可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I am creating an angular library (version 6) which is based on angular material for which I need to include the hammer js library.
I know that in angular 6 we can add the external js library in the angular.json
under the project's configuration. But this does not work in case of above library. I tried to add the external library in the following way.
"my-library": {
"root": "projects/my-library",
"sourceRoot": "projects/my-library/src",
"projectType": "library",
"architect": {
"build": {
"builder": "@angular-devkit/build-ng-packagr:build",
"options": {
"tsConfig": "projects/my-library/tsconfig.lib.json",
"project": "projects/my-library/ng-package.json",
"scripts": [
"../node_modules/hammerjs/hammer.min.js"
]
}
}
}
But I am getting this error.
Schema validation failed with the following errors:
Data path "" should NOT have additional properties(scripts).
Please suggest what is the correct way to add external js file in the angular library.
回答1:
you can add your external library in the tsconfig.lib.json file.
Open that file and add your desires library under types node that you can find in compilerOptios. I give you and example. In my case I referenced the library from my project node module. You can do the same with your hammer.js library. Good luck
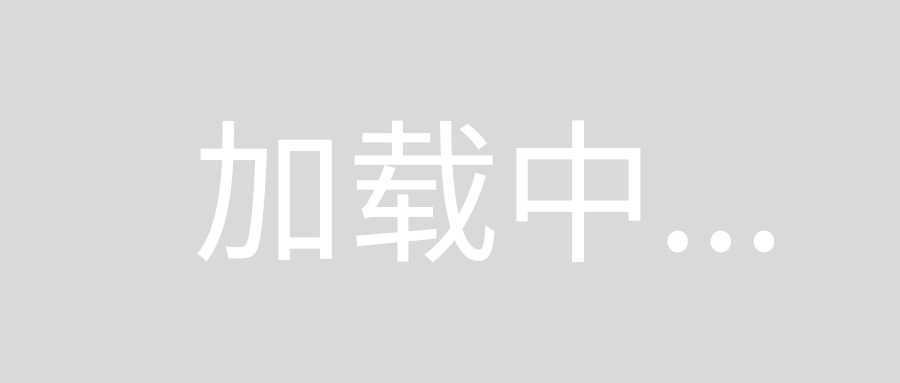
回答2:
Regarding the OP's specific example trying to add hammer.js. Just npm install hammerjs then edit your main.ts file and add import 'hammerjs'
then it will be available globally.
Also see this article.
回答3:
remove ../
before path of node_modules
"scripts": [
"node_modules/hammerjs/hammer.min.js"
]
回答4:
You should try below command if you have used angular version 1.x before. And it works for me.
ng update @angular/cli --migrate-only --from=1.7.4
回答5:
So here are two possible problems we need to address
1) How to add the reference of external JS in the main angular project(demo project)
2) How to add the reference of external JS in NPM package.
The solution for the 1'st scenario is :
Provide the reference of your external JS in your angular.json
file of main angular project in a script tag and provide the path of your package from your libraries node_modules folder like this.
"scripts": [ "./projects/my-cool-library/node_modules/my-exteranl-library/dist/x.js"]
The solution for the 2'nd scenario is :
Approach 1
So now you have created the NPM package from your library and you are going to use it in different project. obviously your 3rd party package dependency will get auto downloaded once you download your package you just have to provide the reference of that JS in script tag of angular.json
file of new project.
"scripts": [ "./node_modules/my-exteranl-library/dist/x.js"]
Approach 2
Don't specify your third party dependency while creating your NPM package
remove the entry from package.json
file of your cool-library
"dependencies": {
"my-exteranl-library": "^1.1.0" <-- Remove this
}
and add the js directly in newly created application via CDN in index.html file using script tag
<script src="https://demo-cdn.com/ajax/libs/my-exteranl-library/dist/x.js"></script>
There is 3rd way very you can download the JS by writing the code in your library will share here shortly.
回答6:
first add the libbrary in index.html or in
"scripts": [
"node_modules/hammerjs/hammer.min.js"
]
npm install d3 --save
npm install @types/d3 --save-dev
If the library doesn't have typings available at @types/, you can still use it by manually adding typings for it:
- First, create a
typings.d.ts
file in your src/
folder. This file will be automatically included as global type definition.
Then, in src/typings.d.ts
, add the following code:
declare module 'typeless-package';
Finally, in the component or file that uses the library, add the following code:
import * as typelessPackage from 'typeless-package';
typelessPackage.method();
please follow the link
回答7:
There two possible ways to solve this problem:
1) In your library specify @angular/material
and hammerjs
as peer dependencies so that all the applications that will use your library are required to provide the library themselves (this means that each app will have it's own angular.json
with the scripts
property containing the hammerjs
js file)
2) Use ng-cli-packagr-tasks
custom build steps to copy a hammen.min.js
file from node_modules
to the dist of a library and use relative import in your code.
Let me explain step #2 more detailed:
you want to use some kind of relative import like require('../dependencies/hammer.min.js')
but this will result an error because after the library is build, the ../dependencies/hammer.min.js
will lead to uncertain place. To make sure that each time not only your .ts
files will be magically transpiled to .js
files but also the static .js
files will be copied to a certain directory of a dist
output of a library, you use ng-cli-packagr-tasks
. After the build phase the ng-cli-packagr-tasks
will copy the files using glob
so that after you install the library in your application, the static .js
files will be bundled as well. This means that the import import('../dependencies/hammer.min.js).then()
will always lead to the file you've provided by yourself.
回答8:
To load js file externally from assets
create service file add file to the assets and write path in array.
import { Injectable } from "@angular/core";
declare var document: any;
@Injectable({
providedIn:'root'
})
export class ScriptService {
private scripts: any = {};
constructor() {
ScriptConstant.forEach((script: any) => {
this.scripts[script.name] = {
loaded: false,
src: script.src
};
});
}
load(...scripts: string[]) {
var promises: any[] = [];
scripts.forEach(script => promises.push(this.loadScript(script)));
return Promise.all(promises);
}
loadAll() {
var promises: any[] = [];
ScriptConstant.forEach(script => {
// promises.push(delay(1000));
promises.push(this.loadScript(script.name));
});
return Promise.all(promises);
}
loadScript(name: string) {
return new Promise((resolve, reject) => {
//resolve if already loaded
if (this.scripts[name].loaded) {
resolve({ script: name, loaded: true, status: "Already Loaded" });
} else {
//load script
let script = document.createElement("script");
script.type = "text/javascript";
script.src = this.scripts[name].src;
if (script.readyState) {
//IE
script.onreadystatechange = () => {
if (
script.readyState === "loaded" ||
script.readyState === "complete"
) {
script.onreadystatechange = null;
this.scripts[name].loaded = true;
resolve({ script: name, loaded: true, status: "Loaded" });
}
};
} else {
//Others
script.onload = () => {
this.scripts[name].loaded = true;
resolve({ script: name, loaded: true, status: "Loaded" });
};
}
script.onerror = (error: any) =>
resolve({ script: name, loaded: false, status: "Loaded" });
document.getElementsByTagName("body")[0].appendChild(script);
}
});
}
}
interface Scripts {
name: string;
src: string;
}
export const ScriptConstant: Scripts[] = [
{ name: "multislider", src: "assets/js/multislider.js" },
];
Inject this ScriptService wherever you need it and load js libs like this
this.script.load('multislider').then(data => {
console.log('script loaded ', data);
}).catch(error => console.log(error));
回答9:
You can't, that's the behavior of ng-packagr
, you'll get that error every time you try to add assets to a Library.
check this discussion for possible solution : https://github.com/angular/angular-cli/issues/11071