I realize there are many similar solutions, such as TPKeyboardAvoiding, Apple's famous solution, and various suggestions involving the use of UIScrollView. In my case, I need to resize a view to accommodate the keyboard rather than scroll or move it. This solution comes closest to what I'm trying to achieve, so it was my basis. However I'm having an issue making things work in landscape mode. My method that resizes the view when the keyboard appears is this:
- (void)keyboardWillShow:(NSNotification *)note {
NSDictionary *userInfo = note.userInfo;
NSTimeInterval duration = [[userInfo objectForKey:UIKeyboardAnimationDurationUserInfoKey] doubleValue];
UIViewAnimationCurve curve = [[userInfo objectForKey:UIKeyboardAnimationCurveUserInfoKey] intValue];
CGRect keyboardFrame = [[self textField].superview convertRect:[[userInfo objectForKey:UIKeyboardFrameEndUserInfoKey] CGRectValue] fromView:nil];
CGRect statusBarFrame = [[self textField].superview convertRect:[UIApplication sharedApplication].statusBarFrame fromView:nil];
CGRect bounds = [self textField].superview.bounds;
CGRect newFrame = CGRectMake(0.0, 0.0, bounds.size.width, keyboardFrame.origin.y + statusBarFrame.size.height);
[UIView animateWithDuration:duration delay:0 options:UIViewAnimationOptionBeginFromCurrentState | curve animations:^{
[self textField].superview.frame = newFrame;
} completion:nil];
}
This works perfectly in portrait mode.
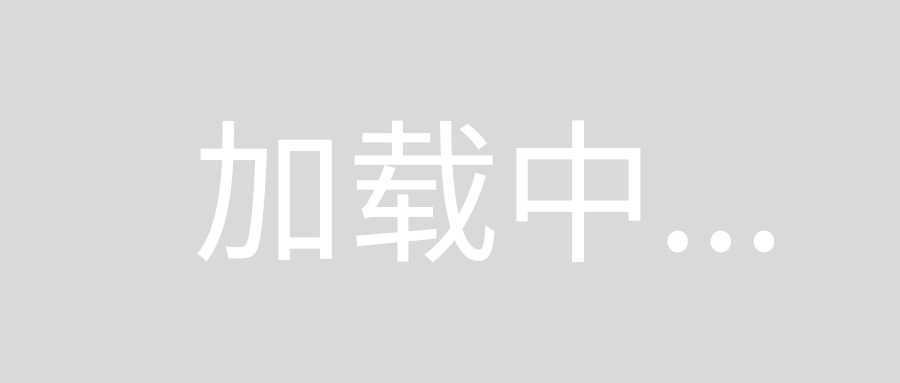
However, in landscape mode, the view resizes from left-to-right or right-to-left depending upon in which direction the device was rotated, rather than from the bottom up.
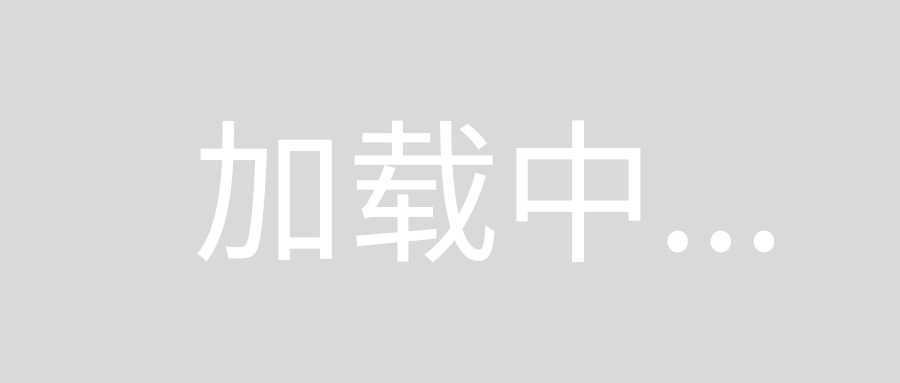
Clearly there is something wrong with how I'm using coordinates, and some frame of reference isn't what I think it is when in landscape mode, but I'm having a heck of a time sorting out how to resolve it. I've tried converting all kinds of things with -convertRect: but nothing I'm trying is getting me anywhere.
I'm really hoping someone who's less confused by all these rectangles and how they change when orientation changes can spot what I'm doing wrong and what I need to do to get this right. For reference, I've created a project showing the simplest case that reproduces the problem I'm having.
I do not advise you to resize root view for your view controller, you can create contentView and add to view of view controller. You can change size of this contentView as below (I don't use autolayouting):
- (void)keyboardWillShow:(NSNotification *)note {
NSDictionary *userInfo = note.userInfo;
NSTimeInterval duration = [userInfo[UIKeyboardAnimationDurationUserInfoKey] doubleValue];
UIViewAnimationCurve curve = [userInfo[UIKeyboardAnimationCurveUserInfoKey] integerValue];
CGRect keyboardFrameEnd = [userInfo[UIKeyboardFrameEndUserInfoKey] CGRectValue];
keyboardFrameEnd = [self.view convertRect:keyboardFrameEnd fromView:nil];
[UIView animateWithDuration:duration delay:0 options:UIViewAnimationOptionBeginFromCurrentState | curve animations:^{
self.contentView.frame = CGRectMake(0, 0, keyboardFrameEnd.size.width, keyboardFrameEnd.origin.y);
} completion:nil];
}
- (void)keyboardWillHide:(NSNotification *)note {
NSDictionary *userInfo = note.userInfo;
NSTimeInterval duration = [userInfo[UIKeyboardAnimationDurationUserInfoKey] doubleValue];
UIViewAnimationCurve curve = [userInfo[UIKeyboardAnimationCurveUserInfoKey] integerValue];
CGRect keyboardFrameEnd = [userInfo[UIKeyboardFrameEndUserInfoKey] CGRectValue];
keyboardFrameEnd = [self.view convertRect:keyboardFrameEnd fromView:nil];
[UIView animateWithDuration:duration delay:0 options:UIViewAnimationOptionBeginFromCurrentState | curve animations:^{
self.contentView.frame = CGRectMake(0, 0, keyboardFrameEnd.size.width, keyboardFrameEnd.origin.y);
} completion:nil];
}
Vitaliy B's answer in swift. I got a view called templateHeaderContentView, I created a function and configured the view height there. You use your own view and change the height accordingly there.
