可以将文章内容翻译成中文,广告屏蔽插件可能会导致该功能失效(如失效,请关闭广告屏蔽插件后再试):
问题:
I have a GPS coordinate (latitude, longitude) and I quickly want to place a single pin on a MKMapView showing that position. Everything works just fine, but as I only need a single pin with no callout is there a quicker way to do this or is what I have below what needs to be done?
- (MKAnnotationView *)mapView:(MKMapView *)mapView viewForAnnotation:(id < MKAnnotation >)annotation {
MKPinAnnotationView *pinView = [[MKPinAnnotationView alloc] initWithAnnotation:annotation reuseIdentifier:@"DETAILPIN_ID"];
[pinView setAnimatesDrop:YES];
[pinView setCanShowCallout:NO];
return pinView;
}
NB: I don't need to check for reusable annotation views as I am only using the pin to show a position in a detail view (which is destroyed and recreated the next time a detail view is requested).
回答1:
Instead of using the -mapView:viewForAnnotation:
method, just put the code for an MKPointAnnotation
into your -viewDidLoad
method. It won't animate the drop, but it is very easy.
// Place a single pin
MKPointAnnotation *annotation = [[MKPointAnnotation alloc] init];
[annotation setCoordinate:centerCoordinate];
[annotation setTitle:@"Title"]; //You can set the subtitle too
[self.mapView addAnnotation:annotation];
Swift version:
let annotation = MKPointAnnotation()
let centerCoordinate = CLLocationCoordinate2D(latitude: 41, longitude:29)
annotation.coordinate = centerCoordinate
annotation.title = "Title"
mapView.addAnnotation(annotation)
回答2:
You can set the point and also the region like this:
CLLocationCoordinate2D coord = CLLocationCoordinate2DMake(lat, lon);
MKCoordinateSpan span = MKCoordinateSpanMake(0.1, 0.1);
MKCoordinateRegion region = {coord, span};
MKPointAnnotation *annotation = [[MKPointAnnotation alloc] init];
[annotation setCoordinate:coord];
[self.staticMapView setRegion:region];
[self.staticMapView addAnnotation:annotation];
回答3:
The easiest and simplest method to drop a pin and displaying Current Location in Map View is to simply add this code in your viewDidLoad method. (Assuming that user's current location is already fetched by you).
NSString *display_coordinates=[NSString stringWithFormat:@"Latitude is %f and Longitude is %f",coordinate.longitude,coordinate.latitude];
MKPointAnnotation *annotation = [[MKPointAnnotation alloc] init];
[annotation setCoordinate:coordinate];
[annotation setTitle:@"Click Labs"];
[annotation setSubtitle:display_coordinates];
[mapView addAnnotation:annotation];
回答4:
Since you want to animate the drop, you will need to implement viewForAnnotation
as you've done because that property is NO
by default.
If you don't need to animate the drop, you could eliminate the viewForAnnotation
method implementation completely and to disable the callout, set the annotation's title
to nil or blank when adding the annotation instead.
If you do need the pin drop animation and you're also showing the user's location (blue dot), you'll also need to check for MKUserLocation
in viewForAnnotation
and return nil
.
Otherwise, you could remove the whole viewForAnnotation
method and the default red pin will appear without animation, the callout will show if title
is not blank, and the user location will show as a blue dot.
回答5:
Swift version of add pin in MKMapView:
func addPin() {
let annotation = MKPointAnnotation()
let centerCoordinate = CLLocationCoordinate2D(latitude: 20.836864, longitude:-156.874269)
annotation.coordinate = centerCoordinate
annotation.title = "Lanai, Hawaii"
mapView.addAnnotation(annotation)
}
Swift version of focusing a region or pin
func focusMapView() {
let mapCenter = CLLocationCoordinate2DMake(20.836864, -156.874269)
let span = MKCoordinateSpanMake(0.1, 0.1)
let region = MKCoordinateRegionMake(mapCenter, span)
mapView.region = region
}
To learn more details visit "Load MKMapView using Swift".
回答6:
Simply you can add it like:
mapView.mapType = MKMapType.standard
let location = CLLocationCoordinate2D(latitude: 23.0225,longitude: 72.5714)
let span = MKCoordinateSpanMake(0.05, 0.05)
let region = MKCoordinateRegion(center: location, span: span)
mapView.setRegion(region, animated: true)
let annotation = MKPointAnnotation()
annotation.coordinate = location
annotation.title = "Javed Multani"
annotation.subtitle = "Surat, India"
mapView.addAnnotation(annotation)
It's look like:
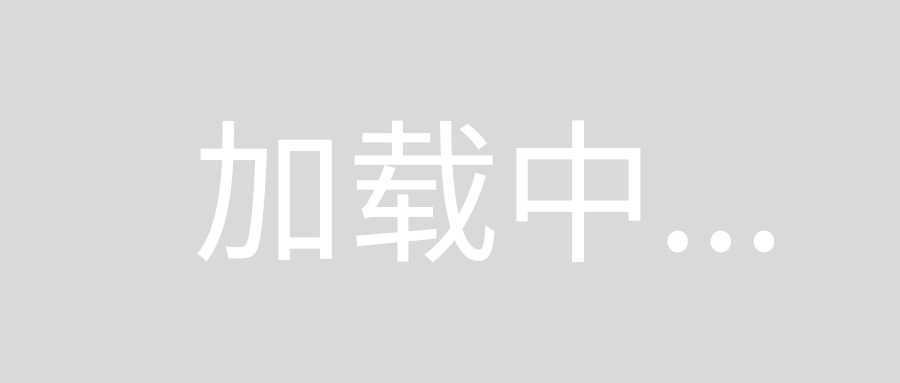