it.But i only have the border with rounded corners and not the image what is the problem in my code am i missing something?
this is how my image looks:
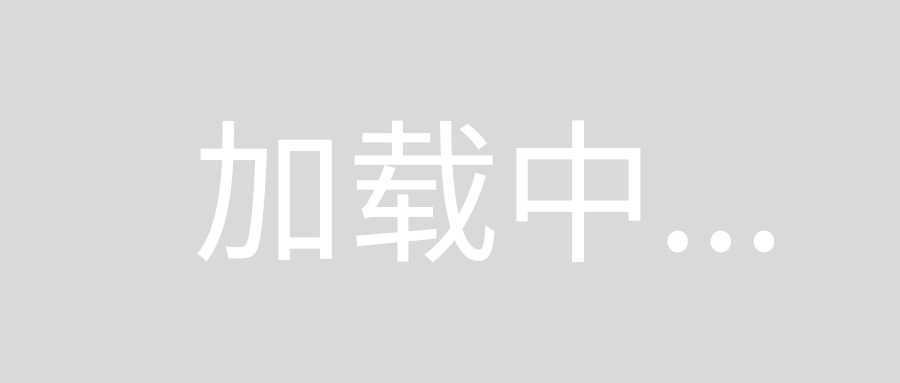
After spending hours of time behind your issue, finally I achieved, hope now it will give you same result as you want, please go through below code and let me know whether its works or not?
Pass appropriate parameter to below function to get rounded corner with your desire color border.
public static Bitmap getRoundedCornerImage(Bitmap bitmap, int cornerDips, int borderDips, Context context) {
Bitmap output = Bitmap.createBitmap(bitmap.getWidth(), bitmap.getHeight(),
Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(output);
final int borderSizePx = (int) TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_DIP, (float) borderDips,
context.getResources().getDisplayMetrics());
final int cornerSizePx = (int) TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_DIP, (float) cornerDips,
context.getResources().getDisplayMetrics());
final Paint paint = new Paint();
final Rect rect = new Rect(0, 0, bitmap.getWidth(), bitmap.getHeight());
final RectF rectF = new RectF(rect);
paint.setAntiAlias(true);
paint.setColor(0xFFFFFFFF);
paint.setStyle(Paint.Style.FILL);
canvas.drawARGB(0, 0, 0, 0);
canvas.drawRoundRect(rectF, cornerSizePx, cornerSizePx, paint);
paint.setXfermode(new PorterDuffXfermode(PorterDuff.Mode.SRC_IN));
canvas.drawBitmap(bitmap, rect, rect, paint);
paint.setColor((Color.RED)); // you can change color of your border here, to other color
paint.setStyle(Paint.Style.STROKE);
paint.setStrokeWidth((float) borderSizePx);
canvas.drawRoundRect(rectF, cornerSizePx, cornerSizePx, paint);
return output;
}
main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
tools:context=".MainActivity" >
<ImageView
android:id="@+id/image"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
/>
</RelativeLayout>
OnCreate
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
ImageView rl=(ImageView)findViewById(R.id.image);
Bitmap bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.testing); // your desire drawable image.
