It is known that when we deny permissions at runtime in Android 6.0 and resume the app from the recent menu, the app process is killed and the app is forcibly restarted. This is allegedly in order to prevent any security snafus:
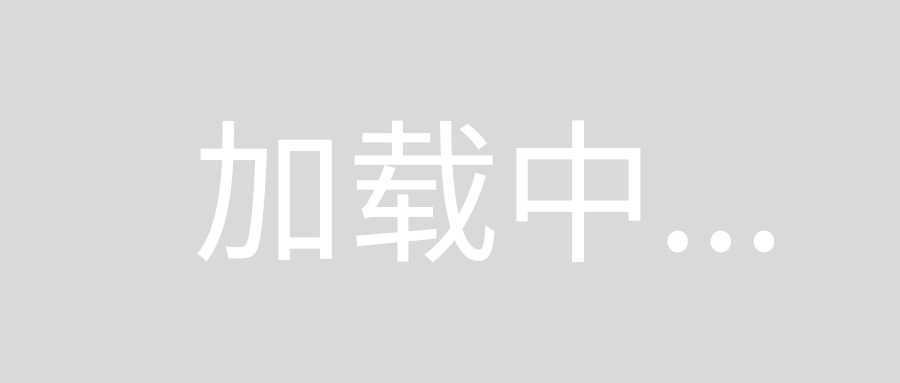
It is remarkable that, when resumed, the app restarts from the last Activity
we left it on. The OS apparently keeps track of the last Activity
the user visited.
Unfortunately, this is a problem, as it breaks the flow of the business logic. The user cannot be allowed to access the app midway in this manner.
My question is, is there a way to force the app to restart from the first Activity
of the application, instead of the one the user left it on?
Are there any callbacks associated with an application restart / process kill / permissions toggle?
Is this a wrong-headed approach? If so, how? And what would be the correct approach?
This behaviour has of course been observed before:
1. Android Preview M: activity recreates after permission grant
2. Application is getting killed after enable/disable permissions from settings on Android M nexus 6
3. Android Marshmallow: permissions change for running app
4. All the permissions of my app are revoked after pressing “Reset app preferences”
5. Android 6 permission - Crashes when toggling permission in Setting and go back to app
Did u mean this?
MainActivity:
public class MainActivity extends AppCompatActivity {
TextView txt;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
txt = (TextView)findViewById(R.id.txt);
txt.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(MainActivity.this, ActivityB.class);
intent.putExtra("from","MainActivity");
startActivity(intent);
finish();
}
});
}
}
ActivityB :
public class ActivityB extends AppCompatActivity {
String intentTxt="";
final int MY_PERMISSIONS_REQUEST_PHONE_CALL = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_b);
intentTxt = getIntent().getStringExtra("from");
}
@Override
protected void onResume() {
super.onResume();
if(intentTxt.equals("MainActivity")) {
if (ContextCompat.checkSelfPermission(ActivityB.this, android.Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(ActivityB.this, new String[]{android.Manifest.permission.CALL_PHONE},
MY_PERMISSIONS_REQUEST_PHONE_CALL);
}
}
intentTxt = "";
}
@Override
public void onRequestPermissionsResult(int requestCode,
String permissions[], int[] grantResults) {
switch (requestCode) {
case MY_PERMISSIONS_REQUEST_PHONE_CALL: {
if (grantResults.length > 0
&& grantResults[0] == PackageManager.PERMISSION_GRANTED) {
Intent intent = new Intent(Intent.ACTION_CALL, Uri.parse("tel:" + "32434"));
startActivity(intent);
} else {
Intent intent = new Intent(ActivityB.this,MainActivity.class);
startActivity(intent);
finish();
}
return;
}
}
}
}
You can start the splashActivity/HomeActivity from onRequestPermissionsResult of ActivityB.Check for permission in onResume of ActivityB so that the permission dialog appears when the ActivityB is called for the first time and also when resumed.
You should be handling Activity
(re)creation using the Bundle
provided by onCreate
, saved by onSaveInstanceState
, more information here.
Pass in the Bundle
all you need in order to recover from a previous state and restore your UI seamlessly. Your Activity
can be recreated by many reasons, cancellation of permissions is just one of them, screen rotation is another, so as long as you can survive one, you can survive all of them.
I don't know if anyone is still looking for an answer on this, but I'd solve by reversing the logic, instead of waiting for a flag indicating the restart/recreate due to the denial of permission, pass a flag indicating that the Activity was started by another Activity and not recreated by the system. So if FLAG doesn't come in extras then the activities stack must be destroyed and first activity lauched.
Hope it helps someone.