I've been trying to use LibCURL in C++ for a couple hours now, and it is really getting on my nerves. I have a feeling someone else has had a problem like this before, but I haven't found and posts that have given me a solution.
This is what I've done:
Since the libCurl download page is so confusing, I am posting exactly what I've done. First, I downloaded the file at the top (curl-7.23.1.zip), and then opened it in winRAR. I then went into the include folder, and then extracted the 'curl' folder out of there.
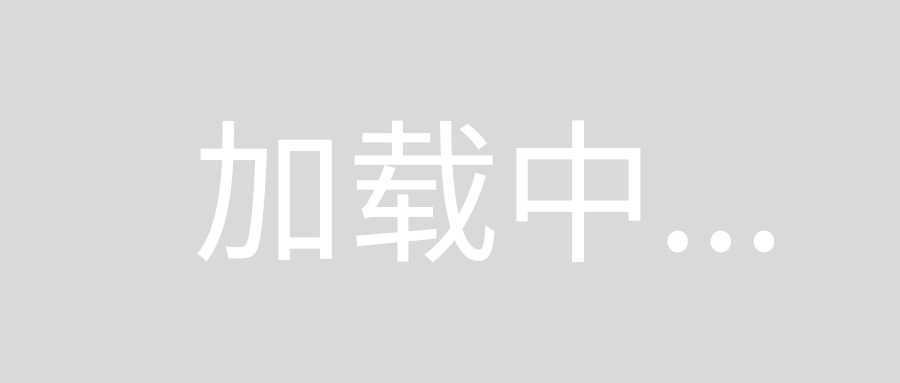
I then created a new project with Code::Blocks, and then moved the 'curl' folder into the same folder as my project.
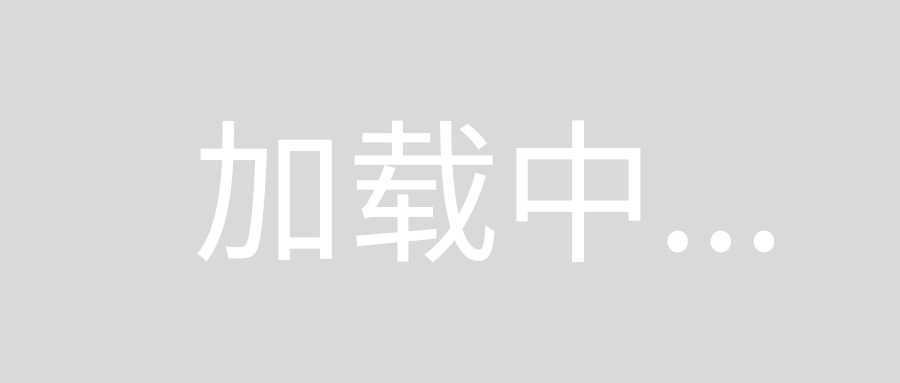
I then add '#include "curl/curl.h"' to the top of my file, and then try and initialize a simple CURL var... I then get an error, saying:
...\main.cpp|22|undefined reference to `_imp__curl_easy_init'|
Here is a picture of the actual code/error:
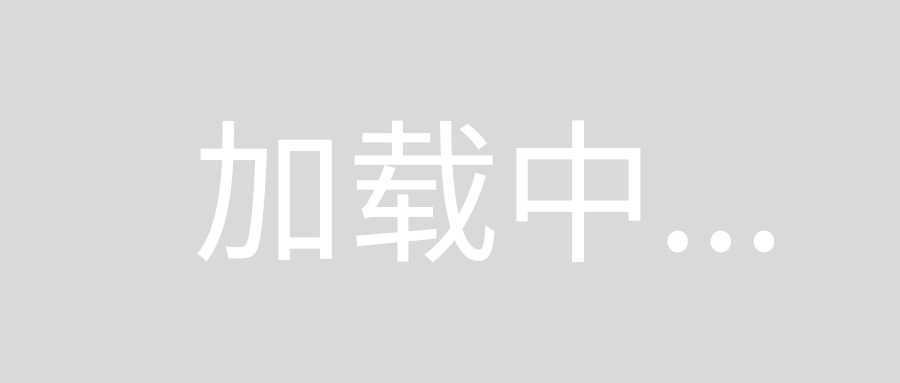
Honestly, I think it is something very very stupid that I am doing, but I just don't know what to do.
Finally got it to work with some of help
Here is how I did it:
- Download the 'Win32 Generic' libcurl package. (7.24.0)
- In Code::Blocks, right click your project and open the build options.
- Go to 'Linker Settings' and add 'curldll' into the 'Link Libraries' listbox. (image)
- Go to 'Search Directories' and under 'Compiler' link it to the path of your 'curl-7.24.0-devel-mingw32\include' folder.
- Go to the 'Linker' tab under 'Search Directories', and add the path of your 'curl-7.24.0-devel-mingw32\lib' directory.
- Move all DLLs from your 'curl-7.24.0-devel-mingw32\bin' folder into your projects 'bin' folder.
- Build and enjoy
To use libCurl with Qt, it is a bit easier.
- Move all necessary files/dlls/libraries into your debug folder. Make sure that you include the 'curl' folder.
Go to your .pro file, and add the location of the 'libcurldll.a' file. For example(mine):
LIBS += C:\libcurl\7.24.0\lib\libcurldll.a
Enjoy.
Thanks R. Martinho Fernandes!
You must also include the lib/
folder from libcurl, which contains *.a
or *.dll
files. Theses files are the library itself, the compiled binary on which you link your program.
If you link the library dynamically, you'll need to put the *.dll
in your project directory, in C:\Windows
or in C:\MinGW\bin
(if C:\MinGW is the compiler path).
"First, I downloaded the file at the top (curl-7.23.1.zip), and then opened it in winRAR. I then went into the include folder, and then extracted the 'curl' folder out of there."
It sounds like you didn't compile the source code for curl. All the downloads at the top of the page are just source.