I have a page in my MVC4 project where user can add its company logo using the file upload control. These images/logos are then shown on map in mobile application. We need to crop these images so that they can look like a Flag.
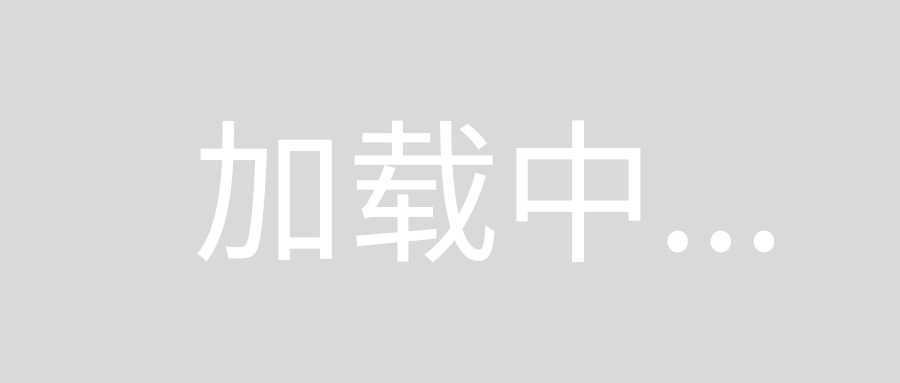
We need to take only the part of image inside the flag frame and leave the rest.
- Can it be done using code in C#?
- If yes, how it can be done. Please help me with some code samples and links.
- I need to show a flag frame over the uploaded image, so that user can adjust its image in that frame, what it wants to be in the frame.
Please suggest me with some APIs and code samples.
Thanks.
Update: In some sites, when we upload profile image, it gives us a frame on top, and the image we have selected can be moved, so that the desired part comes into that frame. Now when we upload our profile image, it gets resized into that size. Can I do something similar here? in the frame above, I can give a flag shape, user can move the uploaded image, to get desired part of image in that frame.
Is it right approach?
How can we do this? I have looked into some jquery code samples, but no help.
You can use SetClip function with the Region as parameter:
https://msdn.microsoft.com/en-us/library/x1zb278e(v=vs.110).aspx
So you need to create Graphics object from Bitmap, set clip with the shape of your flag and then draw image on that Graphics object. That's all.
// Following code derives a cutout bitmap using a
// scizzor path as a clipping region (like Paint would do)
// Result bitmap has a minimal suitable size, pixels outside
// the clipping path will be white.
public static Bitmap ApplyScizzors(Bitmap bmpSource, List<PointF> pScizzor)
{
GraphicsPath graphicsPath = new GraphicsPath(); // specified Graphicspath
graphicsPath.AddPolygon(pScizzor.ToArray()); // add the Polygon
var rectCutout = graphicsPath.GetBounds(); // find rectangular range
Matrix m = new Matrix();
m.Translate(-rectCutout.Left, -rectCutout.Top); // translate clip to (0,0)
graphicsPath.Transform(m);
Bitmap bmpCutout = new Bitmap((int)(rectCutout.Width), (int)(rectCutout.Height)); // target
Graphics graphicsCutout = Graphics.FromImage(bmpCutout);
graphicsCutout.Clip = new Region(graphicsPath);
graphicsCutout.DrawImage(bmpSource, (int)(-rectCutout.Left), (int)(-rectCutout.Top)); // draw
graphicsPath.Dispose();
graphicsCutout.Dispose();
return bmpCutout;
}