I want to move the label with respect to slider thumb just like this one:
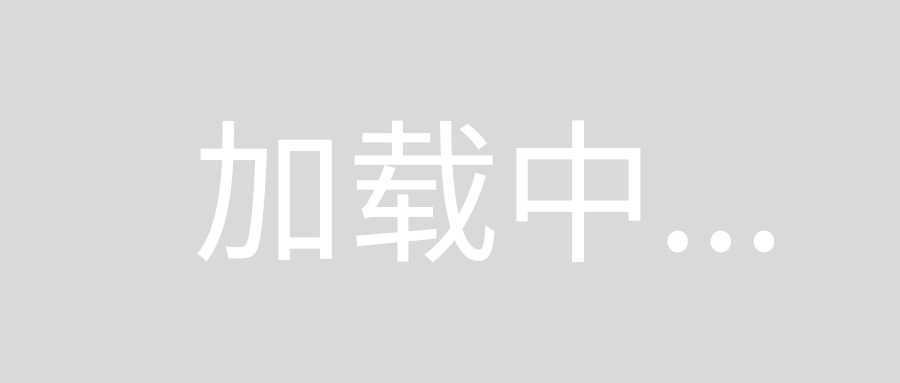
Right now my slider is like this:
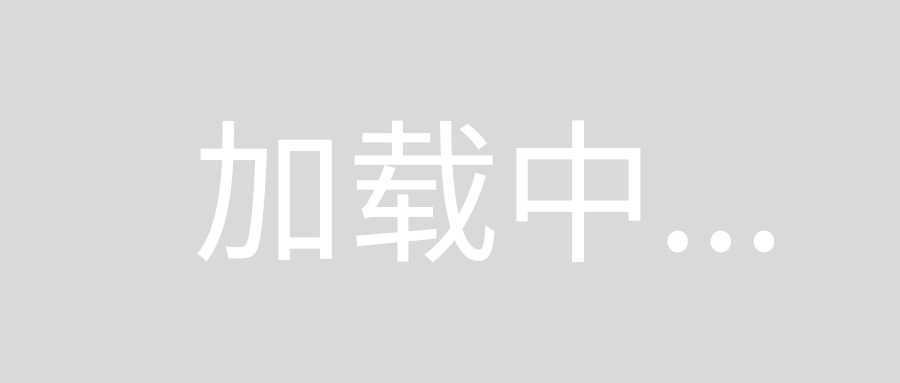
I want to display the label shown as 30 km with respect to the slider thumb such that as the slider moves, the label should move accordingly.
I am using Native React Slider component.
this is my code:
<Slider
style={styles.slider}
thumbTintColor='rgb(252, 228, 149)'
step={1}
maximumValue={5}
thumbTintColor='rgb(252, 228, 149)'
maximumTrackTintColor='#494A48'
minimumTrackTintColor='rgb(252, 228, 149)' />
Solution to your problem:
constructor(props){
super(props)
this.state = {
distance: 30,
minDistance: 10,
maxDistance: 100
}
}
render() {
return (
<View style={styles.container}>
<Slider
style={{ width: 300}}
step={1}
minimumValue={this.state.minDistance}
maximumValue={this.state.maxDistance}
value={this.state.distance}
onValueChange={val => this.setState({ distance: val })}
thumbTintColor='rgb(252, 228, 149)'
maximumTrackTintColor='#d3d3d3'
minimumTrackTintColor='rgb(252, 228, 149)'
/>
<View style={styles.textCon}>
<Text style={styles.colorGrey}>{this.state.minDistance} km</Text>
<Text style={styles.colorYellow}>
{this.state.distance + 'km'}
</Text>
<Text style={styles.colorGrey}>{this.state.maxDistance} km</Text>
</View>
