By default iOS is providing "Share appname" option in the shortcut options,when the app is downloaded from the app store.
Refer the image below:
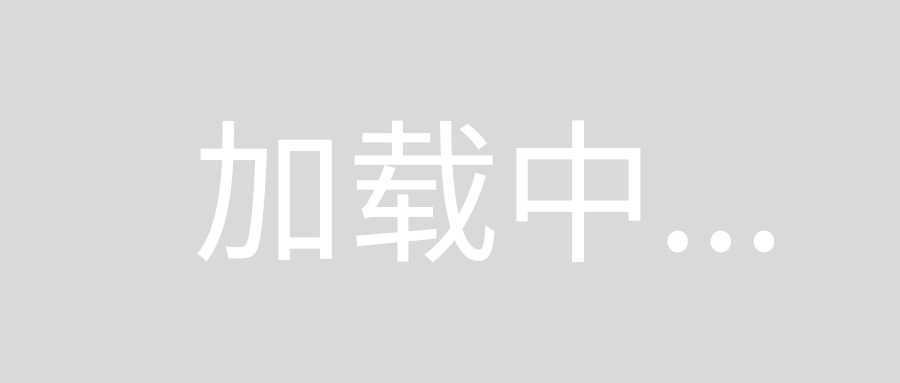
When clicked on it,it is opening the share intent in the phone menu screen itself,without redirecting to the app,where the user can share the app.
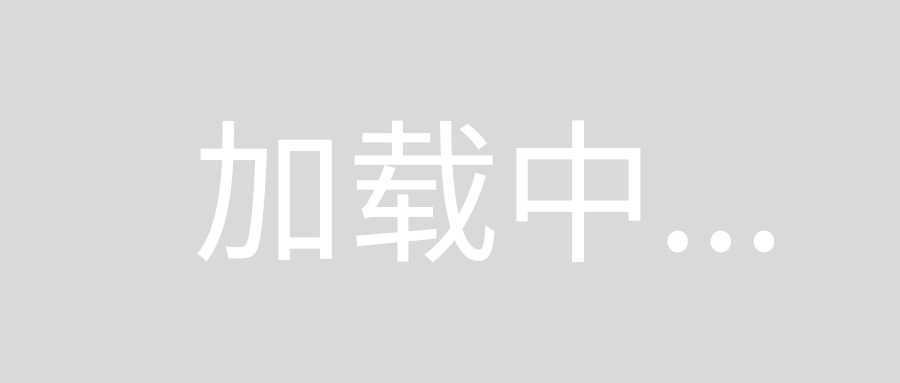
I want to implement this in android.
Here is what I have I tried so far:
<shortcut
android:shortcutId="share_app"
android:enabled="true"
android:icon="@drawable/ic_cart_active"
android:shortcutShortLabel="@string/shortcut_share"
android:shortcutLongLabel="@string/shortcut_share">
<intent
android:action="android.intent.action.send"
android:targetPackage="com.example.android.internal"
android:targetClass="" />
</shortcut>
But this is not working,since I am not able to understand, what should be the
targetClass over here.
EDIT: Nilesh`s answer almost worked,but the only problem that I am facing right now is,whenever I am clicking the share button from shortcut, the launcher activity is shown for a whisker of a second ,then the sharing intent chooser is showing up.Now when I am pressing the home button with the sharing options showing,the app is going to background.This should not happen.Any idea how to avoid this.
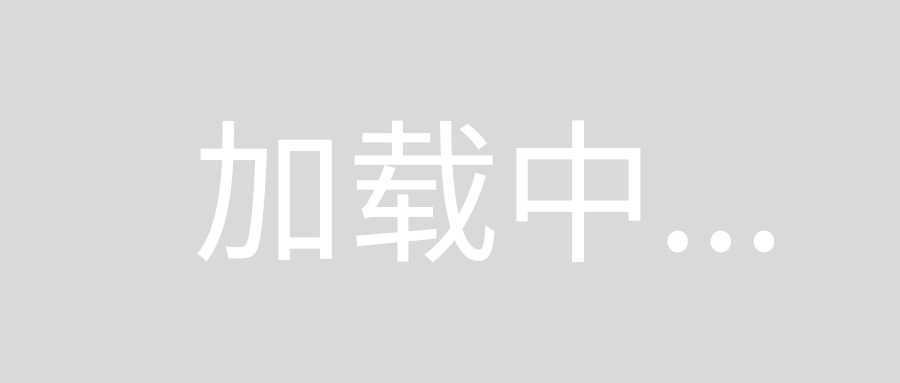
You can use App shortcuts
but the main disadavtange of this option is that it is available from Oreo and above version of android
Here is the sample code
Create a resource file: res/xml
directory (Screenshot)
<shortcuts xmlns:android="http://schemas.android.com/apk/res/android">
<shortcut
android:enabled="true"
android:icon="@drawable/ic_share"
android:shortcutDisabledMessage="@string/share_app"
android:shortcutId="nilu"
android:shortcutLongLabel="@string/share_app"
android:shortcutShortLabel="@string/share_app">
<intent
android:action="android.intent.action.VIEW"
android:targetClass="neel.com.demo.ShareActivity"
android:targetPackage="neel.com.demo" />
<categories android:name="android.shortcut.conversation" />
</shortcut>
</shortcuts>
Now you need to register your shortcut in your manifest file under activity tag
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="neel.com.demo">
<application
android:allowBackup="false"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity" />
<activity android:name=".ShareActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<meta-data
android:name="android.app.shortcuts"
