I'm trying to use the new TabLayout in the android design library to create app bar with icons only.
like this:
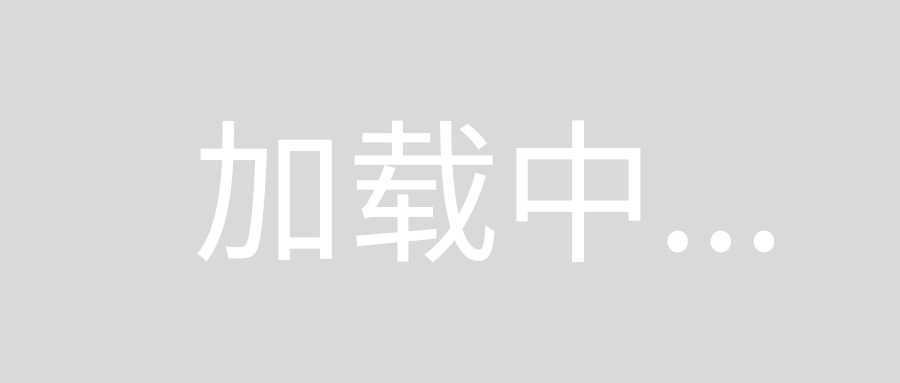
how can I do it using the new TabLayout Android Design Library.
is there a simple solution for this, or i have to use the setCustomView only. i'm trying to avoid using it. because i didn't get the tint color for the icon like this image above.
i try to write like this:
tabLayout.addTab(tabLayout.newTab().setIcon(R.drawable.ic_dashboard))
but the icon still stay in the same color when i select the tab
you have to create a selector
for the icon. For example:
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/ic_dashboard_pressed"
android:state_pressed="true" />
<item android:drawable="@drawable/ic_dashboard_selected"
android:state_selected="true" />
<item android:drawable="@drawable/ic_dashboard_normal" />
</selector>
I used it like this:
created an xml file in drawable as shown by @Budius.
in the code:
tabLayout.getTabAt(0).setIcon(R.drawable.settings_tab_drawable);
and so on.
i solved it like this:
tint_tab.xml
<com.hannesdorfmann.appkit.image.TintableImageView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:tint="@color/tab_color_selector"/>
in you java code
TintableImageView tab1 = (TintableImageView) LayoutInflater.from(this).inflate(R.layout.tint_tab, null);
tab1.setImageResource(R.drawable.ic_dummy);
mTabLayout.getTabAt(0).setCustomView(tab1)
ref: https://github.com/sockeqwe/appkit/blob/master/image/src/main/java/com/hannesdorfmann/appkit/image/TintableImageView.java