After migrating to newest version of Material Components (1.1.0-alpha09
), I've noticed that TextInputLayout
has some padding horizontal padding. My goal is to achieve the old style of text fields, where there is no background or outline box. So I extended one of the styles, i.e. Widget.MaterialComponents.TextInputLayout.FilledBox
and set background color to transparent.
<style name="Widget.MyApp.TextInputLayout" parent="Widget.MaterialComponents.TextInputLayout.FilledBox.Dense">
<item name="boxBackgroundColor">@color/transparent</item>
</style>
Now the problem is that this input field looks weird in my app with such horizontal padding (which was necessary for filled or outline box).
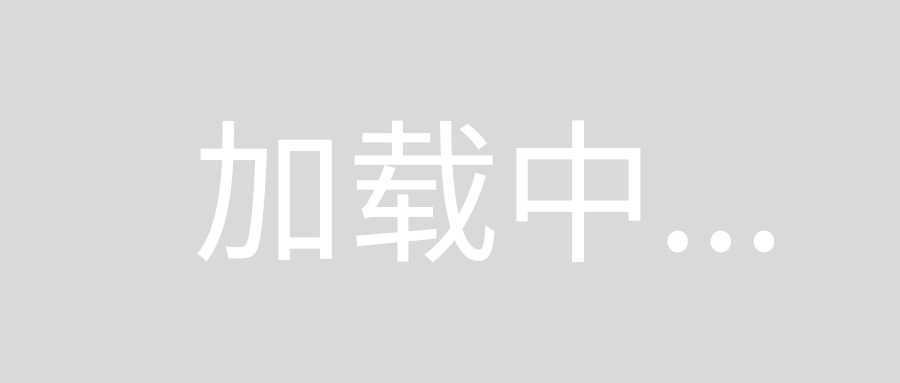
So my question is, how can I remove this padding?
You can use something like:
<com.google.android.material.textfield.TextInputLayout
....
android:hint="Hint text"
style="@style/My.TextInputLayout.FilledBox.Dense" >
Then you can define (it requires the version 1.1.0) a custom style using the materialThemeOverlay
attribute:
<style name="My.TextInputLayout.FilledBox.Dense" parent="Widget.MaterialComponents.TextInputLayout.FilledBox.Dense">
<item name="materialThemeOverlay">@style/MyThemeOverlayFilledDense</item>
</style>
<style name="MyThemeOverlayFilledDense">
<item name="editTextStyle">@style/MyTextInputEditText_filledBox_dense
</item>
</style>
<style name="MyTextInputEditText_filledBox_dense" parent="@style/Widget.MaterialComponents.TextInputEditText.FilledBox.Dense">
<item name="android:paddingStart" ns2:ignore="NewApi">4dp</item>
<item name="android:paddingEnd" ns2:ignore="NewApi">4dp</item>
<item name="android:paddingLeft">4dp</item>
<item name="android:paddingRight">4dp</item>
</style>
Here the results (with a default style and the custom style):
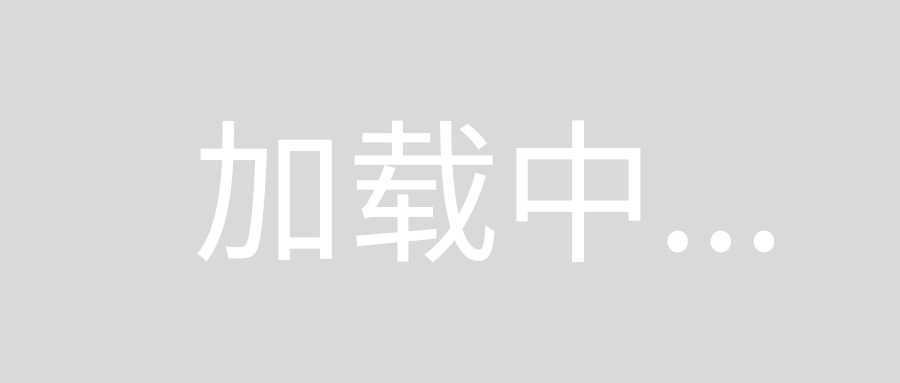
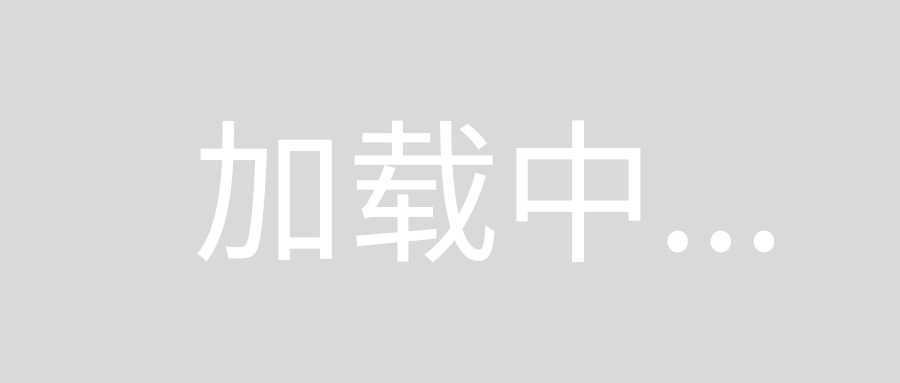