func keyboardWillShow(notification: NSNotification) {
keyboardShowOrHide(notification)
}
func keyboardWillHide(notification: NSNotification) {
keyboardShowOrHide(notification)
}
private func keyboardShowOrHide(notification: NSNotification) {
guard let userInfo = notification.userInfo else {return}
guard let duration = userInfo[UIKeyboardAnimationDurationUserInfoKey]else { return }
guard let curve = userInfo[UIKeyboardAnimationCurveUserInfoKey] else { return }
guard let keyboardFrameEnd = userInfo[UIKeyboardFrameEndUserInfoKey] else { return }
let curveOption = UIViewAnimationOptions(rawValue: UInt(curve.integerValue << 16))
let keyboardFrameEndRectFromView = view.convertRect(keyboardFrameEnd.CGRectValue, fromView: nil)
UIView.animateWithDuration(duration.doubleValue ?? 1.0,
delay: 0,
options: [curveOption, .BeginFromCurrentState],
animations: { () -> Void in
self.templateHeaderContentView.configureView(keyboardFrameEndRectFromView.origin.y)
}, completion: nil)
}
Covered very well straight form the horses mouth:
https://developer.apple.com/library/ios/documentation/StringsTextFonts/Conceptual/TextAndWebiPhoneOS/KeyboardManagement/KeyboardManagement.html
i had done it hope this code will be helpfull for u.
- (void)viewWillAppear:(BOOL)animated
{
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(keyboardWillShow:)
name:UIKeyboardWillShowNotification
object:nil];
[[NSNotificationCenter defaultCenter] addObserver:self
selector:@selector(keyboardWillBeHidden:)
name:UIKeyboardWillHideNotification
object:nil];
}
- (void)keyboardWillShow:(NSNotification*)notification
{
[self moveControls:notification up:YES];
}
- (void)keyboardWillBeHidden:(NSNotification*)notification
{
[self moveControls:notification up:NO];
}
- (void)moveControls:(NSNotification*)notification up:(BOOL)up
{
NSDictionary* userInfo = [notification userInfo];
CGRect newFrame = [self getNewControlsFrame:userInfo up:up];
[self animateControls:userInfo withFrame:newFrame];
}
- (CGRect)getNewControlsFrame:(NSDictionary*)userInfo up:(BOOL)up
{
CGRect kbFrame = [[userInfo objectForKey:UIKeyboardFrameBeginUserInfoKey] CGRectValue];
kbFrame = [self.view convertRect:kbFrame fromView:nil];
CGRect newFrame = self.view.frame;
newFrame.origin.y += kbFrame.size.height * (up ? -1 : 1);
return newFrame;
}
- (void)animateControls:(NSDictionary*)userInfo withFrame:(CGRect)newFrame
{
NSTimeInterval duration = [[userInfo objectForKey:UIKeyboardAnimationDurationUserInfoKey] doubleValue];
UIViewAnimationCurve animationCurve = [[userInfo objectForKey:UIKeyboardAnimationCurveUserInfoKey] integerValue];
[UIView animateWithDuration:duration
delay:0
options:animationOptionsWithCurve(animationCurve)
animations:^{
self.view.frame = newFrame;
}
completion:^(BOOL finished){}];
}
static inline UIViewAnimationOptions animationOptionsWithCurve(UIViewAnimationCurve curve)
{
return (UIViewAnimationOptions)curve << 16;
}
Try this methods. Edit it according to your requirement.
#define kOFFSET_FOR_KEYBOARD 280.0
- (void)keyboardWillHide:(NSNotification *)notif {
[self setViewMoveUp:NO];
}
- (void)keyboardWillShow:(NSNotification *)notif{
[self setViewMoveUp:YES];
}
- (void)textFieldDidBeginEditing:(UITextField *)textField {
stayup = YES;
[self setViewMoveUp:YES];
}
- (void)textFieldDidEndEditing:(UITextField *)textField {
stayup = NO;
[self setViewMoveUp:NO];
}
//method to move the view up/down whenever the keyboard is shown/dismissed
-(void)setViewMoveUp:(BOOL)moveUp
{
[UIView beginAnimations:nil context:NULL];
[UIView setAnimationDuration:0.3]; // if you want to slide up the view
[UIView setAnimationBeginsFromCurrentState:YES];
CGRect rect = self.view.frame;
if (moveUp)
{
// 1. move the view's origin up so that the text field that will be hidden come above the keyboard
// 2. increase the size of the view so that the area behind the keyboard is covered up.
if (rect.origin.y == 0 ) {
rect.origin.y -= kOFFSET_FOR_KEYBOARD;
//rect.size.height += kOFFSET_FOR_KEYBOARD;
}
}
else
{
if (stayup == NO) {
rect.origin.y += kOFFSET_FOR_KEYBOARD;
//rect.size.height -= kOFFSET_FOR_KEYBOARD;
}
}
self.view.frame = rect;
[UIView commitAnimations];
}