rl.setImageBitmap(getRoundedCornerImage(bitmap, 10, 10, this));
}
Original image
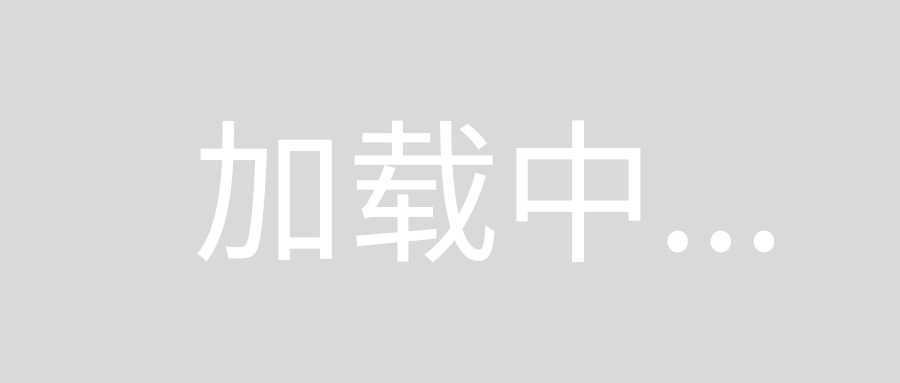
Output
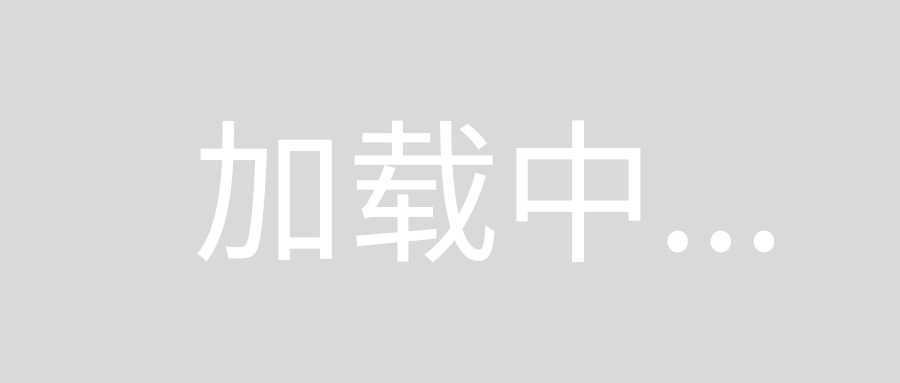
Below links help me to achieve my goal:
Border over a bitmap with rounded corners in Android
Creating ImageView with round corners
How to make an ImageView to have rounded corners
How to set paint.setColor(int color)
try your back.xml some thing like like this instead of giving a rectangle background image.
<?xml version="1.0" encoding="UTF-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="#ff00ff >
</item>
<item>
<shape android:shape="rectangle" >
<solid/>
<stroke android:width="1dip" android:color="#225786" />
<corners android:radius="10dip"/>
<padding android:left="0dip" android:top="0dip" android:right="0dip" android:bottom="0dip" />
</shape>
</item>
Another alternative to what Raj has posted is to use a background image with transparency at the corner.
The BEST solution, as your background is simple, is to use a 9-patch, which is the Android-native solution to the majority of background images: http://developer.android.com/guide/topics/graphics/2d-graphics.html#nine-patch, which is similar in style to how some website backgrounds are drawn.
Android also has it's own 9-patch creator bundled with the sdk: http://developer.android.com/tools/help/draw9patch.html, so it's easy enough to edit your own png files, then apply the 9-patch to the layout. The advantage of 9-patches is that they also handle the resizing the UI for you, meaning you won't encounter as many problems when using your app on different screens
Use your shape in combination with an image with rounded corners.
You can use AndroidQuery to load the image with rounded corners.
See http://code.google.com/p/android-query/wiki/ImageLoading#Rounded_Corner
String url ="http://www.vikispot.com/z/images/vikispot/android-w.png";
ImageOptions options = new ImageOptions();
options.round = 15;
aq.id(R.id.image).image(url, options);
I think you should have done it like this.
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item>
<shape>
<solid android:color="@color/back" />
<stroke android:width="1dip" android:color="#225786" />
<corners android:radius="10dip"/>
<padding android:left="0dip" android:top="0dip" android:right="0dip" android:bottom="0dip" />
</shape>
</item>
If the background is not a color but it is a bitmap, you should follow this beautiful guide
http://www.curious-creature.org/2012/12/11/android-recipe-1-image-with-rounded-corners/
Solution 1
create a 9-patch image for the border. the middle of the image should be transparent.
use a RelativeLayout as a parent and put your image LinearLayout at the bottom. set the border 9-patch as the background to another linear layout and place it on top of the image LinearLayout. Then it will cover the corners and you will get the expected result.
Solution 2
public static Bitmap getRoundedCornerBitmap(Bitmap bitmap) {
Bitmap output = Bitmap.createBitmap(bitmap.getWidth(),
bitmap.getHeight(), Config.ARGB_8888);
Canvas canvas = new Canvas(output);
final int color = 0xff424242;
final Paint paint = new Paint();
final Rect rect = new Rect(0, 0, bitmap.getWidth(), bitmap.getHeight());
final RectF rectF = new RectF(rect);
final float roundPx = 12;
paint.setAntiAlias(true);
canvas.drawARGB(0, 0, 0, 0);
paint.setColor(color);
canvas.drawRoundRect(rectF, roundPx, roundPx, paint);
paint.setXfermode(new PorterDuffXfermode(Mode.SRC_IN));
canvas.drawBitmap(bitmap, rect, rect, paint);
return output;
}