可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I've tried react-native-hr package - doesn't work for me nor on Android nor on iOS.
Following code is also not suitable because it renders three dots at the end
<Text numberOfLines={1}}>
______________________________________________________________
</Text>
回答1:
You could simply use an empty View with a bottom border.
<View
style={{
borderBottomColor: 'black',
borderBottomWidth: 1,
}}
/>
回答2:
One can use margin in order to change the width of a line and place it center.
import { StyleSheet } from 'react-native;
<View style = {styles.lineStyle} />
const styles = StyleSheet.create({
lineStyle:{
borderWidth: 0.5,
borderColor:'black',
margin:10,
}
});
if you want to give margin dynamically then you can use Dimension width.
回答3:
I recently had this problem.
<Text style={styles.textRegister}> ──────── Register With ────────</Text>
with this result:
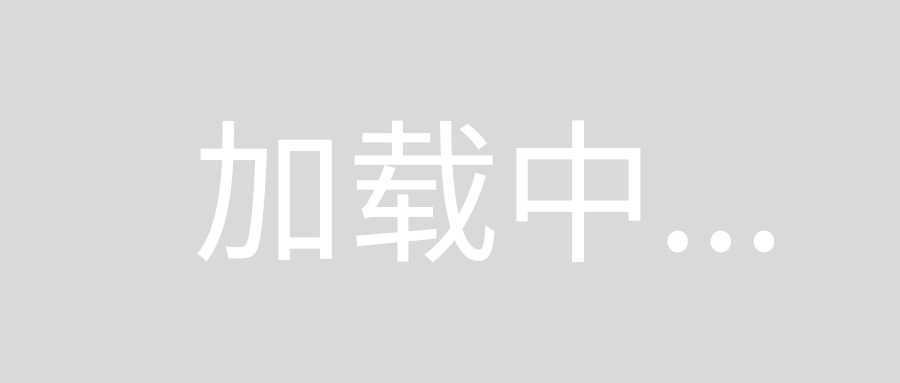
回答4:
I did it like this. Hope this helps
<View style={styles.hairline} />
<Text style={styles.loginButtonBelowText1}>OR</Text>
<View style={styles.hairline} />
for style:
hairline: {
backgroundColor: '#A2A2A2',
height: 2,
width: 165
},
loginButtonBelowText1: {
fontFamily: 'AvenirNext-Bold',
fontSize: 14,
paddingHorizontal: 5,
alignSelf: 'center',
color: '#A2A2A2'
},
回答5:
You can also try react-native-hr-component
npm i react-native-hr-component --save
Your code:
import Hr from 'react-native-hr-component'
//..
<Hr text="Some Text" fontSize={5} lineColor="#eee" textPadding={5} textStyles={yourCustomStyles} hrStyles={yourCustomHRStyles} />
回答6:
This is in React Native (JSX) code, updated to today:
<View style = {styles.viewStyleForLine}></View>
const styles = StyleSheet.create({
viewStyleForLine: {
borderBottomColor: "black",
borderBottomWidth: StyleSheet.hairlineWidth,
alignSelf:'stretch',
width: "100%"
}
})
you can use either alignSelf:'stretch'
or width: "100%"
both should work...
and,
borderBottomWidth: StyleSheet.hairlineWidth
here StyleSheet.hairlineWidth
is the thinnest,
then,
borderBottomWidth: 1,
and so on to increase thickness of the line.
回答7:
Why don't you do something like this?
<View
style={{
borderBottomWidth: 1,
borderBottomColor: 'black',
width: 400,
}}
/>
回答8:
import { View, Dimensions } from 'react-native'
var { width, height } = Dimensions.get('window')
// Create Component
<View style={{
borderBottomColor: 'black',
borderBottomWidth: 0.5,
width: width - 20,}}>
</View>
回答9:
Creating a reusable Line
component worked for me:
import React from 'react';
import { View } from 'react-native';
export default function Line() {
return (
<View style={{
height: 1,
backgroundColor: 'rgba(255, 255, 255 ,0.3)',
alignSelf: 'stretch'
}} />
)
}
Now, Import and use Line
anywhere:
<Line />
回答10:
Maybe you should try using react-native-hr something like this:
<Hr lineColor='#b3b3b3'/>
documentation: https://www.npmjs.com/package/react-native-hr
回答11:
import {Dimensions} from 'react-native'
const { width, height } = Dimensions.get('window')
<View
style={{
borderBottomColor: '#1D3E5E',
borderBottomWidth: 1,
width : width ,
}}
/>
回答12:
This is how i solved divider with horizontal lines and text in the midddle:
<View style={styles.divider}>
<View style={styles.hrLine} />
<Text style={styles.dividerText}>OR</Text>
<View style={styles.hrLine} />
</View>
And styles for this:
import { Dimensions, StyleSheet } from 'react-native'
const { width } = Dimensions.get('window')
const styles = StyleSheet.create({
divider: {
flexDirection: 'row',
alignItems: 'center',
marginTop: 10,
},
hrLine: {
width: width / 3.5,
backgroundColor: 'white',
height: 1,
},
dividerText: {
color: 'white',
textAlign: 'center',
width: width / 8,
},
})
回答13:
You can use native element divider.
Install it with:
npm install --save react-native-elements
# or with yarn
yarn add react-native-elements
import { Divider } from 'react-native-elements'
Then go with:
<Divider style={{ backgroundColor: 'blue' }} />
Source
回答14:
Just create a View component which has small height.
<View style={{backgroundColor:'black', height:10}}/>
回答15:
If you have a solid background (like white), this could be your solution:
<View style={container}>
<View style={styles.hr} />
<Text style={styles.or}>or</Text>
</View>
const styles = StyleSheet.create({
container: {
flex: 0,
backgroundColor: '#FFFFFF',
width: '100%',
},
hr: {
position: 'relative',
top: 11,
borderBottomColor: '#000000',
borderBottomWidth: 1,
},
or: {
width: 30,
fontSize: 14,
textAlign: 'center',
alignSelf: 'center',
backgroundColor: '#FFFFFF',
},
});
回答16:
I was able to draw a separator with flexbox
properties even with a text in the center of line.
<View style={{flexDirection: 'row', alignItems: 'center'}}>
<View style={{flex: 1, height: 1, backgroundColor: 'black'}} />
<View>
<Text style={{width: 50, textAlign: 'center'}}>Hello</Text>
</View>
<View style={{flex: 1, height: 1, backgroundColor: 'black'}} />
</View>
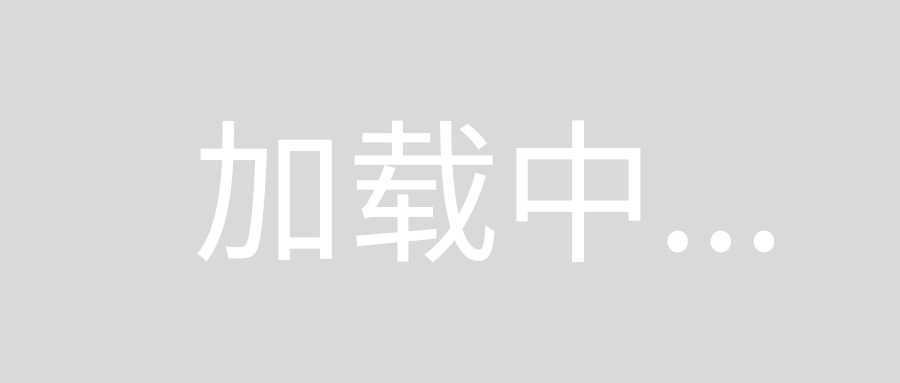