My reducers are split, and in one of them my initial state and reducer looks like:
import Constants from '../constants';
const initialState = {
fetching: true,
};
const boards = (state = initialState, action) => {
switch (action.type) {
case Constants.BOARDS_FETCHING:
return state;
default:
return state;
}
};
export default boards;
How can I change the fetching property to true in the BOARDS_FETCHING case?
Update
My .babelrc looks like:
{
"presets": ["react", "es2015"],
"env": {
"development": {
"presets": ["react-hmre"]
}
}
}
Error:
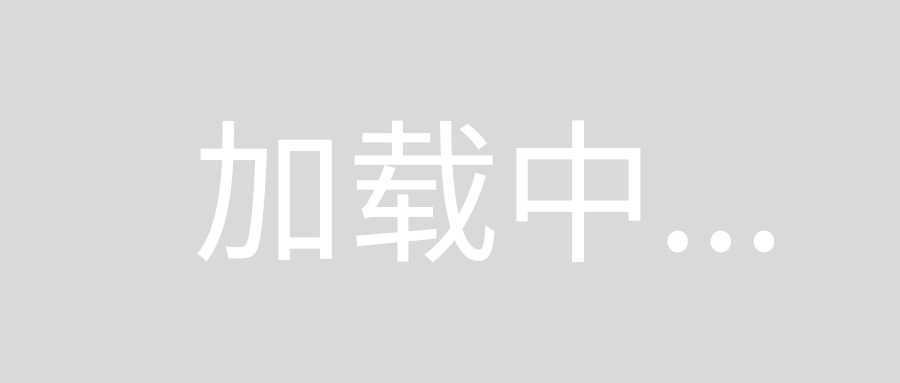
You need to install transform-object-rest-spread
"plugins": ["transform-object-rest-spread"]
For more, please check babeljs-plugins#transform-object-rest-spread
You should set it in the case Constants.BOARDS_FETCHING
clause:
import Constants from '../constants';
const initialState = {
fetching: true,
};
const boards = (state = initialState, action) => {
switch (action.type) {
case Constants.BOARDS_FETCHING:
return {...state, fetching: true};
default:
return state;
}
};
export default boards;
Replace this:
const boards = (state = initialState, action) => {
switch (action.type) {
case Constants.BOARDS_FETCHING:
return state;
default:
return state;
}
};
to:
const boards = (state = initialState, action) => {
switch (action.type) {
case Constants.BOARDS_FETCHING:
return Object.assign({},state,action.fetching);
default:
return state;
}
};