I am trying to work with a path in Python 2.7
This is what I am trying to do in my main class:
program = MyProgram()
program.doSomething('C:\Eclipse\workspace\MyProgram\files\12345678_Testing1_ABCD005_Static_2214_File12.txt')
Inside the function doSomething(filePath)
the string already looks like this:
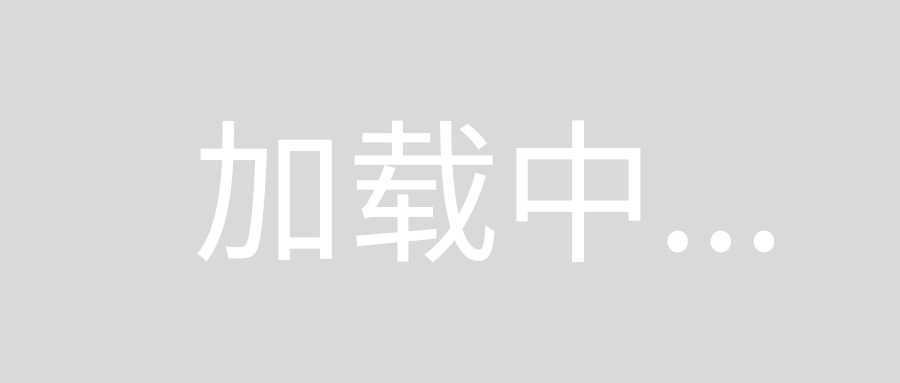
So there is a kind of special character plus some characters are removed copletely. What could cause this problem?
\
is escape char in Python. According to docs, you have created string with \f ASCII Formfeed (FF)
character.
String literals can be enclosed in matching single quotes ('
) or
double quotes ("
). They can also be enclosed in matching groups of
three single or double quotes (these are generally referred to as
triple-quoted strings). The backslash (\
) character is used to escape
characters that otherwise have a special meaning, such as newline,
backslash itself, or the quote character.
Either use double slashes (\\
- to escape escape character) or use raw string literals (r"some\path"
).
String literals may optionally be prefixed with a letter 'r' or 'R';
such strings are called raw strings and use different rules for
interpreting backslash escape sequences.
This is probably overkill in your situation,
you could use os.path.join
to build the path. With this you have two advantages:
- it builds paths using the current system's separator (Unix '/' VS Windows '\')
- you don't have to care about escaping the separator in case of windows
As said, this is probably overkill for your code:
import os.path
program = MyProgram()
my_path = os.path.join('C:',
'Eclipse',
'workspace',
'MyProgram',
'files',
'12345678_Testing1_ABCD005_Static_2214_File12.txt')
program.doSomething(my_path)
The backslash seems to be creating a special character. Placing an extra backslash (to act as an escape charater) behind the backslashes should fix it.
program = MyProgram()
program.doSomething('C:\\Eclipse\\workspace\\MyProgram\\files\\12345678_Testing1_ABCD005_Static_2214_File12.txt')
We can convert Windows path names containing escape characters by converting it into raw string.
If you want to hard code your string then you can use
mypath = r'C:\this_is_my_path'
and python will ignore '\t' in the above string.
However if you have a string variable containing escape characters then you can use the below mentioned method.
def string2RawString(string):
rawString = ''
for i in string.split('\\'):
rawString = rawString+("%r"%i).strip("'")+"\\"
return rawString