可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
i have a question view and will show 4 answers, only 1 are correct.
but i do not want the same button to be always the correct answer.
i will like to know how do i do a random placement of 4 UIButton and value every time.
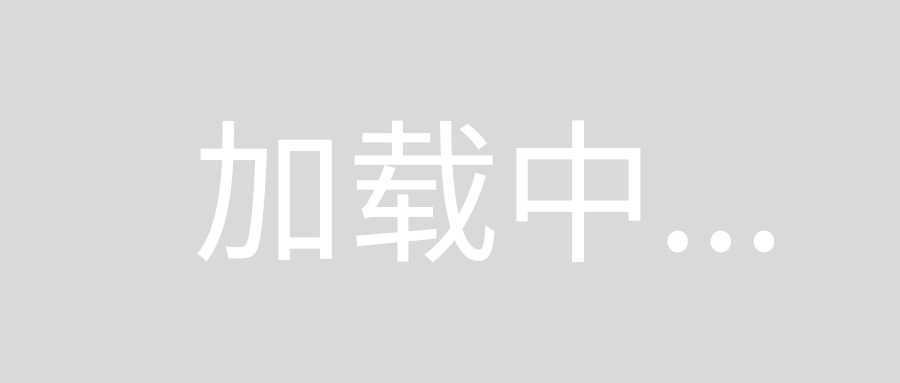
when user go to this question again the answer will be in differ button
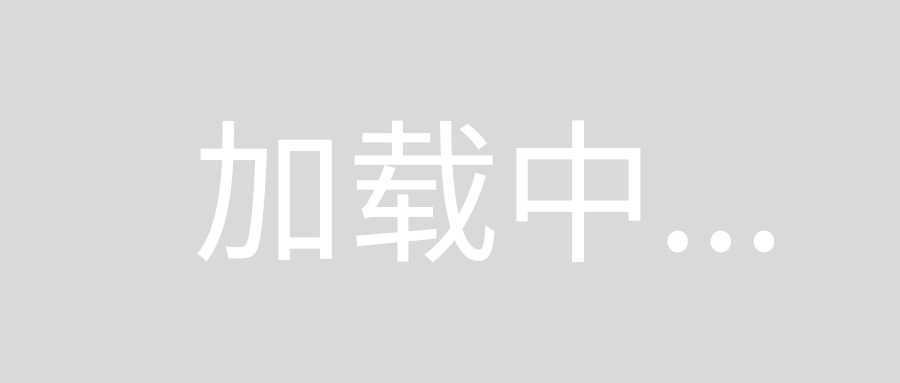
my placement of X,y,W,H
button 1
5,219,230,45
button 2
5,273,230,45
button 3
244,224,230,45
button 4
244,273,230,45
回答1:
I happened to be working on a similar game app, so got some code which will definitely help. Check it out below. Note that I've put it in that you'd call a 'correctAnswerMethod' and a 'wrongAnswerMethod' for the correct and incorrect button clicks.
// Define the four buttons in their respective frames, create the first button as the correct one, we'll shuffle it later.
UIButton *button1 = [UIButton buttonWithType:UIButtonTypeRoundedRect];
[button1 setTitle:@"Correct Answer" forState:UIControlStateNormal];
[button1 addTarget:self action:@selector(correctAnswerMethod:) forControlEvents:UIControlEventTouchUpInside];
[self.view addSubview:button1];
UIButton *button2 = [UIButton buttonWithType:UIButtonTypeRoundedRect];
[button2 setTitle:@"Wrong Answer 1" forState:UIControlStateNormal];
[button2 addTarget:self action:@selector(wrongAnswerMethod:) forControlEvents:UIControlEventTouchUpInside];
[self.view addSubview:button2];
UIButton *button3 = [UIButton buttonWithType:UIButtonTypeRoundedRect];
[button3 setTitle:@"Wrong Answer 2" forState:UIControlStateNormal];
[button3 addTarget:self action:@selector(wrongAnswerMethod:) forControlEvents:UIControlEventTouchUpInside];
[self.view addSubview:button3];
UIButton *button4 = [UIButton buttonWithType:UIButtonTypeRoundedRect];
[button4 setTitle:@"Wrong Answer 3" forState:UIControlStateNormal];
[button4 addTarget:self action:@selector(wrongAnswerMethod:) forControlEvents:UIControlEventTouchUpInside];
[self.view addSubview:button4];
//Create an array with the rectangles used for the frames
NSMutableArray *indexArray = [NSMutableArray arrayWithObjects:
[NSValue valueWithCGRect:CGRectMake(5, 219, 230, 45)],
[NSValue valueWithCGRect:CGRectMake(5, 273, 230, 45)],
[NSValue valueWithCGRect:CGRectMake(244, 219, 230, 45)],
[NSValue valueWithCGRect:CGRectMake(244, 273, 230, 45)], nil];
//Randomize the array
NSUInteger count = [indexArray count];
for (NSUInteger i = 0; i < count; ++i) {
int nElements = count - i;
int n = (arc4random() % nElements) + i;
[indexArray exchangeObjectAtIndex:i withObjectAtIndex:n];
}
//Assign the frames
button1.frame = [((NSValue *)[indexArray objectAtIndex:0]) CGRectValue];
button2.frame = [((NSValue *)[indexArray objectAtIndex:1]) CGRectValue];
button3.frame = [((NSValue *)[indexArray objectAtIndex:2]) CGRectValue];
button4.frame = [((NSValue *)[indexArray objectAtIndex:3]) CGRectValue];
回答2:
I have implemented code for same at my side and it works fine.
