I'm developing one application in which I have to add a custom layout on actionbar. Adding the custom layout is done, but when I'm adding the menu items on actionbar my custom layout changes it's position from right of the actionbar to center of the actionbar. Below is the image what I have achieved so far.
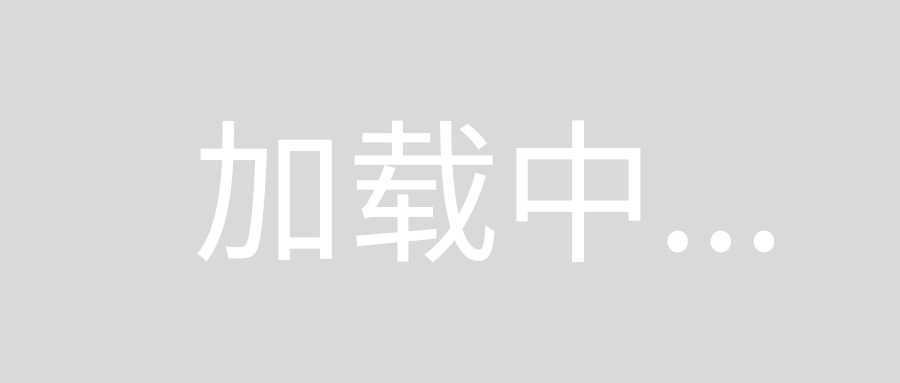
I want something like this on the actionbar.
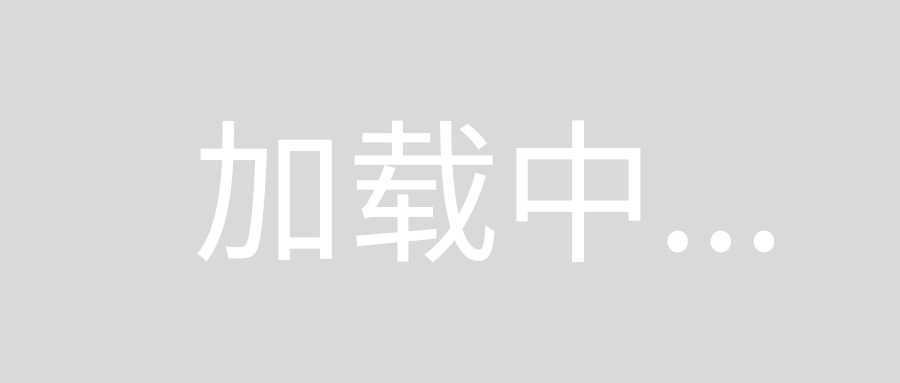
Custom layout(yellow button) at the right most part of actionbar and menu items in the middle.
Adding my code to achieve this custom layout using native android actionbar:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
getActionBar().setDisplayHomeAsUpEnabled(true);
getActionBar().setHomeButtonEnabled(true);
actionBar = getActionBar();
actionBar.setDisplayShowTitleEnabled(false);
actionBar.setDisplayUseLogoEnabled(false);
actionBar.setDisplayHomeAsUpEnabled(false);
actionBar.setDisplayShowCustomEnabled(true);
cView = getLayoutInflater().inflate(R.layout.header, null);
actionBar.setCustomView(cView);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.websearch_menu, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case android.R.id.home:
finish();
default:
return super.onOptionsItemSelected(item);
}
}
How can this be achieved?
Any kind of help will be appreciated.
I assume you are setting your custom view as a custom view in the action bar. If you are using ActionbarSherlock you can instead add the custom view as a normal menu item and set an action view for it using .setActionView(yourCustomView or yourCustomLayoutId)
. You can then set .setShowAsAction(MenuItem.SHOW_AS_ACTION_ALWAYS)
to display the view. Just make sure your custom view is set to width wrap_content
or otherwise it will push the other menu items off the screen. This might also work without ActionbarSherlock, but I haven't tried it there yet.
EDIT: Try modifying your onCreateOptionsMenu
method
@Override
public boolean onCreateOptionsMenu(Menu menu) {
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.websearch_menu, menu);
// add this
menu.add(Menu.NONE, 0, Menu.NONE, "custom")
.setActionView(R.layout.header)
.setShowAsAction(MenuItem.SHOW_AS_ACTION_ALWAYS);
return true;
}
and remove this from your onCreate method
actionBar.setDisplayShowCustomEnabled(true);
cView = getLayoutInflater().inflate(R.layout.header, null);
actionBar.setCustomView(cView);
EDIT:
If you need to reference the views from your custom layout, you can do this in your onCreateOptionsMenu
method as follows (note: I wrote this code in the browser, so no guarantee that I didn't make a typo or something):
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// inflate the menu and add the item with id viewId,
// which has your layout as actionview
View actionView = menu.getItem(viewId).getActionView();
View viewFromMyLayout = actionView.findViewById(R.id.viewFromMyLayout);
// here you can set listeners to your view or store a reference
// to it somewhere, so you can use it later
return true;
}
Just use orderInCategory
in your xml file and it will order from less number to greater (from left side of your action bar). Such as:
<item
android:id="@+id/Refresh"
android:icon="@drawable/ic_action_refresh"
android:showAsAction="ifRoom"
android:title="@string/refresh"
android:orderInCategory="2"/>
<item
android:id="@+id/Search"
android:icon="@drawable/ic_action_action_search"
android:showAsAction="ifRoom|collapseActionView"
android:title="@string/search"
android:orderInCategory="1"/>
Even though, Refresh item is coming first in xml file, it won't be first. But Search icon from left to rigth in your action bar will be first according to its orderInCategory attribute.
The order of the menu items will be set according to their order in the XML file that they are inflated.
Therefore all you need to do is change the order in the XML file as you like.
One way to achieve it is by calling invalidateOptionMenu and in the onCreateOptionsMenu method, put the items according to the order you wish to put them.
BTW, if you use ActionBarSherlock, this method is available , so ignore the Lint warnings about it.