可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I want to do an Application test that parses some json, stores to core data, and reads out some objects.
How can my code know if it's being run as part of a test or normal run? Just some way to know "are we in test target"? Because the app when it fires up now kicks off a bunch of requests to populate my coredata with info from the server. I don't want it to do this during my tests. I want to fire up the App, read HARDCODED json from a file and store this using the same methods as otherwise into coredata, and verify the results.
If someone could explain how to pass specific key-value pairs on a per target basis that can be read from within the app, I would be even more delighted.
回答1:
Never mind... figured out that it is in "Schemes" that you set this.
For example if you want TARGET=TEST to be available during Test and TARGET=RUN to show during run, just set that in your Scheme > Environment Variables > Name/Value.
Then from your app you can do:
[[[NSProcessInfo processInfo] environment] objectForKey:@"TARGET"]
Using build settings with preprocessor macros DID NOT work for me b/c my test target (for application/integration testing) is dependent on my main (not test) target, so the main target is built first and that's what runs, and you end up with main target preprocessor macros even though you are after the ones defined in the target you ran. If I missed something here someone feel free to explain please.
回答2:
If by "test target" you mean your unit tests (i.e. Product > Test
or ⌘U
), you can add a preprocessor macro to the target and check for that macro in your code. This allows something like the following:
#ifdef TEST
// Load the hard-coded data.
#else
// Load data from the server.
#endif
To do this, click on your project file in the project navigator, select your test target, click the Build Settings
tab, search for "macros", double click the Preprocessor Macros
option, and add one!
回答3:
There two situations to deal with:
- Run some code if a certain target such as
Tests
is selected, and
- Conditionally
#import
some files for a certain target such as Tests
.
Target Code for Test Target:
Create a macro in your ProjectName-Prefix.pch
file as following:
#define IsTestTarget [[[[NSProcessInfo processInfo] environment][@"XCInjectBundle"] pathExtension] isEqualToString:@"xctest"]
and then call it anywhere in the app:
if (IsTestTarget) {
//Do something specific for test target;
} else {
//Otherwise do something else
}
Conditional #import
:
To #import
certain files when Tests
target is selected, you do need to add a Preprocessor Macro
to your Test
target and use it as:
#ifdef APPTESTS
#import "TestSpecificFile.h"
#else
#import "SomeOtherFile.h"
#endif
Here is how you can add a Preprocessor Macro
:
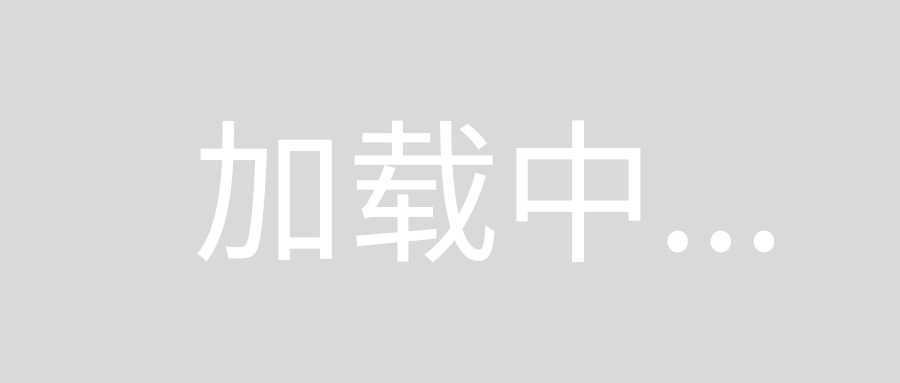
回答4:
You can use the below function.
+(BOOL) isRunningTests
{
NSDictionary* environment = [[NSProcessInfo processInfo] environment];
NSString* injectBundle = environment[@"XCInjectBundle"];
return [[injectBundle pathExtension] isEqualToString:@"xctest"];
}
回答5:
Usually in Unit Test programmers are using mocking classes and functionalities.
You can create a class with target membership only for the test target.
@interface MockClass : NSObject
@end
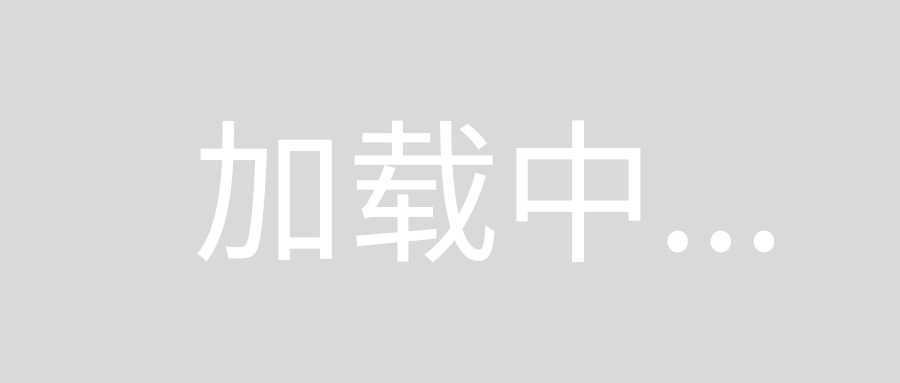
Then in the application code you can check if class exist using NSClassFromString
function (which will return Nil
for target not included in the class's target membership, in this case - non test target.
if (NSClassFromString(@"MockClass")) {
//Test Target
} else {
//App Target
}
And you can of curse function it
BOOL isUnitTest(){
return NSClassFromString(@"MockClass") != Nil;
}