可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I have a collection of requests in POSTMAN. I wanted to add a pause between 2 requests but I couldn't find a way to do so from reading their docs. Any idea?
UPDATE I only wanted to put a pause after one request instead of after every request in the collection.
回答1:
In case someone is still looking for this - You can add delay after/before 1 of many test in a collection you can use:
setTimeout(function(){}, [number]);
where 'number' is the milliseconds. If it's added at 'Tests' it will be executed after request is send. If it's added at 'pre-request tests' it will be executed before request is send.
回答2:
I know two possible ways to do this
Method I
Run your request as a collection. (https://www.getpostman.com/docs/collections)
Use Newman (Postman's collection runner from command line) to run your collection with --delay flag. The delay input value is in milliseconds.
Method II
This is a nice hack which I found here https://github.com/postmanlabs/postman-app-support/issues/1038. You can add a delay function to your test script in Postman.
回答3:
Using javascript's busy wait is a good hack but it makes your CPU hot and the app unresponsive. I figured out this solution using postman-echo.
Assuming you want to add a long delay between Request_A and Request_B.
First, in Request_A's test script set up an env var to mark the start.
environment.delayTimerStart = new Date();
Then, create a GET request in the creation (here called 'Delay 10s'). It makes a GET on https://postman-echo.com/delay/10 (It returns after 10s)
In its test script, add
var curDate = new Date();
if (curDate - environment.delayTimerStart < delay_time_in_sec*1000) {
postman.setNextRequest('Delay 10s');
} else {
postman.setNextRequest("Request_B");
}
In this way you can add a delay of any length.
Note: 10 sec is the maxium value that postman-echo accepts. If you just need a short delay, simply GET https://postman-echo.com/delay/[1~10].
回答4:
Try something like this-
if(jsonBody.responseCode=="SUCCESS"){
setTimeout(function(){
console.log("Sleeping for 3 seconds before next request.");
}, 3000);
postman.setNextRequest("nextAPI");
}
回答5:
If you have the standalone Postman App (supports ES7) and you are intending to test only on Postman, not on newman(which doesn't support ES7), then you can have something like this in the Pre-Request script of the Request you want to delay:
function foo() {
return (new Promise((resolve, reject) => {
setTimeout(() => {
resolve("done!"); // passing argument is optional, can just use resolve()
}, 10000) // specify the delay time in ms here..
}))
}
async function waitForMe() {
await foo().then((val) => {
console.log(val); // not required, you can just do an await without then
})
}
waitForMe();
回答6:
looking at the current documentation if you are using Postman Runner
Delay
This is the interval (in ms) between each request in your collection run.
https://www.getpostman.com/docs/postman/collection_runs/starting_a_collection_run
and if you are using newman
--delay-request [number] Specify a delay (in ms) between requests [number]
https://www.getpostman.com/docs/postman/collection_runs/command_line_integration_with_newman
回答7:
I prefer to use an online service Postman Echo's delay endpoint (docs are here). Simply create a request that uses this service and call it between the two other requests you wish to add a delay between.
If you want to check the status of something before continuing, you can use postman.setNextRequest()
in the Tests
of a request to loop until something has been completed. Simply do the following:
1) Create a collection structured as:
- Delay For 10 Seconds
- Status Check
- Continue Processing
2) In the Status Check
request Tests:
if(responseBody.has("Success")) //or any other success condition
{
postman.setNextRequest('Continue Processing');
tests["Success found"] = true;
}
else
{
postman.setNextRequest('Delay For 10 Seconds');
tests["No success found"] = true;
}
回答8:
Just simple example, I'm sure you will be understand.
setTimeout(() => {}, 15000);
15000 it's a value in miliseconds
回答9:
You can use JavaScript setTimeout method. This will make sure that your method will be executed after given delay.
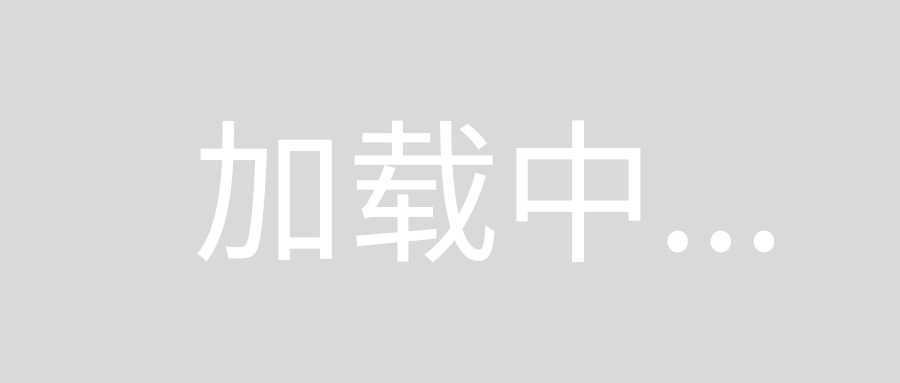
setTimeout(checkStatusCode, 500);
function checkStatusCode() {
pm.sendRequest('https://postman-echo.com/get', function (err, res) {
tests['status code should be 200']= res.code ===200;
});
}