</View>
);
}
}
Styles:
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#000',
},
textCon: {
width: 320,
flexDirection: 'row',
justifyContent: 'space-between'
},
colorGrey: {
color: '#d3d3d3'
},
colorYellow: {
color: 'rgb(252, 228, 149)'
}
});
Output:
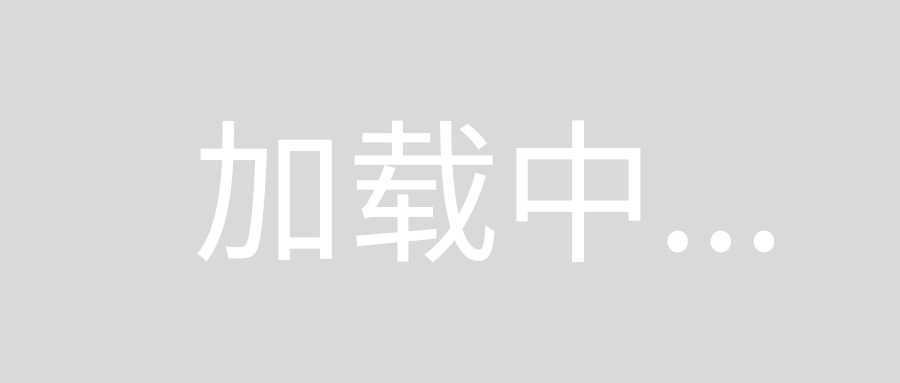
Working Snippet:
https://snack.expo.io/Syrt3Bs7z
You can adjust left of the text to the value of the slider.
const left = this.state.value * (screenWidth-60)/100 - 15;
<Text style={ { width: 50, textAlign: 'center', left: left } }>
{Math.floor( this.state.value )}
</Text>
<Slider maximumValue={100}
value={this.state.value}
onValueChange={value => this.setState({ value })} />
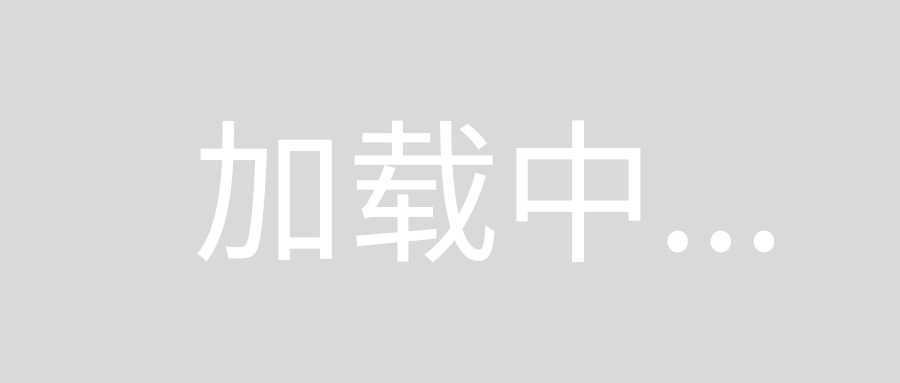
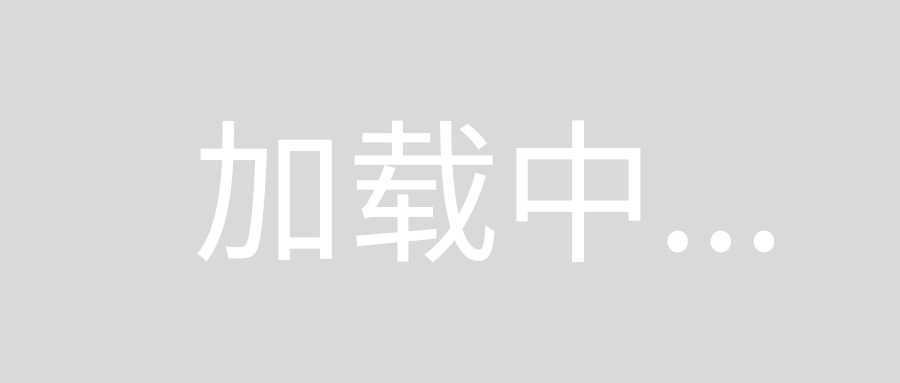
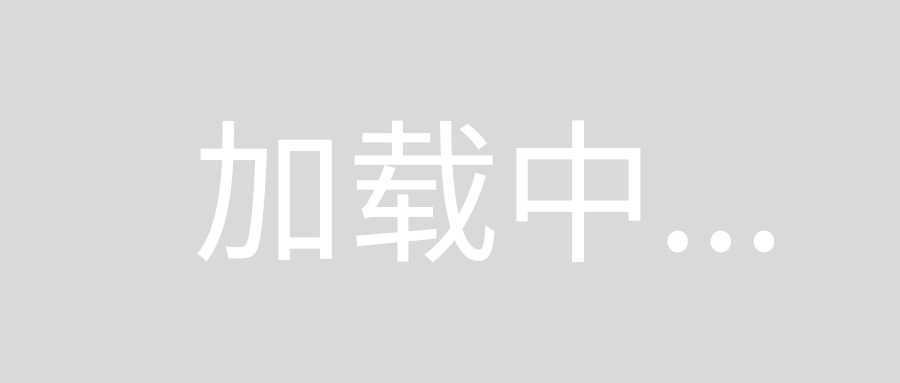
Built in <Slider />
doesn't provide those flexibility to customize what you want.
This should works, react-native-slider, which is a drop-in replacement of official <Slider />
.
What you need is very similar to it's demo style #4.
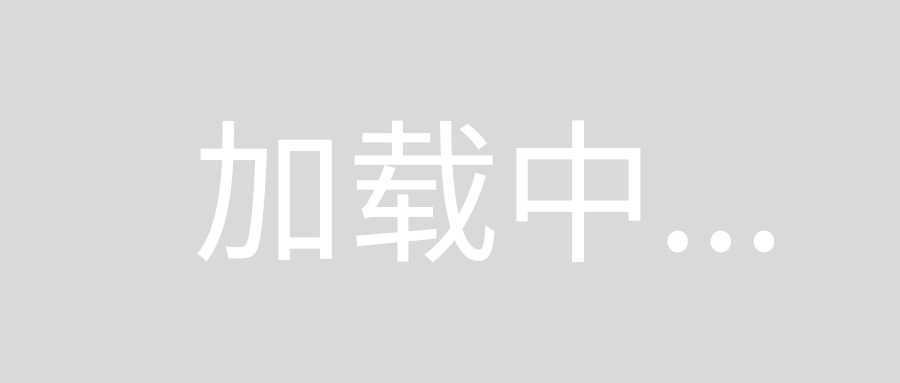
For your Slider Label of value, you can modify its function _renderThumbImage() to replace default <Image />
.
measure the size and position of the slider View
<Slider
maximumValue={10}
onLayout={(event)=>{this.slider_bound(event)}}/>
//majore size of Slider.
slider_bound=(event)=>{
var {x, y, width, height} = event.nativeEvent.layout;
this.state.slider_Width=width;
this.state.slider_Height=height;
this.state.slider_x = x;
this.state.slider_y = y;
this.state.slider_x_step_size = width/10; //Devide the width by slider maximum value
this.setState({triger:''});
console.log(TAG+"Slider Dimen:"+width,height+'pos:',x,y);
}
2.Now in "onValueChange" callback of slider.
//compute position to show slider value txt
this.state.value_x = (value * this.state.slider_x_step_size) + this.state.slider_x;
this.state.value_y = this.state.slider_Height + this.state.slider_y;
Show the slider Value txt on Calculated positions.
<Text style={{position:'absolute',top:this.state.value_y,left:this.state.value_x,color:colors.blackc}}>{this.state.data.slider_value}</Text>
..............
that will do the job, but You might have to tweak it little bit.
I think react-native-multi-slider will solve your problem . You can change the slider thumb by sending your custom designed component to the customMarker
prop that is available. Then you can wrap the Multislider
in another component, maintain the state(for slider position value) there and send value as prop to your custom designed marker everytime the thumb position changes which can be detected using onValuesChange
prop.
This link might also help you.