I dynamically add features to my cluster, however, as far as I can see clustering does not work. My layer is defined like so:
var source = new ol.source.Vector({});
var cluster = new ol.source.Cluster({
distance: 10,
source: source
});
var style = new ol.style.Style({
fill: new ol.style.Fill({
color: "rgba(255,150,0,1)"
}),
stroke: new ol.style.Stroke({
color: "rgba(255,150,0,1)",
width: 1
}),
image: new ol.style.Circle({
radius: 1,
fill: new ol.style.Fill({
color: "rgba(255,150,0,1)"
})
}),
zIndex: 1
});
var layer = new ol.layer.Vector({
source: cluster,
style: style,
zIndex: 1
});
And I add multiple features (Point geometries) in bulk in one of my functions, which take the layer as an argument. It does it like so:
layer.getSource().clear();
layer.setVisible(true);
layer.getSource().addFeatures(features); // features is a large array of features
However, if I zoom in and zoom out, I see these pictures:
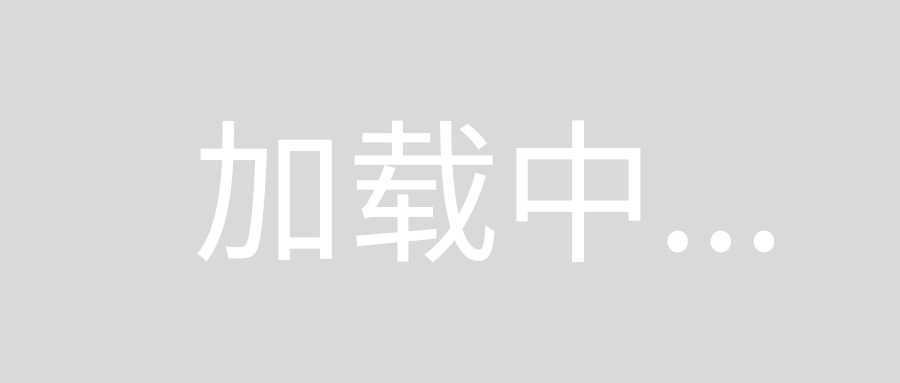
and:
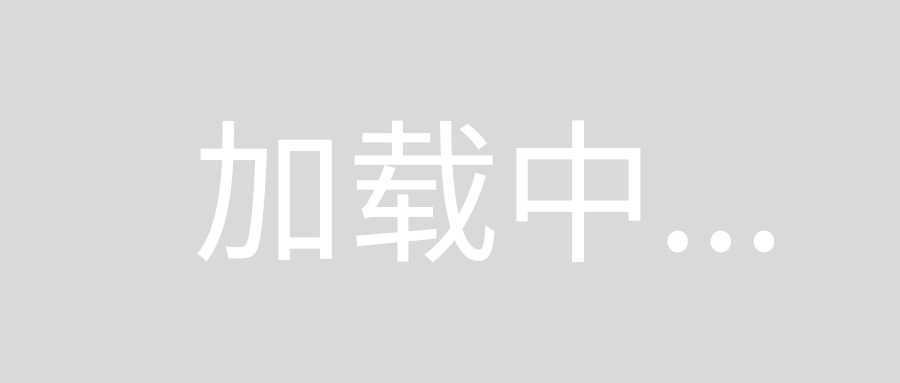
On the second screen shot, you can see, that my layer shows all features and ignores clustering parameter. Why is that and how can I fix it? (PS. If it matters, I'm using the latest version of OL)
this is working fine, i updates this example with your code and added features with random values
openlayers.org/en/latest/examples/cluster.html
<!DOCTYPE html>
<html>
<head>
<title>Clustered Features</title>
<link rel="stylesheet" href="https://openlayers.org/en/v4.6.5/css/ol.css" type="text/css">
<!-- The line below is only needed for old environments like Internet Explorer and Android 4.x -->
<script src="https://cdn.polyfill.io/v2/polyfill.min.js?features=requestAnimationFrame,Element.prototype.classList,URL"></script>
<script src="https://openlayers.org/en/v4.6.5/build/ol.js"></script>
</head>
<body>
<div id="map" class="map"></div>
<form>
<label>cluster distance</label>
<input id="distance" type="range" min="0" max="100" step="1" value="40"/>
</form>
<script>
var distance = document.getElementById('distance');
var source = new ol.source.Vector({
});
var cluster = new ol.source.Cluster({
distance: 10,
source: source
});
var style = new ol.style.Style({
fill: new ol.style.Fill({
color: "rgba(255,150,0,1)"
}),
stroke: new ol.style.Stroke({
color: "rgba(255,150,0,1)",
width: 1
}),
image: new ol.style.Circle({
radius: 1,
fill: new ol.style.Fill({
color: "rgba(255,150,0,1)"
})
}),
zIndex: 1
});
var clusters = new ol.layer.Vector({
source: cluster,
style: style,
zIndex: 1
});
var map = new ol.Map({
layers: [clusters],
target: 'map',
view: new ol.View({
center: [0, 0],
zoom: 2
})
});
var count = 20000;
var features = new Array(count);
var e = 4500000;
for (var i = 0; i < count; ++i) {
var coordinates = [2 * e * Math.random() - e, 2 * e * Math.random() - e];
/*features[i] =*/ source.addFeature(new ol.Feature(new ol.geom.Point(coordinates)));
}
distance.addEventListener('input', function() {
cluster.setDistance(parseInt(distance.value, 10));
});
</script>
</body>
</html>
Good Luck