-(void)randomAllocation
{
NSMutableArray* allstring = [[NSMutableArray alloc]initWithObjects:@"Correct",@"Wrong",@"Wrong1",@"Wrong2", nil];
NSMutableArray* outcomeOFrandom = [[NSMutableArray alloc]init];
for (int i=0; i<[allstring count]; i++) {
int temp = 0;
while (temp==0) {
int randomInt = arc4random() % 4;
BOOL result = [outcomeOFrandom containsObject:[allstring objectAtIndex:randomInt]];
if (result==FALSE ) {
UIButton* btn = [[UIButton alloc]init];
[btn setTitle:[allstring objectAtIndex:randomInt] forState:UIControlStateNormal];
[btn setBackgroundColor:[UIColor blackColor]];
NSLog(@"tital=%@",[allstring objectAtIndex:randomInt]);
if (i==0) {
[btn setFrame:CGRectMake(5,219,230,45)];
}
else if(i==1)
[btn setFrame:CGRectMake(5,273,230,45)];
}
else if(i==2) {
[btn setFrame:CGRectMake(244,224,230,45)];
}
else if(i==3) {
[btn setFrame:CGRectMake(244,273,230,45)];
}
[outcomeOFrandom addObject:[allstring objectAtIndex:randomInt]];
[self.view addSubview:btn];
[btn release];
temp=1;
break;
}
}
}
}
回答3:
You can create the buttons in same position always and randomize the labels on the button. If you are using an array to provide labels like val1, val2, val3 etc, you can randomize the array.
回答4:
I would suggest do not change the position of buttons rather what you can do is change the currentTitle
property of the buttons randomly and once the user clicks the button ; you can get the currentTitle
of the button and check if it is the correct answer or not.
回答5:
Out of 4 options only one is correct so why you worry, just give tag to your UIButton. In touchUpInside event or any action you've specified on it. Just match the correct answer tag with user touched button tag.
You should set some logic to set answer with different button tag randomly.
回答6:
You should create button and add them as subView
. When you need to put button at random location, you can use:
[button1 setFrame:CGRectMake(5,219,230,45)];
[button2 setFrame:CGRectMake(5,273,230,45)];
[button3 setFrame:CGRectMake(244,224,230,45)];
[button4 setFrame:CGRectMake(244,273,230,45 )];
回答7:
i dont think you need to set your button's x and y here. just set the tag of your buttons, say tag 1 - 4, generate random number 1 - 4 (possible tag of your buttons), save your buttons to NSArray and do a loop like:
NSArray *buttonsArr = [[NSArray alloc]initWithObjects:button1,button2, button3, button4, nil];
int randomInt = (arc4random() % 4) + 1;
for (int i = 0; i < [buttonsArr count]; i++) {
if ([(UIButton*)[buttonsArr objectAtIndex:i] tag] == randomInt) {
[[buttonsArr objectAtIndex:i] setTitle:@"correct" forState:UIControlStateNormal];
}else{
[[buttonsArr objectAtIndex:i] setTitle:@"wrong" forState:UIControlStateNormal];
}
}
回答8:
create your own class with these 4 buttons. In this class you can store the positions of your buttons. then you can add a member function (something like the following) which will draw them:
-(void)drawButtonsWithIncorrectAtIndex:(int)index