Want to improve this question? Update the question so it's on-topic for Stack Overflow.
Closed 3 years ago.
I've put a video in my site using an iframe
from YouTube, while the iframe
is full width (100%
) the video is very small (height) within the frame. How do I make it fit the width of the container?
Code:
<iframe width="100%" height="100%" src="https://www.youtube.com/embed/5m_ZUQTW13I" frameborder="0" allowfullscreen></iframe>
See screenshot
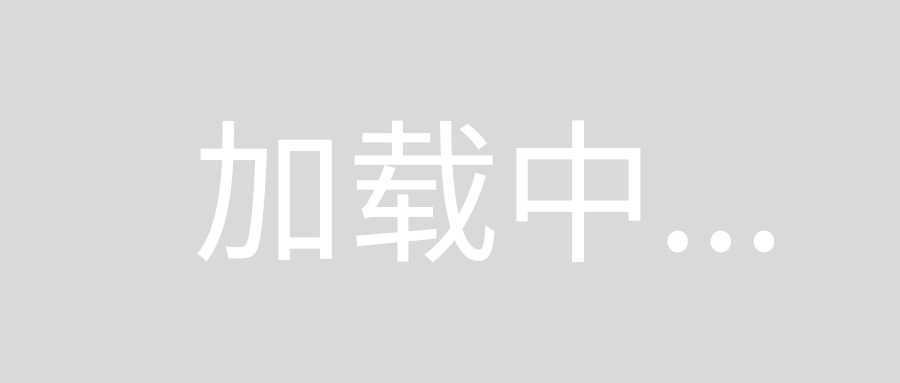
To make a You Tube Video full width and preserve the height (and keep it responsive) you can use the following setup.
HTML with video
<div class="videoWrapper">
<!-- Copy & Pasted from YouTube -->
<iframe width="560" height="349" src="http://www.youtube.com/embed/n_dZNLr2cME?rel=0&hd=1" frameborder="0" allowfullscreen></iframe>
</div>
CSS
.videoWrapper {
position: relative;
padding-bottom: 56.25%; /* 16:9 */
padding-top: 25px;
height: 0;
}
.videoWrapper iframe {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
The issue is that an iframe and video can't scale like an image, where it gets the proportionally correct height based on the aspect ratio if you just leave the height at the default.
A solution for this is to figure out the native width/height of your video, and do a little math to figure out what the height should be at 100% screen width. Assuming it's 1920x1080, you can do something like this using viewport width (vw). You can reduce the 100vw to whatever fits better, if you don't want it to literally fill the screen.
iframe{
width: 100vw
height: calc(100vw/1.77);
}
Use the fullscreen attribute:
<html>
<body>
<iframe id="myFrame" src="http://www.youtube.com/embed/W7qWa52k-nE" frameborder="0" allowfullscreen></iframe>
</body>
</html>
If it not worked then also include the following css code:
#myFrame{
position:relative;
top:0;
left:0;
width:100%;
height:100%;