android:resource="@xml/shortcuts" />
</activity>
</application>
</manifest>
Now create a simple share acivity to share your app link
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
public class ShareActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_home);
Intent sendIntent = new Intent();
sendIntent.setAction(Intent.ACTION_SEND);
sendIntent.putExtra(Intent.EXTRA_TEXT,
"Hey check out my app at: https://play.google.com/store/apps/details?id=neel.com.demo");
sendIntent.setType("text/plain");
startActivity(sendIntent);
finish();
}
}
OUTPUT
When user long press on app icon your shortcut will display like below image
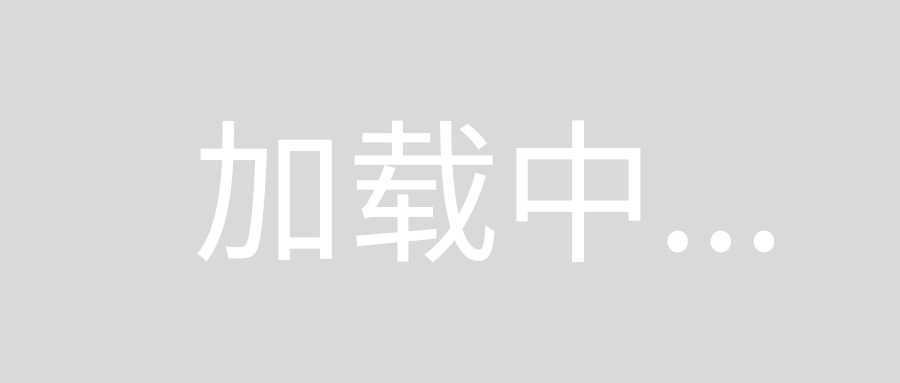
after click of shortcut
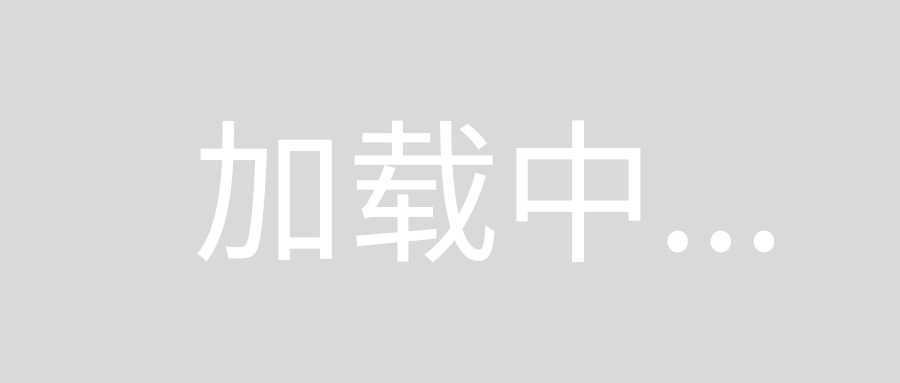
UPDATE use android:excludeFromRecents="true"
in your ShareActivity
<application
android:allowBackup="false"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity" />
<activity
android:name=".ShareActivity"
android:excludeFromRecents="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<meta-data
android:name="android.app.shortcuts"
android:resource="@xml/shortcuts" />
</activity>
</application>
there is two way to create shortcut.
one: create static shortcuts,In your app's manifest file (AndroidManifest.xml), find an activity whose intent filters are set to the android.intent.action.MAIN action and the android.intent.category.LAUNCHER category. and then add a element to this activity that references the resource file where the app's shortcuts are defined:
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.myapplication">
<application ... >
<activity android:name="Main">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<meta-data android:name="android.app.shortcuts"
android:resource="@xml/shortcuts" />
</activity>
...
two:Create dynamic shortcuts
you can use code to do it,The ShortcutManager API allows you to complete the following operations on dynamic shortcuts:
ShortcutManager shortcutManager = getSystemService(ShortcutManager.class);
ShortcutInfo shortcut = new ShortcutInfo.Builder(context, "id1")
.setShortLabel("Website")
.setLongLabel("Open the website")
.setIcon(Icon.createWithResource(context, R.drawable.icon_website))
.setIntent(new Intent(Intent.ACTION_VIEW,
Uri.parse("https://www.mysite.example.com/")))
.build();
shortcutManager.setDynamicShortcuts(Arrays.asList(shortcut));
for more information, you can through the official website how to create shortcut in android?