This snipet Outputs two Letters when i press one Key (r).
string key = string.Empty;
key = Console.ReadKey().Key.ToString();
Console.WriteLine(key);
Console.ReadKey();
// Output: rR
Why does it output lower 'r' and Upercase 'R' when i only press once the lowercase 'r'?
The lower case r
is what you have written from keyboard. You do not remove it form console by reading it. And the capital letter R
is the character representation of the Key
from ConsoleKeyInfo
structure. If you want to read exactly what was written to console use Console.Read()
or Console.ReadLine()
.
Console.ReadKey
returns the ConsoleKeyInfo
object which expose a property Key
which is of ConsoleKey
type. Where the Key
is represented with enum
value With upper case letter. See below.
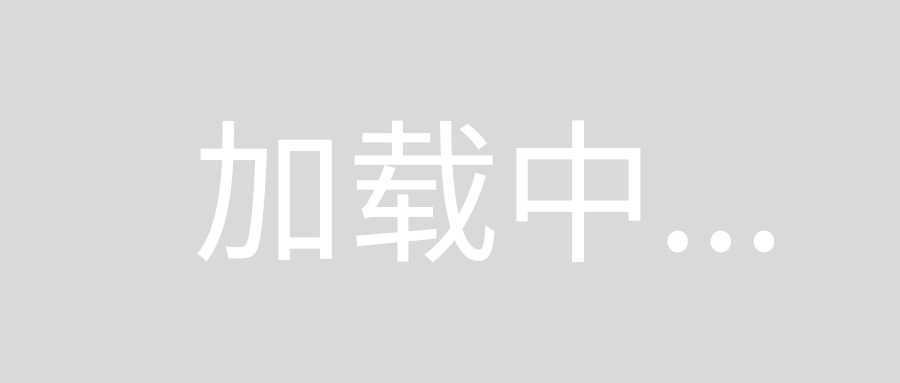
Now in your case, you are first reading which is r
using Console.ReadKey()
and they you are outputting the code using Console.WriteLine
which is printing Key value as R
.
The Key
-property returns a value of the enum ConsoleKey
.
The enum ConsoleKey
has a equivalent for every non-modifier-key (Alt, Shift & Ctrl; see enum ConsoleModifiers
).
Therefor, there are 2 possible signs that can be produced by pressing the key "R".
Another example to illustrate this is when you press 4
in your line of numbers above the keys. This is the ConsoleKey
D4. It can be (see key.KeyChar
) 4
or $
. Also note the property Modifiers
in the ConsoleKeyInfo
-class that shows the pressed modifying key.
See the example below to illustrate it:
var key = Console.ReadKey();//press 4
Console.WriteLine("ConsoleKey: " + key.Key); // D4
Console.WriteLine("Char: " + key.KeyChar); // 4
Console.WriteLine("ConsoleModifier: " + key.Modifiers); // 0
key = Console.ReadKey(); //press shift + 4
Console.WriteLine("ConsoleKey: " + key.Key); // D4
Console.WriteLine("Char: " + key.KeyChar); // $
Console.WriteLine("ConsoleModifier: " + key.Modifiers); // Shift
I've taken some information from MSDN
BTW: I only get "R" when pressing "R" or "r". I'm using C#6 with .Net 4.7. Perhaps you're using some newer or older version where it outputs all possible chars instead of the enum value itself on .ToString()
, like it does @ me.
Old question, but thought this may be useful to add.
As others have noted, Console.ReadKey()
does the job of both reading your inputted key and also writing it to screen (before your Console.WriteLine()
takes effect).
If you just wanted to read the input and not write it, you can use the optional bool intercept argument of Console.ReadKey()
to hide the user input, and then write it later:
ConsoleKeyInfo cki = Console.ReadKey(true); // hiding input
Console.Write($"You pressed: {cki.Key}");
// press letter "a" (lower case)
// Output: You pressed: A
// i.e. no duplicated letter
Console.ReadLine();
Link to MSDN section
If you needed lowercase output, just needs a slight modification:
ConsoleKeyInfo cki = Console.ReadKey(true); // hiding input
Console.Write($"You pressed: {cki.Key.ToString().ToLower()}");
// press letter "a" (lower case)
// Output: You pressed: a
Console.ReadLine();